
Computers and Technology, 10.09.2019 18:30 Jessicadiaz8602
You will implement random maze generation. start by downloading the attached source file. study the starting code and make the following changes:
implement makemazerecursive(char[][]level, int startx, int starty, int endx, int endy) using the recursive division method. a general description is on wikipedia:
"mazes can be created with recursive division, an algorithm which works as follows: begin with the maze's space with no walls. call this a chamber. divide the chamber with a randomly positioned wall (or multiple walls) where each wall contains a randomly positioned passage opening within it. then recursively repeat the process on the subchambers until all chambers are minimum sized. this method results in mazes with long straight walls crossing their space, making it easier to see which areas to avoid.
for example, in a rectangular maze, build at random points two walls that are perpendicular to each other. these two walls divide the large chamber into four smaller chambers separated by four walls. choose three of the four walls at random, and open a one cell-wide hole at a random point in each of the three. continue in this manner recursively, until every chamber has a width of one cell in either of the two directions."
you will implement random maze generation. start b
this function is recursive. note: do not call it on a sub-area when that area is less than 3x3 - this will cause walls to double up.
since this algorithm does not use any data structures or back tracking, it can create mazes that are not solveable.
hints:
(the screen shots below use numbers for walls in order to indicate which call to makemazerecursive created them - you should use only a # in your final submission.)
it is not necessary to follow these hints, you may implement this algorithm as you see fit based on the description above.
consider creating a function called randbetween(int l, int u) that creates a random number in an interval - wouldn't this function be useful for deciding where walls should go?
as a first step, implement the basic functionality to draw the two walls that divide the area into four rooms; don't proceed (i. e. add recursion) until it works.
then implement the recursive call for just one of the sub-areas; don't proceed until it works.
then extend the code to the other sub-areas.
after you are done, rename the finalized code to "lastname_pp3.java" (e. g. "acuna_pp3.java"). remember to change the class name as well.
/**
* write a description of class base_pp3 here.
*
* @author (your name), acuna
* @version (a version number or a date)
*/
import java. util. random;
public class base_pp3
{
//standard console size in characters.
private static final int level_height = 25;
private static final int level_width = 80;
private static final char icon_wall = '#';
private static final char icon_blank = ' ';
/**
* returns a 2d array containing a statically created maze with dimentions 80x24.
*
* @return 2d array containing a maze
*/
private static char[][] makemazestatic()
{
//the following maze was generated with the recursive division method and then modified by hand.

Answers: 1
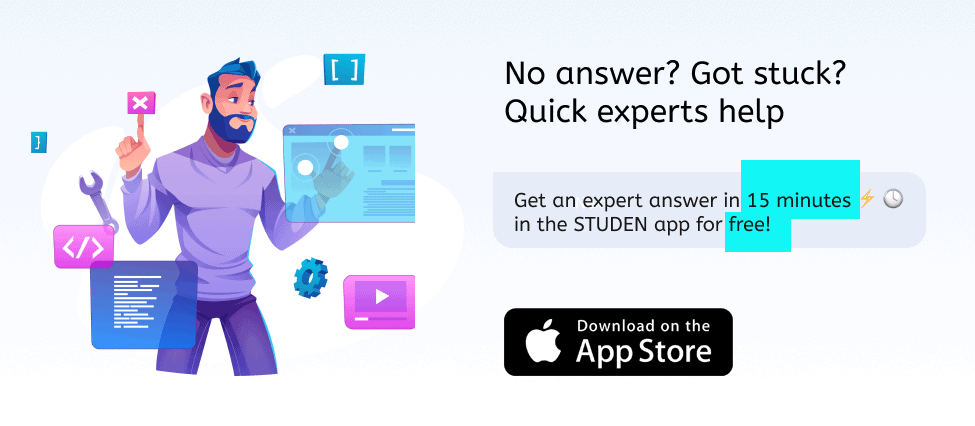
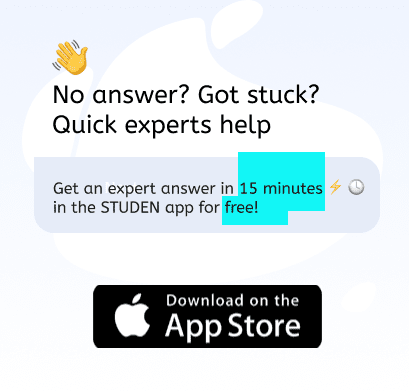
Another question on Computers and Technology

