A stack machine executes programs consisting of commands that manipulate an internal stack.
T...

Computers and Technology, 02.03.2020 21:25 aliviafrancois2000
A stack machine executes programs consisting of commands that manipulate an internal stack.
The stack is initially empty.
The commands are:
push N; push N onto the stack (N is any Double)
pop; removes top element from a stack
add; replace top two elements of the stack by their sum
mul; replace top two elements of the stack by their product
sub; replace top two elements of the stack by their difference:
[a, b, ... ] => [a-b, ... ]
div; replace top two elements of the stack by their quotient:
[a, b, ... ] => [a/b, ... ]
For example, the following program computes 23:
(push 1, push 2, mul, push 2, mul, push 2, mul)
The following types of exceptions are thrown by the stack machine:
stack empty
stack too short (not enough elements to complete the operation)
divide by 0
syntax error
(A) Implement a generic stack class for the stack machine:
class Stack { ??? }
Notes:
·You will need to implement the following methods: push, pop, top, clear, and toString.
·pop and top may throw EmptyStack exceptions
·How will you store the elements in the stack? List? Set?
(B) Implement your design using your solution for part 2.
Notes:
·Executing arithmetic commands may throw ShortStack exceptions if there aren't at least two elements on the stack.
·div may throw a DivByZero exception. Restore the stack before throwing this exception.
·Consider making the execute method and stack in your StackMachine class static. Then, commands can make static references to the stack without needing to have an association to the stack machine.
·The execute method of StackMachine should have separate catch clauses for each type of exception. For now it just prints the exception but in the future we could potentially have different strategies for each type of exception caught.
·After the last command is executed the stack machine should print the stack.
·Before the first command is executed the stack machine should clear the stack.

Answers: 1
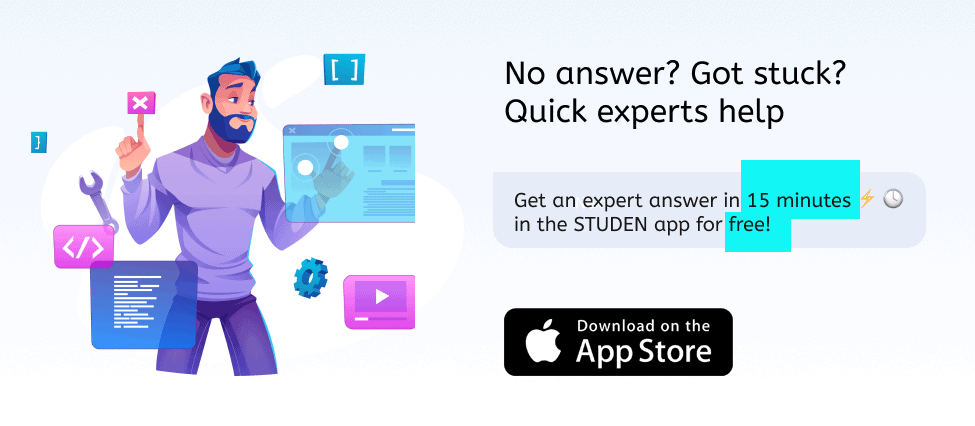
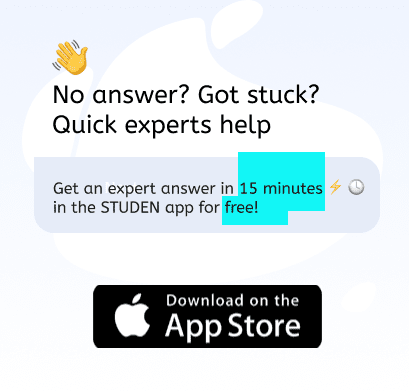
Another question on Computers and Technology

Computers and Technology, 22.06.2019 18:30
What is outfitting a workplace with video in a technology
Answers: 2

