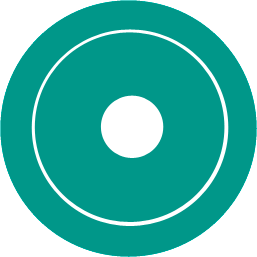
Advanced Placement (AP), 23.10.2020 01:01 fabiolabaritto
Implement a class Car with the following properties. A car has a certain fuel efficiency (measured in miles/gallon or liters/km—pick one) and a certain amount of fuel in the gas tank. The efficiency is specified in the constructor, and the initial fuel level is 0. Supply a method drive that simulates driving the car for a certain distance, reducing the amount of gasoline in the fuel tank. Also supply methods getGasInTank, returning the current amount of gasoline in the fuel tank, and addGas, to add gasoline to the fuel tank. Sample usage: Car myHybrid = new Car(50); // 50 miles per gallon myHybrid. addGas(20); // Tank 20 gallons myHybrid. drive(100); // Drive 100 miles double gasLeft = myHybrid. getGasInTank(); // Get gas remaining in tank You may assume that the drive method is never called with a distance that consumes more than the available gas. Supply a CarTester class that tests all methods.
This is my code, please help me fix it:
Car. java
public class Car {
// Instant Fields
private double gas;
private double efficiency;
// Constructor
public Car(double fe) {
fuelEff = fe;
gas = 0;
}
// Methods
public void addGas(double amount) {
gas = gas + amount;
}
public void drive(double distance) {
drive = drive + distance;
gas = gas - (distance / milesPerGallon);
}
public double getGasInTank() {
return gas;
}
}
Tester
class Main {
public static void main(String[] args) {
//E3.12 car
carTester();
public static void carTester() {
Car myHybrid = new Car(50);
myHybrid. addGas(20);
myHybrid. drive(100);
double gasLeft = myHybrid. getGasInTank();
System. out. println("Gas Left: " + gasLeft);
}
}

Answers: 2
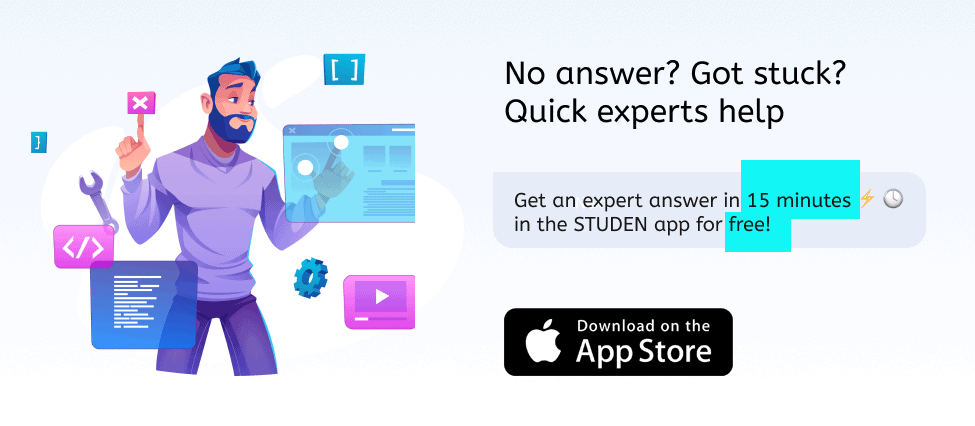
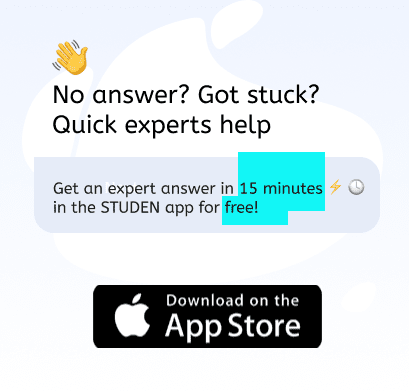
Another question on Advanced Placement (AP)
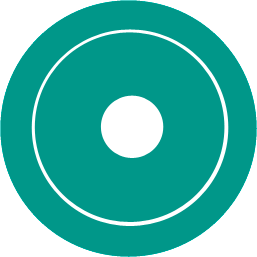
Advanced Placement (AP), 23.06.2019 19:30
How does public opinion differ on foreign and domestic policy issues? how does this affect the policy- making process?
Answers: 1
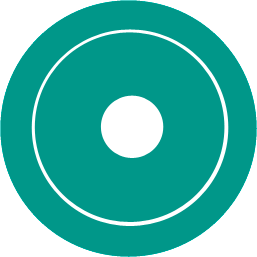
Advanced Placement (AP), 24.06.2019 04:00
Astudent is revising the sentence below: i know brendan was upset, but i feel he overreacted. you would have thought he'd just lost his best friend, the way he would bemoan his fate. based on the context of this sentence, which is the best synonym for bemoan? a. complain: express dissatisfaction or annoyance b. grieve: cause distress to someone c. nag: annoy with persistent fault-finding d. provoke: stimulate or incite
Answers: 1
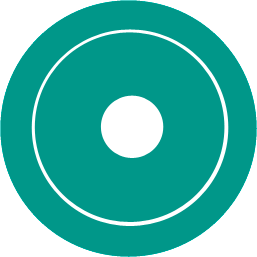
Advanced Placement (AP), 24.06.2019 11:20
Arectangle is removed from a right triangle to create the shaded region shown below. find the area of the shaded region.be sure to include the correct unit in your answer.14 mmm² mxlal? 4m3m
Answers: 1
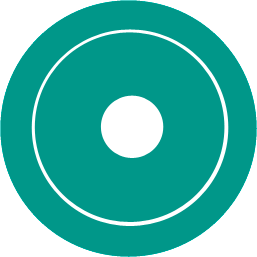
Advanced Placement (AP), 25.06.2019 04:30
Which of the following tools can be used to determine humidity? select all that apply. barometer: hygrometers: anemometer: hair: thermometer
Answers: 1
You know the right answer?
Implement a class Car with the following properties. A car has a certain fuel efficiency (measured i...
Questions

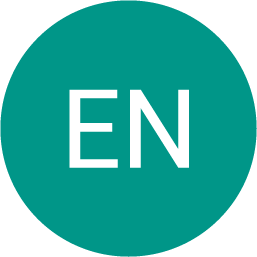


Chemistry, 31.03.2021 21:10

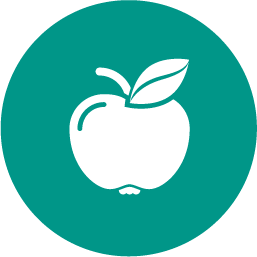
Physics, 31.03.2021 21:10




Mathematics, 31.03.2021 21:10
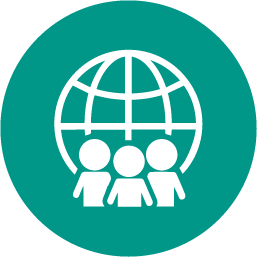

Mathematics, 31.03.2021 21:10

Mathematics, 31.03.2021 21:10


Mathematics, 31.03.2021 21:10


Computers and Technology, 31.03.2021 21:10

Business, 31.03.2021 21:10

Mathematics, 31.03.2021 21:10