Computers and Technology, 22.06.2019 04:30
Kyle, a receptionist for a building supply company, recently won an award for saving his company money on their phone system. after being presented with kyle's research, the company changed the way in which it made long-distance phone calls and cut their expenses in this area by 75 percent. the new system the kyle's company most likely adopted was wired communications switching stations voip hdtv
Answers: 3

Computers and Technology, 24.06.2019 01:00
Me if you do then you get 10 points and brainliest
Answers: 1

Computers and Technology, 24.06.2019 03:40
4. does the kernel phenotype distribution support the idea that the cob is the result of a dihybrid cross? what information supports your answer? if a dihybrid cross (i.e. f1 to f2 of standard mendelian crosses) is not indicated what conditions might contribute to this finding.
Answers: 2

Computers and Technology, 24.06.2019 09:30
Atype of researcher who uses computers to make sense of complex digital data
Answers: 1
You know the right answer?
You will implement random maze generation. start by downloading the attached source file. study the...
Questions
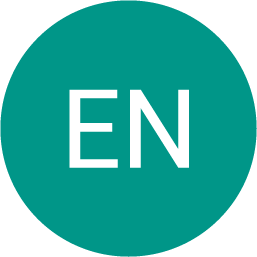
English, 24.08.2021 17:50
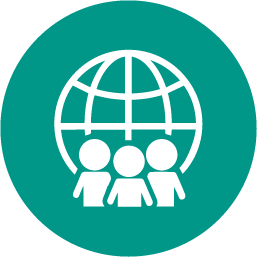

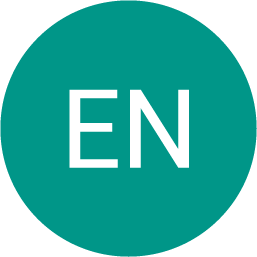



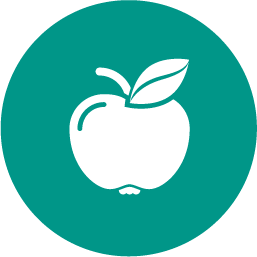
Physics, 24.08.2021 17:50

Mathematics, 24.08.2021 17:50

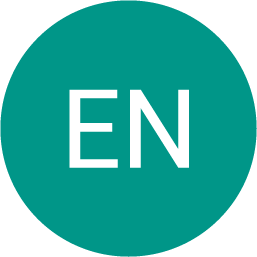

Mathematics, 24.08.2021 17:50
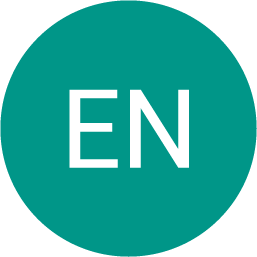
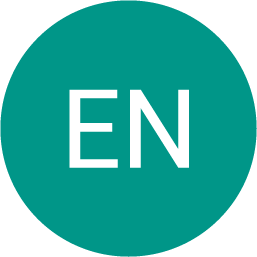
English, 24.08.2021 17:50




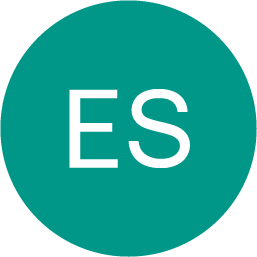
Spanish, 24.08.2021 17:50

Mathematics, 24.08.2021 17:50