
Computers and Technology, 12.02.2020 05:57 genyjoannerubiera
Compare Fibonacci (recursion vs. bottom up)
In this project we will compare the computational time taken by a recursive algorithm to determine the Fibonacci number of an integer n and the time taken by a bottom-up approach (using a loop) to calculate the Fibonacci number of the same integer n.
A Fibonacci number F(n) is determined by the following recurrence function:
F(0) =0; F(1)=1;
F(n)= F(n-1) + F(n-2), for n ≥ 2
Thus the recursive algorithm can be written in C++ as
int FiboR ( int n) // array of size n
{ if (n==0 || n==1)
return (n);
else return (FiboR (n-1) + FiboR(n-2));
}
And the non-recursive algorithm can be written in C++ as
int FiboNR ( int n) // array of size n
{ int F[max];
F[0]=0; F[1]=1;
for (int i =2; i <=n; i++)
{ F[i] = F[i-1] + F[i-2];
}
return (F[n]);
}
While FiboR takes exponential time FiboNR takes n steps
Write an algorithm that computes the time (in seconds using ctime. h header library file that takes to determine Fibonacci (n) using FiboR and the time taken by FiboNR on the same input n.
Try to run both routines using different values of n (n={1,5,10,15,20,25,30,35,40,45,50, 55,60…)
You final output should look like:
Fibonacci time analysis (recursive vs. non-recursive)
Integer FiboR (seconds) FiboNR(seconds) Fibo-value
1 XX. XX XX. XX 1
5 XX. XX XX. XX 5
.. .. ..
60 XX. XX XX. XX
…

Answers: 1
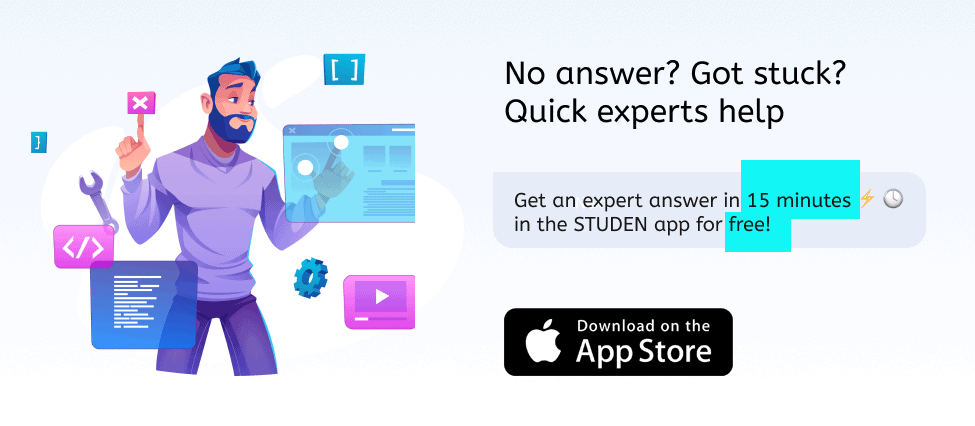
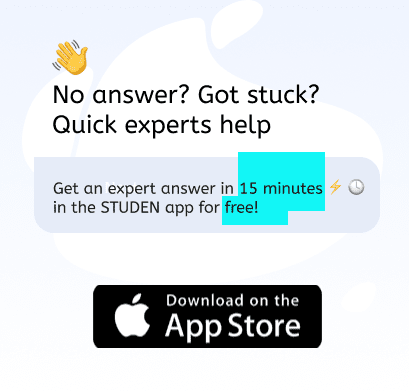
Another question on Computers and Technology

Computers and Technology, 23.06.2019 00:30
Quic which one of the following is the most accurate definition of technology? a electronic tools that improve functionality b electronic tools that provide entertainment or practical value c any type of tool that serves a practical function d any type of tool that enhances communication
Answers: 1

Computers and Technology, 23.06.2019 09:00
Which best describes the role or restriction enzymes in the analysis of edna a. to break dna into fragments that vary in size so they can be sorted and analyzed b. to amplify small amounts of dna and generate large amounts of dna for analysis c. to purify samples of dna obtained from the environment so they can be analyzed d. to sort different sizes of dna fragments into a banding pattern that can be analyzed
Answers: 1

Computers and Technology, 23.06.2019 20:30
Column a of irma’s spreadsheet contains titles for each row, but her document is too big and will be printed three pages across. she wants to be sure that every page will be understood. what can irma do to with this problem?
Answers: 3

Computers and Technology, 24.06.2019 04:30
Fall protection, confined space entry procedures, controlled noise levels, and protection from chemical hazards are some of the things that contribute to a safe what
Answers: 1
You know the right answer?
Compare Fibonacci (recursion vs. bottom up)
In this project we will compare the computa...
In this project we will compare the computa...
Questions
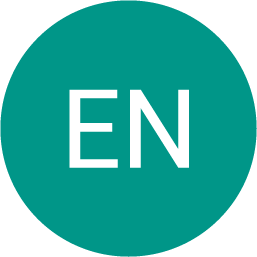
English, 20.07.2019 03:00
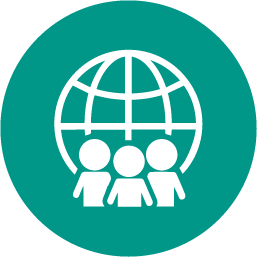
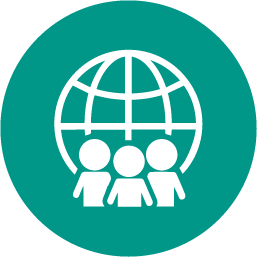
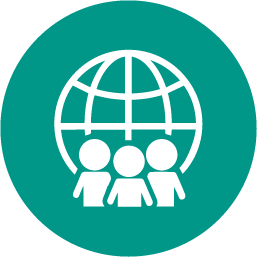

Mathematics, 20.07.2019 03:00

Mathematics, 20.07.2019 03:00


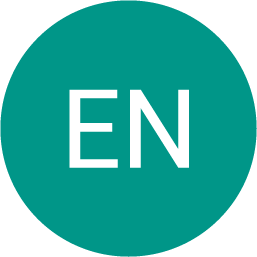


Mathematics, 20.07.2019 03:00

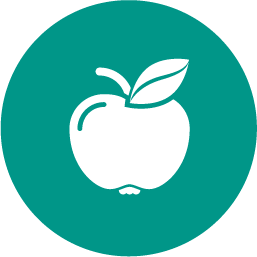

Health, 20.07.2019 03:00
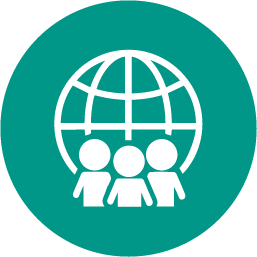
Social Studies, 20.07.2019 03:00

Chemistry, 20.07.2019 03:00
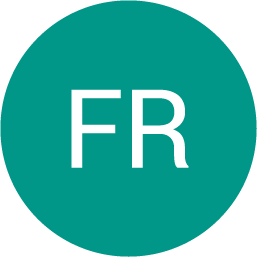
French, 20.07.2019 03:00
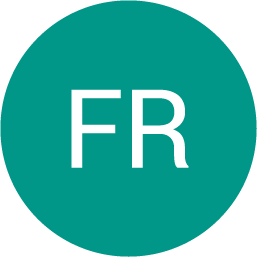
French, 20.07.2019 03:00

Geography, 20.07.2019 03:00