Objectives:
junit testing
collections: set
logic exception handling
gettin...

Computers and Technology, 27.11.2019 23:31 live4dramaoy0yf9
Objectives:
junit testing
collections: set
logic exception handling
getting started
to begin this lab, create a new java project named abc123-lab7, and create the following packages and classes:
bank. account. java
bank. bank. java
this lab does not deal with synchronized or multithreading. correct solutions to this lab may not be thread-safe.
banks & accounts
bank. java will define functionality for a bank. banks must have a name and a set of accounts. use a java. util. set implementation of your choosing for this data structure, and the account. java implementation for the accounts stored.
bank. java must have a single constructor to properly initialize banks. in addition, there should be an overloaded method called addaccount which can add account objects to the bank given either a name for the account, or both a name for the account and a starting balance. this method will create an account object and add it to the set stored in the bank object, nothing will be returned.
creating a new object would be as follows:
bank bank = new bank("gringotts");
account. java will define functionality for an account. accounts must have a name associated with them, an account number, and a balance. as described above, an account object can be defined using either all three of these (name, number, and balance) or only with the name and number. (this indicates the constructor must be overloaded - one for each of these cases.) if a starting balance is not provided, it will be assumed to be 0. there should be getters and setters for all of these three variables.
account. java must have two additional methods: deposit and withdraw.
deposit will be an object method that takes in an amount of money and adds the amount to the balance of an account. deposits should inherently only the positive values. this method will return the new balance of the account.
withdraw will be an object method that takes in an amount of money and reduces the balance by that amount. withdrawls should inherently only be positive values. withdrawls larger than the current balance should not be permitted. this method will return the new balance of the account.
creating a new object would be as follows:
account account1 = new account("amy smith", 2202);
or
account account2 = new account("bob smith", 1584, 100.0);
junit testing
create a junit 4 test case, called accounttest. java. this class will test only the functionality in the account class, defined above. implement the following methods:
setup() - method runs before each test is run.
testinit() - test the constructors.
testvaliddeposit() - test depositing a valid amount (i. e. positive amount) into the account.
testinvaliddeposit() - test depositing an invalid amount (i. e. negative amount) into the account.
testvalidwithdrawl() - test withdrawing a valid amount (i. e. positive amount) from the account.
testinvalidwithdrawl() - test withdrawing an invalid amount (i. e. negative amount) from the account.
testoverdraft() - test withdrawing more than the balance from the account.
no test methods should be created for the getters or setters in the account class. each method should have the appropriate junit annotation. overdrafts should not be permitted, instead a message must be presented to the user. for each test listed, consider the preconditions and postconditions of the method in testing. consider any input (parameters), logical requirements (described in the class descriptions above), and outputs (returned values). what could go wrong when the method is called? equally important, what could be wrong after the method is called?
in the process of implementing these tests, you should run them and verify they are passing. if a test does not pass, first assess the test (is it set up then verify your implementation meets the requirements (see account. java above). a completed submission for this lab will correctly assess all test cases, and running accounttest. java will result in a pass (green bar in the junit view).
note that your submitted eclipse project must include the dependecy for junit 4. to ensure this, export your code from eclipse to an archive file, as in the lab instructions!
unsure about a test or implementation?
imagine you are writing code for your bank. how do you want your money to be handled? if you were to try to deposit or withdraw an invalid amount of money, would you want your account balances to be affected? would you want to be warned of the error?

