
Computers and Technology, 03.03.2020 04:52 JBBunny
In this assignment, you will model the game of Bulgarian Solitaire. The game starts with 45 cards. (They need not be playing cards. Unmarked index cards work just as well.) Randomly divide them into some number of piles of random size. For example, you might start with piles of size 20, 5, 1, 9, and 10. In each round, you take one card from each pile, forming a new pile with these cards. For example, the sample starting configuration would be transformed into piles of size 19, 4, 8, 10, and 5. The solitaire is over when the piles have size 1, 2, 3, 4, 5, 6, 7, 8, and 9, in some order. (It can be shown that you always end up with such a configuration.)
In your program, produce a random starting configuration and print it. Then keep applying the solitaire step and print the result. Stop when the solitaire final configuration is reached.
Use the following class as your main class:
import java. util. ArrayList;
import java. util. Random;
public class BulgarianSolitaire
{
private ArrayList piles;
/**
Sets up the game randomly with some number of piles of random
size. The pile sizes add up to 45.
*/
public void setupRandomly()
{
. . .
}
/**
This method can be used to set up a pile with a known (non-random)
configuration for testing.
@param pileSizes an array of numbers whose sum is 45
*/
public void setup(int[] pileSizes)
{
piles = new ArrayList();
for (int s : pileSizes)
piles. add(s);
}
public String getPiles()
{
return piles. toString();
}
/**
Play the game.
*/
public void play()
{
while (!isDone())
{
System. out. println(getPiles());
playRound();
}
System. out. println(getPiles());
}
/**
Play one round of the game.
*/
public void playRound()
{
. . .
}
/**
Checks whether the game is done.
@return true when the piles have size
1, 2, 3, 4, 5, 6, 7, 8, and 9, in some order.
*/
public boolean isDone()
{
. . .
}
}
Sample run:
13 4 6 6 10 6
6 12 3 5 5 9 5
7 5 11 2 4 4 8 4
8 6 4 10 1 3 3 7 3
9 7 5 3 9 2 2 6 2
9 8 6 4 2 8 1 1 5 1
10 8 7 5 3 1 7 4
8 9 7 6 4 2 6 3
8 7 8 6 5 3 1 5 2
9 7 6 7 5 4 2 4 1
9 8 6 5 6 4 3 1 3
9 8 7 5 4 5 3 2 2
9 8 7 6 4 3 4 2 1 1
β¦β¦β¦.
9 8 7 6 4 5 3 2 1
9 8 7 6 5 3 4 2 1
9 8 7 6 5 4 2 3 1
9 8 7 6 5 4 3 1 2
9 8 7 6 5 4 3 2 1
Number of iterations: 52

Answers: 3
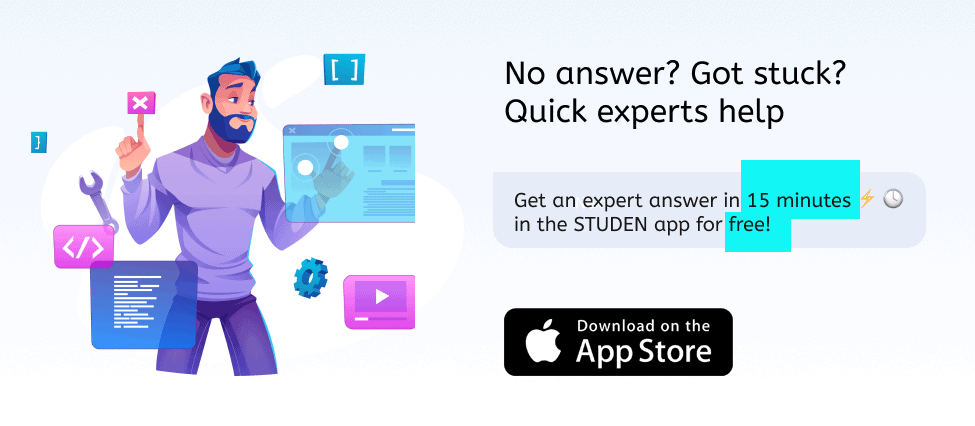
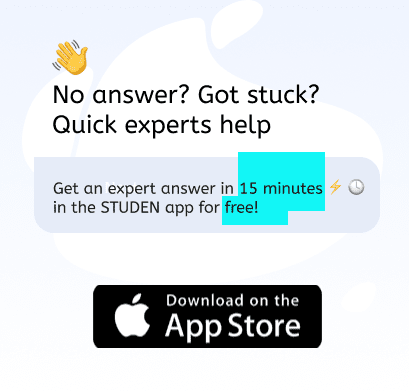
Another question on Computers and Technology