Computers and Technology, 22.06.2019 19:40
Solve the following javafx application: write a javafx application that analyzes a word. the user would type the word in a text field, and the application provides three buttons for the following: - one button, when clicked, displays the length of the word.- another button, when clicked, displays the number of vowels in the word.- another button, when clicked, displays the number of uppercase letters in the word(use the gridpane or hbox and vbox to organize the gui controls).
Answers: 1

Computers and Technology, 23.06.2019 16:00
Write a grading program for a class with the following grading policies: a. there are two quizzes, each graded on the basis of 10 points. b. there is one midterm exam and one final exam, each graded on the basis of 100 points. c. the final exam counts for 50% of the grade, the midterm counts for 25%, and the two quizzes together count for a total of 25%. (do not forget to normalize the quiz scores. they should be converted to a percentage before they are averaged in.) any grade of 90 or more is an a, any grade of 80 or more (but less than 90) is a b, any grade of 70 or more (but less than 80) is a c, any grade of 60 or more (but less than 70) is a d, and any grade below 60 is an f. the program will read in the student’s scores and output the student’s record, which consists of two quiz and two exam scores as well as the student’s average numeric score for the entire course and final letter grade. define and use a structure for the student reco
Answers: 2

Computers and Technology, 23.06.2019 21:30
To move a file or folder in microsoft windows, you can click and hold down the left mouse button while moving your mouse pointer to the location you want the file or folder to be, which is also known as.
Answers: 3
You know the right answer?
Questions

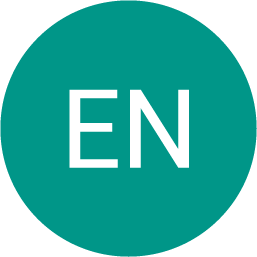

Arts, 13.10.2020 17:01

Mathematics, 13.10.2020 17:01
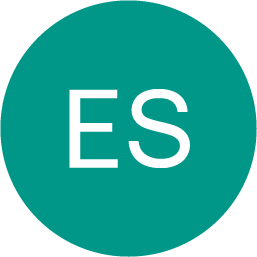
Spanish, 13.10.2020 17:01

Biology, 13.10.2020 17:01

Mathematics, 13.10.2020 17:01
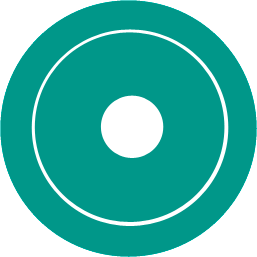
Advanced Placement (AP), 13.10.2020 17:01
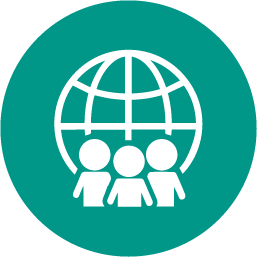

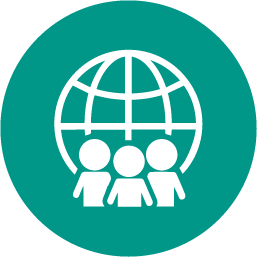
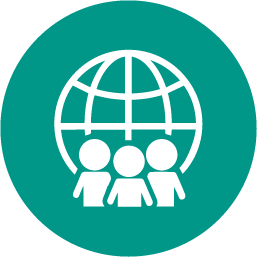


Mathematics, 13.10.2020 17:01

Mathematics, 13.10.2020 17:01

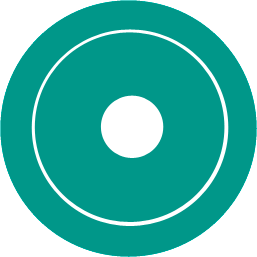
Advanced Placement (AP), 13.10.2020 17:01

Mathematics, 13.10.2020 17:01
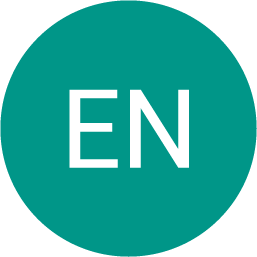