Answers: 3
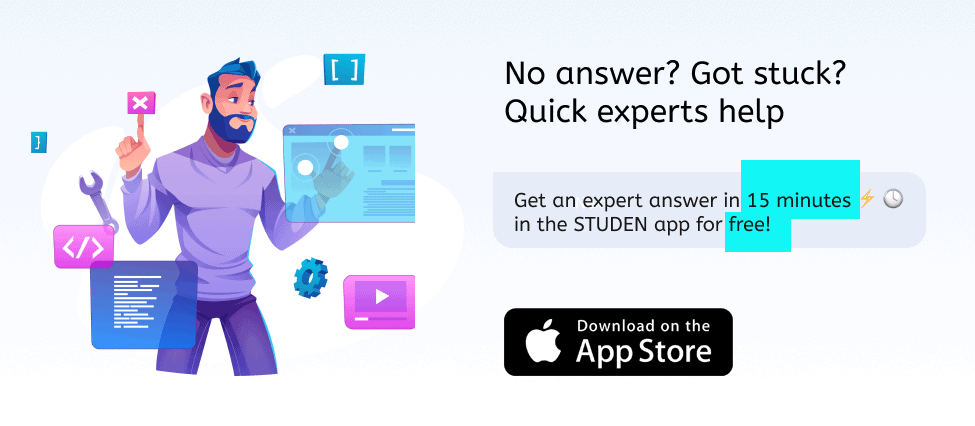
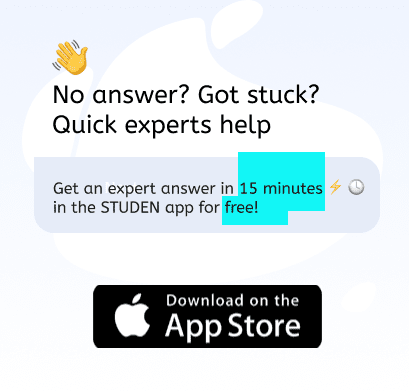
Another question on Computers and Technology

Computers and Technology, 22.06.2019 01:50
Click on this link toopens a new window. bring up a flowchart in a new browser window. based on this flowchart, would a d-link 3347 gateway with an xbox 360 multiplayer problem be in scope or out of scope
Answers: 2

Computers and Technology, 22.06.2019 10:40
Nims is applicable to all stakeholders with incident related responsibilities. true or false
Answers: 1

Computers and Technology, 22.06.2019 23:30
What is the digital revolution and how did it change society? what are the benefits of digital media?
Answers: 1

Computers and Technology, 23.06.2019 06:30
When early motion pictures played in movie theaters, they were often accompanied by live organ or piano music. which of the following are the most likely reasons that this happened? (select all that apply). the music was provided to distract audience members from the loud sounds made when filmstrips were changed. the music accompanied the movies because the movies were silent and audiences were used to hearing music during plays in theaters. the music usually was played before, and sometimes after the movie, as an alternative form of entertainment. the music viewers to interpret the dramatic action in the films.
Answers: 2
You know the right answer?
Questions


Mathematics, 07.07.2020 02:01
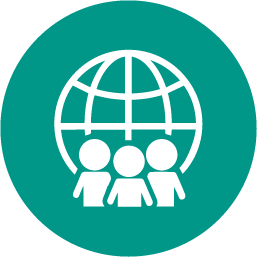
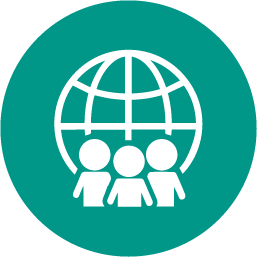


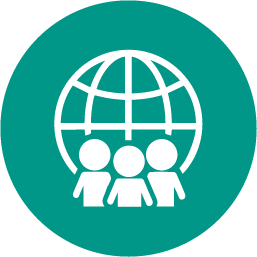
Social Studies, 07.07.2020 02:01
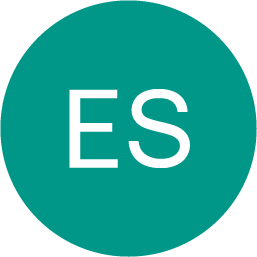




Mathematics, 07.07.2020 02:01


Mathematics, 07.07.2020 02:01

Mathematics, 07.07.2020 02:01
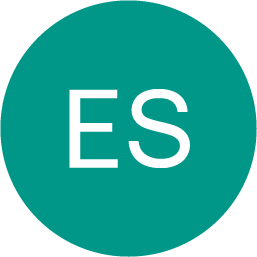

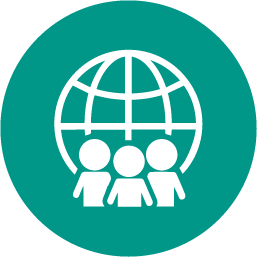
History, 07.07.2020 02:01

Chemistry, 07.07.2020 02:01