Computers and Technology, 22.06.2019 06:00
The width of a piece of rectangular land is 5m shorter rhan 1/3 of its length .find the width of the land if the length is 60m,150m.
Answers: 1

Computers and Technology, 22.06.2019 14:40
You are working with a professional edition organization. they wish to install the expense tracker which requires the use of 4 custom tabs, 3 custom objects, and one custom app. if the company is already using 4 applications, 36 custom objects, and 7 custom tabs, what will happen when they try to install expense tracker?
Answers: 1

Computers and Technology, 22.06.2019 21:00
Kirk found a local community college with a two-year program and he is comparing the cost with that of an out-of-state two-year school. what is the expected total cost for one year at the local community college if kirk lives at home? what is the expected total cost for one year at the out-of-state school if kirk lives on campus?
Answers: 2

Computers and Technology, 23.06.2019 19:30
2. fluorine and chlorine molecules are blamed fora trapping the sun's energyob forming acid rainoc producing smogod destroying ozone molecules
Answers: 2
You know the right answer?
In this assignment, you will model the game of Bulgarian Solitaire. The game starts with 45 cards. (...
Questions

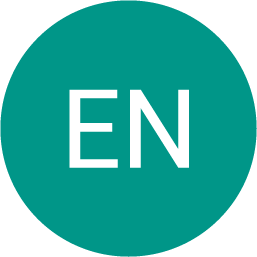
English, 20.11.2019 14:31
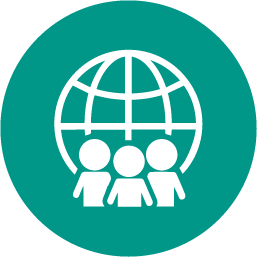

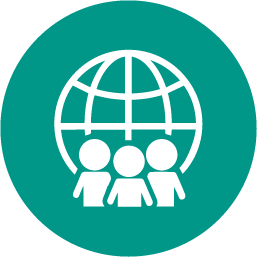

Biology, 20.11.2019 14:31



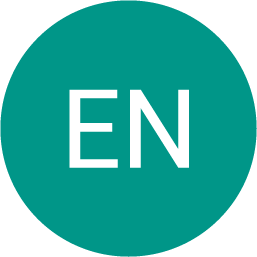

Mathematics, 20.11.2019 14:31
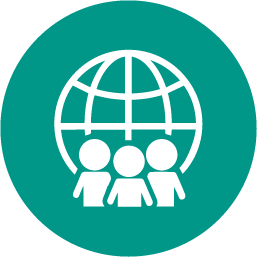
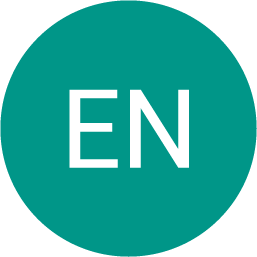
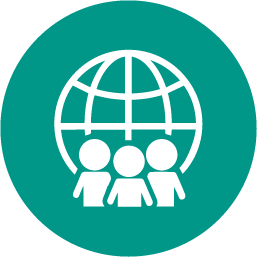
History, 20.11.2019 14:31
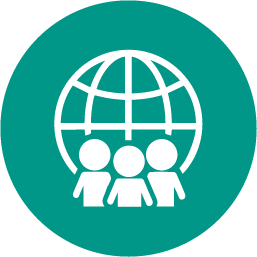
Social Studies, 20.11.2019 14:31

Mathematics, 20.11.2019 14:31

Mathematics, 20.11.2019 14:31

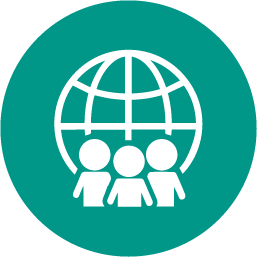
History, 20.11.2019 14:31

Mathematics, 20.11.2019 14:31