In this problem we are going to use ArrayLists and classes to design a road trip.
You ha...

Computers and Technology, 11.02.2020 22:21 vlactawhalm29
In this problem we are going to use ArrayLists and classes to design a road trip.
You have three classes: GeoLocation. java from earlier, which represents a geo location. A RoadTrip. java class which represents a road trip (or an ordered list of places), and a RoadTripTester. java class which brings them all together.
In GeoLocation. java:
Add a private instance variable called name which is a String. This represents the name of the location.
Modify the Geolocation class constructor so that it is now of the format
public GeoLocation(String name, double theLatitude, double theLongitude)
Add a getter method for name called getName().
Update the toString so that it returns a String of the format
San Francisco (37.7833, -122.4167)
Now, you’ll also need to create a RoadTrip class. The RoadTrip stores an ordered list of locations, so you’ll need to have an ArrayList. You’ll also need to support these methods.
// Create a GeoLocation and add it to the road trip
public void addStop(String name, double latitude, double longitude)
// Get the total number of stops in the trip
public int getNumberOfStops()
// Get the total miles of the trip
public double getTripLength()
// Return a formatted toString of the trip
public String toString()
We’ve given you a tester program to help get you started.
The output from that program would be:
1. San Francisco (37.7833, -122.4167)
2. Los Angeles (34.052235, -118.243683)
3. Las Vegas (36.114647, -115.172813)
Stops: 3
Total Miles: 572.9708850442705
import java. util.*;
public class RoadTrip
{
}
public class GeoLocation
{
// Earth radius in miles
public static final double RADIUS = 3963.1676;
private double latitude;
private double longitude;
private String name;
/**
* Constructs a geo location object with given latitude and longitude
*/
public GeoLocation(String name, double theLatitude, double theLongitude)
{
latitude = theLatitude;
longitude = theLongitude;
}
/**
* Returns the latitude of this geo location
*/
public String getName()
{
return name;
}
public double getLatitude()
{
return latitude;
}
/**
* returns the longitude of this geo location
*/
public double getLongitude()
{
return longitude;
}
public void addStop(String name, double latitude, double longitude)
{
}
// returns a string representation of this geo location
public String toString()
{
return name + "(" + latitude + ", " + longitude + ")";
}
// returns the distance in miles between this geo location and the given
// other geo location
public double distanceFrom(GeoLocation other)
{
double lat1 = Math. toRadians(latitude);
double long1 = Math. toRadians(longitude);
double lat2 = Math. toRadians(other. latitude);
double long2 = Math. toRadians(other. longitude);
// apply the spherical law of cosines with a triangle composed of the
// two locations and the north pole
double theCos = Math. sin(lat1) * Math. sin(lat2) +
Math. cos(lat1) * Math. cos(lat2) * Math. cos(long1 - long2);
double arcLength = Math. acos(theCos);
return arcLength * RADIUS;
}
}

Answers: 2
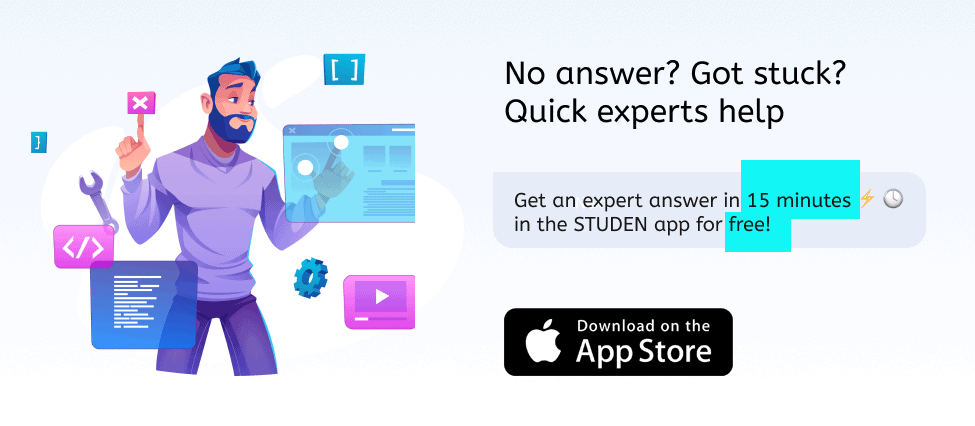
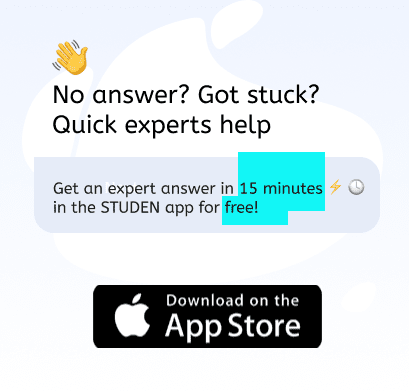
Another question on Computers and Technology

Computers and Technology, 22.06.2019 01:00
Program description: a c# app is to be created to produce morse code. the morse code assigns a series of dots and dashes to each letter of the alphabet, each digit, and a few special characters (such as period, comma, colon, and semicolon). in sound-oriented systems, the dot represents a short sound and the dash represents a long sound. separation between words is indicated by a space, or, quite simply, the absence of a dot or dash. in a sound-oriented system, a space is indicated by a short period of time during which no sound is transmitted. the international version of the morse code is stored in the data file morse.txt.
Answers: 3

Computers and Technology, 23.06.2019 19:30
2. fluorine and chlorine molecules are blamed fora trapping the sun's energyob forming acid rainoc producing smogod destroying ozone molecules
Answers: 2

Computers and Technology, 23.06.2019 22:30
How many points do i need before i can send a chat
Answers: 1

Computers and Technology, 24.06.2019 07:00
You are most likely to automatically encode information about
Answers: 1
You know the right answer?
Questions

Mathematics, 11.02.2020 18:27


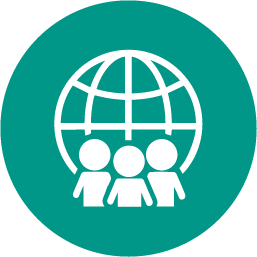
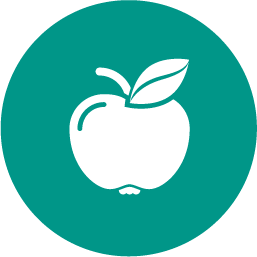


Mathematics, 11.02.2020 18:27

Engineering, 11.02.2020 18:27



Computers and Technology, 11.02.2020 18:27

Computers and Technology, 11.02.2020 18:27


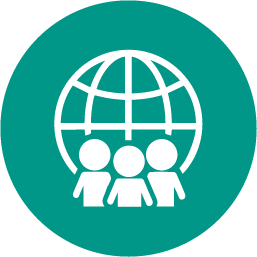




Computers and Technology, 11.02.2020 18:27

Engineering, 11.02.2020 18:27