
Computers and Technology, 24.02.2020 23:33 TonyHaas07
Complete the implementation of the ArrayStack class. Specifically, complete the implementations of the isEmpty, size, and toString methods. See Base_A05Q1.java for a starting place and a description of these methods./** * Write a description of the program here. * * @author Lewis et al., (your name) * @version (program version) */import java. util. Arrays;public class Base_A05Q1{ /** * Program entry point for stack testing. * @param args Argument list. */ public static void main(String[] args) { ArrayStack stack = new ArrayStack(); System. out. println("STACK TESTING"); stack. push(3); stack. push(7); stack. push(4); System. out. println(stack. peek()); stack. pop(); stack. push(9); stack. push(8); System. out. println(stack. peek()); System. out. println(stack. pop()); System. out. println(stack. peek()); System. out. println("The size of the stack is: " + stack. size()); System. out. println("The stack contains:\n" + stack. toString()); } /** * An array implementation of a stack in which the bottom of the * stack is fixed at index 0. * * @author Java Foundations * @version 4.0 */ public static class ArrayStack implements StackADT { private final static int DEFAULT_CAPACITY = 100; private int top; private T[] stack; /** * Creates an empty stack using the default capacity. */ public ArrayStack() { this(DEFAULT_CAPACITY); } /** * Creates an empty stack using the specified capacity. * @param initialCapacity the initial size of the array */ @SuppressWarnings("unchecked") //see p505. public ArrayStack(int initialCapacity) { top = 0; stack = (T[])(new Object[initialCapacity]); } /** * Adds the specified element to the top of this stack, expanding * the capacity of the array if necessary. * @param element generic element to be pushed onto stack */ public void push(T element) { if (size() == stack. length) expandCapacity(); stack[top] = element; top++; } /** * Creates a new array to store the contents of this stack with * twice the capacity of the old one. */ private void expandCapacity() { stack = Arrays. copyOf(stack, stack. length * 2); } /** * Removes the element at the top of this stack and returns a * reference to it. * @return element removed from top of stack * @throws EmptyCollectionException if stack is empty */ public T pop() throws EmptyCollectionException { if (isEmpty()) throw new EmptyCollectionException("stack"); top--; T result = stack[top]; stack[top] = null; return result; } /** * Returns a reference to the element at the top of this stack. * The element is not removed from the stack. * @return element on top of stack * @throws EmptyCollectionException if stack is empty */ public T peek() throws EmptyCollectionException { if (isEmpty()) throw new EmptyCollectionException("stack"); return stack[top-1]; } /** * Returns true if this stack is empty and false otherwise. * @return true if this stack is empty */ public boolean isEmpty() { //TODO: Implement me. return false; } /** * Returns the number of elements in this stack. * @return the number of elements in the stack */ public int size() { //TODO: Implement me. return 0; } /** * Returns a string representation of this stack. The string has the * form of each element printed on its own line, with the top most * element displayed first, and the bottom most element displayed last. * If the list is empty, returns the word "empty". * @return a string representation of the stack */ public String toString() { //TODO: Implement me. return ""; } }}

Answers: 3
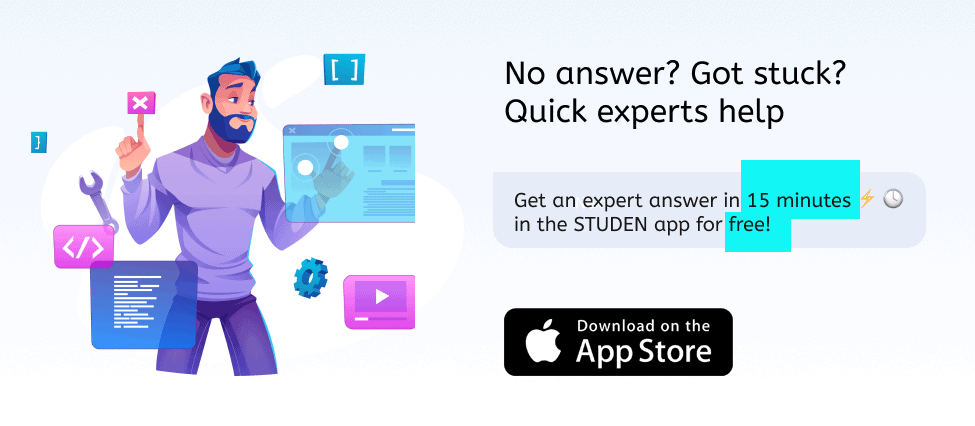
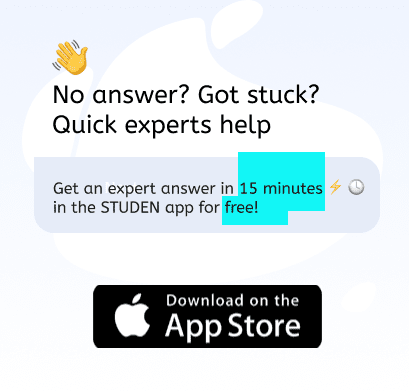
Another question on Computers and Technology

