
Computers and Technology, 02.03.2020 21:29 mariaaalopezz
Create a LinkedList of Word objects using all the words found in the input file words. txt. A Word object contains 2 String fields; 1 to store a word in its normal form and the other to store a word in its canonical form. The canonical form stores a word with its letters in alphabetical order, e. g. bob would be bbo, cat would be act, program would be agmoprr, and so on. The class Word constructor has the responsibility of storing the normal form of the word in the normal form field and converting the normal form into the canonical form which is stored in the canonical form field (you should call a separate method for this conversion purpose).
Once all the words from the input file have been properly stored in a LinkedList of Word, you should use Collections to sort this list ascending alphabetically based on the canonical words by making the Word class Comparable.
Using an Iterator on the LinkedList of Word, create a 2nd list (new LinkedList) consisting of objects of a new class named AnagramFamily. AnagramFamily should contain at least 2 fields; 1 to hold a list of "Word" words that are all anagrams of each other (these should all be grouped together in the original canonical sorted list), and the 2nd field to store an integer value of how many items are in the current list. (Keep in mind, because the original list contains both the normal and canonical forms, as the AnagramFamily List will also have, a family of anagrams will all have the same canonical form with different normal forms stored in the normalForm field of the Word class). Each AnagramFamily List of Word should be sorted Descending by normal form using a Comparator of Word (if you insert Word(s) into a family one at a time, this presents an issue on how to get this list sorted as each Word insertion will require a new sort to be performed to guarantee the list is always sorted. For this reason it is best to form a list, sort it, and then create an AnagramFamily by passing the sorted list to it).
Sort the AnagramFamily LinkedList in descending order based on family size by use of a Comparator to be passed to the Collections sort method.
Next, output the top five largest families then, all families of length 8, and lastly, the very last family stored in the list to a file named "out9.txt." Be sure to format the output to be very clear and meaningful.
Finally, the first 4 people to complete the assignment should post their output results to the Canvas discussion forum for the remaining students to see the correct answer.
Be sure to instantiate new objects whenever transferring data from one object to another to avoid a repeat of the issues involved with Programming Assignment 6. Also, be sure to include various methods for manipulation and access of fields as well as helper methods to reduce code in main, such as the input/output of file data (like all other assignments, you will be graded on decomposition, i. e. main should not contain too many lines of code).
Keep in mind such items as proper documentation (including javadoc), meaningful variable names, proper indentation, reduction of redundancy whenever possible, and so on.
If written correctly (use of iterators and care taken when creating the anagram families), the running time should be less than 3 seconds.
Though the basic algorithms involved are straight forward enough, there is a great deal of complexity involved with various levels of access to specific data.
As a reminder, you will create at least 5 files: the driver, Word class, AnagramFamily class, and 2 comparators: 1 to compare Word objects for sorting descending based on the normal form of Word objects and 1 to compare AnagramFamily sizes for a descending sort.
will contain a minimum of 5 classes.

Answers: 1
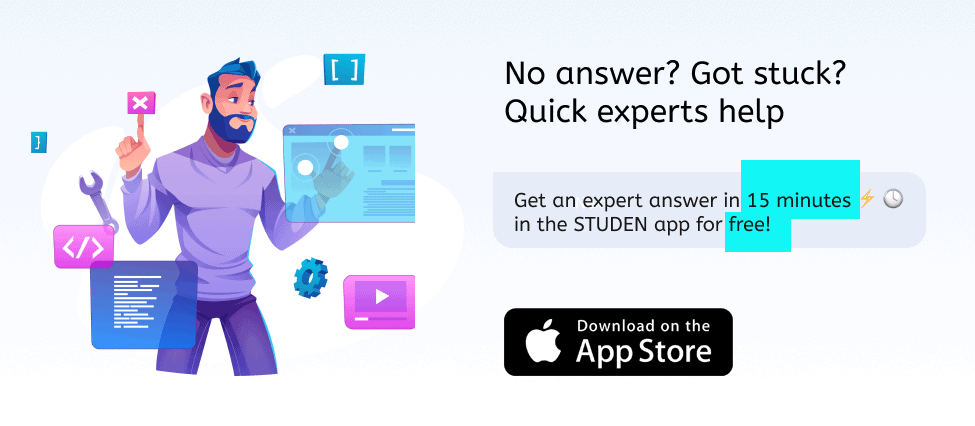
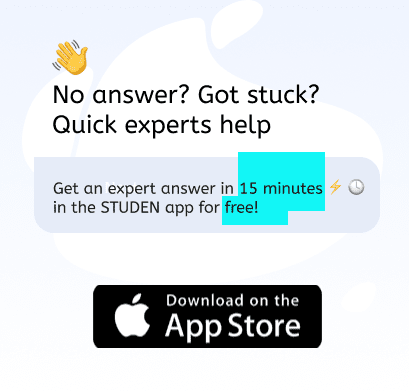
Another question on Computers and Technology

Computers and Technology, 23.06.2019 12:20
When guido van rossum created python, he wanted to make a language that was more than other programming languages. a. code-based b. human-readable c. complex d. functional
Answers: 1

Computers and Technology, 24.06.2019 11:00
Each row in a database is a set of unique information called a(n) ? a.) table. b.) record. c.) object. d.) field.
Answers: 2

Computers and Technology, 24.06.2019 12:50
When is it most apprpriate for a development team to change the definition of done
Answers: 1

Computers and Technology, 25.06.2019 06:00
Me on this app how do you take a picture of your work
Answers: 1
You know the right answer?
Create a LinkedList of Word objects using all the words found in the input file words. txt. A Word o...
Questions

Law, 24.05.2021 08:20

Computers and Technology, 24.05.2021 08:20
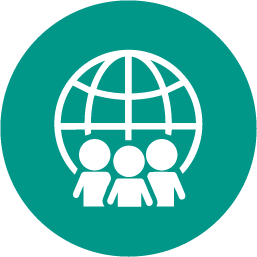
World Languages, 24.05.2021 08:20


Mathematics, 24.05.2021 08:20


Mathematics, 24.05.2021 08:20

Mathematics, 24.05.2021 08:20
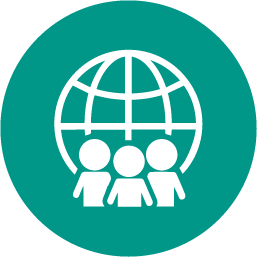
History, 24.05.2021 08:20


Mathematics, 24.05.2021 08:30
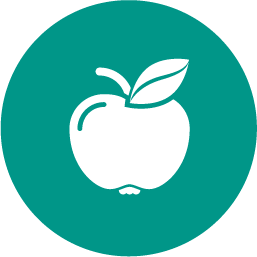
Physics, 24.05.2021 08:30


Biology, 24.05.2021 08:30
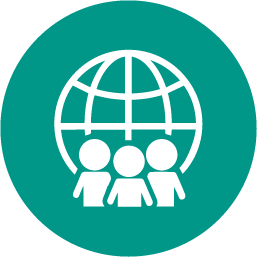

Mathematics, 24.05.2021 08:30


Mathematics, 24.05.2021 08:30
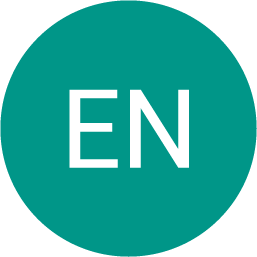
English, 24.05.2021 08:30

Mathematics, 24.05.2021 08:30