
Computers and Technology, 22.11.2019 23:31 asiababbie33
The c language allows you to define new names for existing types using typedefs. here is some example code that uses typedefs:
typedef int money;
int x;
money y;
typedef money dollars;
dollars z;
x = 10;
y = x; // ok because x and y are of type int
z = y; // ok because y and z are of type int
the first typedef defines money to be a synonym for int. any declaration that follows this typedef can use money instead of int. the second typedef defines dollars to be a synonym for money, which makes it a synonym for int. any declaration that follows this typedef can use dollars instead of int.
typedefs can also be used with struct types:
struct pair {
int x;
int y;
};
typedef struct pair point;
point p;
a typedef can occur anywhere that a variable declaration (local or global) can occur. the usual c scoping rules apply to the names in typedefs. note that typedef int money; is considered to be a declaration of the name money and that both money x; and typedef money dollars; are considered to be uses of the name money.
question 2
now consider the name-analysis phase of the compiler. note that, in addition to the usual errors for multiply-defined names and for uses of undefined names, the name analyzer must enforce the following rules:
the declaration typedef t xxx; is an error if t is not a built-in type (e. g., int, bool) or a struct type (in which case t will be of the form: struct ttt) or a new type defined by a previous typedef in the current or an enclosing scope.
the declaration typedef t xxx; is an error if xxx has already been declared in the current scope (as a variable, function, parameter, or new type).
a variable, function, or parameter can only be declared to be of type t if t is either a built-in type or a new type defined by a previous typedef in the current or an enclosing scope. (a variable can still be declared to be of type struct ttt as before.)
answer each of the following questions:
what information should be stored with each name in the symbol table?
what should be done to process a typedef: typedef t xxx; ?
what should be done to process a declaration of a variable, function, or parameter named xxx and declared to be of type t?
what should be done to process the use of a name xxx in a statement?
illustrate your answer by showing the entries that would be in the symbol table after processing the following declarations:
struct monthdayyear {
int month;
int day;
int year;
};
typedef struct monthdayyear date;
date today;
typedef int dollars;
dollars salary;
typedef dollars moredollars;
moredollars md;
int d;

Answers: 3
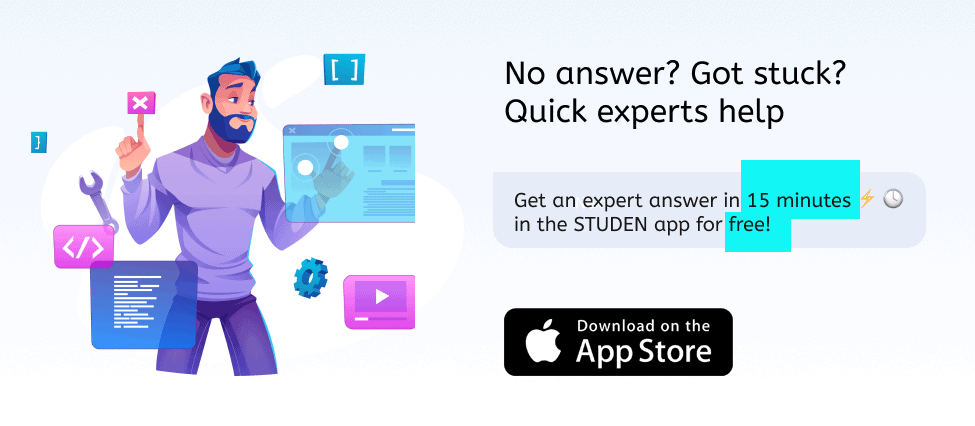
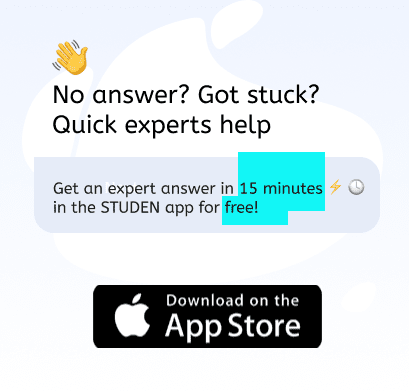
Another question on Computers and Technology

Computers and Technology, 22.06.2019 00:00
Sam is a data analyst at an advertising firm. he often uses a spreadsheet that contains media ratings details. he would like to filter the spreadsheet data based on different filter criteria. which operators can he use to specify the combination of filter criteria? sam can use the ( blank ) operators to specify a combination of filter criteria.
Answers: 3

Computers and Technology, 22.06.2019 06:30
Plz 40 points what are raster vectors? a bitmap image a vector file a type of printing press a small projector
Answers: 1

Computers and Technology, 22.06.2019 22:40
In this lab, you complete a python program that calculates an employee's annual bonus. input is an employee's first name, last name, salary, and numeric performance rating. if the rating is 1, 2, or 3, the bonus rate used is .25, .15, or .1 respectively. if the rating is 4 or higher, the rate is 0. the employee bonus is calculated by multiplying the bonus rate by the annual salary.
Answers: 1

Computers and Technology, 23.06.2019 10:50
Your friend kayla is starting her own business and asks you whether she should set it up as a p2p network or as a client-server network. list three questions you might ask to kayla decide which network to use and how her answers to those questions would affect your recommendation.
Answers: 2
You know the right answer?
The c language allows you to define new names for existing types using typedefs. here is some exampl...
Questions
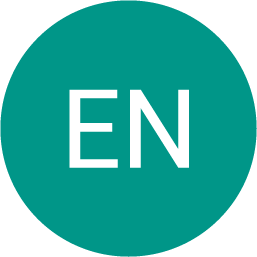
English, 04.03.2021 05:10
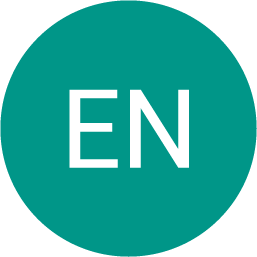
English, 04.03.2021 05:10
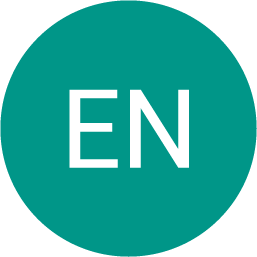
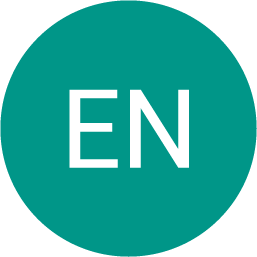

Biology, 04.03.2021 05:10


Mathematics, 04.03.2021 05:10

Mathematics, 04.03.2021 05:10
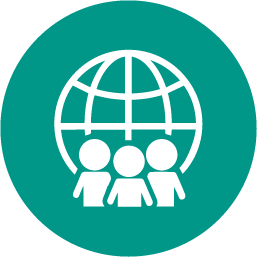

Mathematics, 04.03.2021 05:10


Mathematics, 04.03.2021 05:10




Mathematics, 04.03.2021 05:10

Mathematics, 04.03.2021 05:10
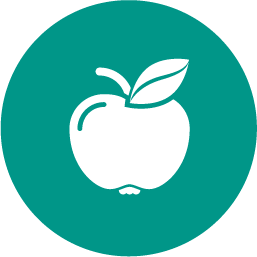
Physics, 04.03.2021 05:10

Mathematics, 04.03.2021 05:10

Medicine, 04.03.2021 05:10