Computers and Technology, 22.06.2019 20:00
What side length would you specify if you were required to create a regular hexagonal plate that was composed of 33 cm(squared) of sheet metal? dimension the side length to 0.1 cm
Answers: 2

Computers and Technology, 23.06.2019 02:00
As with any small island country, cuba has fewer natural resources than countries such as brazil. this affects their economy in that cuba a) exports only manufactured products. b) exports more products than it imports.. c) must import more products than it exports. d) has imposed trade barriers against the united states.
Answers: 3


Computers and Technology, 24.06.2019 10:20
Multiple choice project create a program with two multiple choice questions. 1. users have two attempts only, show attempt number each time. hint: while loop with break control. (20%) 2. only one correct answer for each question, use switch case for each question. (20%) 3. show total score after the two questions are answered. hint: . (20%) 4. user have options to answer the two questions again if first attempt score is not 100%. hint: if statment. (20%) 5. use string method .toupper() to allow users to enter with lowercase or uppercase letters. (20%) 1. where is the capital of the state of florida? a. orlando b. tallahassee c. miami d. tampa b 2. where is walt disney world park located in florida? a. orlando b. tallahassee c. miami d. tampa a
Answers: 1
You know the right answer?
Complete the implementation of the ArrayStack class. Specifically, complete the implementations of t...
Questions

Mathematics, 21.07.2019 06:30


Mathematics, 21.07.2019 06:30

Business, 21.07.2019 06:30

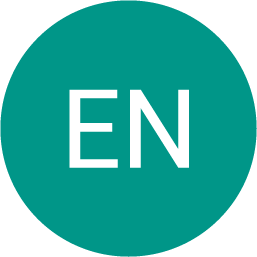


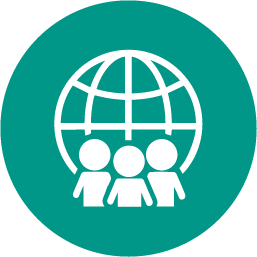
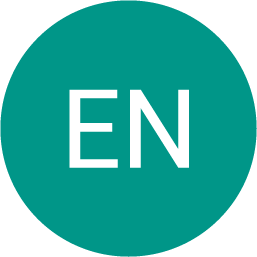
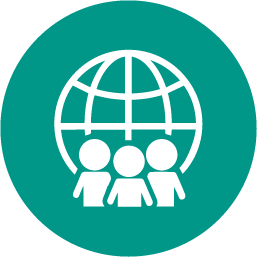
History, 21.07.2019 06:30
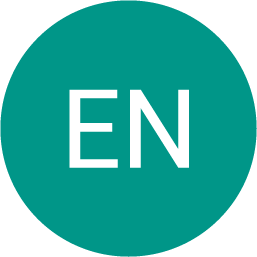
English, 21.07.2019 06:30
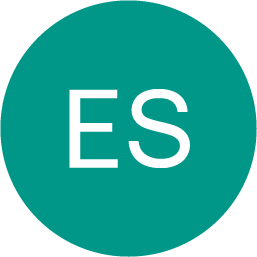
Spanish, 21.07.2019 06:30
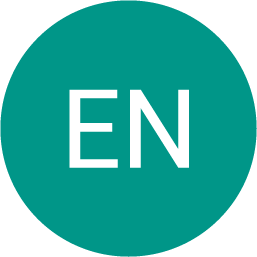


Mathematics, 21.07.2019 06:30
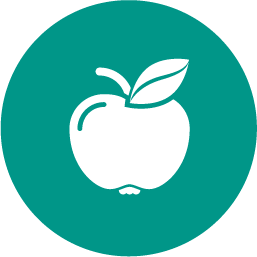

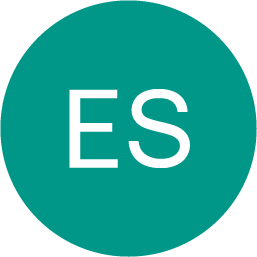