
Computers and Technology, 03.03.2020 16:34 gabrieljerron
Consider the following C++ program. It prompts the user for a number and calculates and prints the square root of the number using the "sqrt" function and the "pow" function.
#include
#include
using namespace std;
int main()
{
double num, root, error;
cout << "Enter number:\n";
cin >> num;
root = sqrt(num);
cout << "sqrt(" << num << ")= " << root << endl;
root = pow(num, 0.5);
cout << "pow(" << num << ",1/2)= " << root << endl;
return 0;
}
Step 1: Copy this program into your C++ program editor, and compile it. Hopefully you will not get any error messages.
Step 2: Run the program and type in the number "42" to see what it prints. You should see the square root of 42 calculated using the "sqrt" function. The second output line shows 42 raised to the power 1/2 which is another way to calculate the square root. Try several other input values. Do the two square root calculations always match each other?
Step 3: Type in "man sqrt" in your Linux window to see the manual page for this function. Near the top of the manual page you will see the function prototype "double sqrt(double x);". If you read the description you will see that the sqrt function expects one double parameter and returns a double value that is the "nonnegative square root of x".
Step 4: Type in "man pow" in your Linux window to see the manual page for this function. Near the top of the manual page you will see the function prototype "double pow(double x, double y);". If you read the description you will see that the pow function expects two double parameters and returns a double value that is equal to "x raised to the power of y".
Step 5: If you entered the number "4" above you saw that the square root was equal to 2, which is exactly correct. On the other hand, we can only approximate the square root of "3" using a double in C++ because the true answer has infinite length. To see what the error in this square root calculation is, copy/paste the following two lines after the "cout << sqrt" and "cout << pow" lines above.
error = num - root * root;
cout << "error= " << error << endl << endl;
Step 6: Compile and run your program and try a few different input values. What is your error when you input a perfect square like "49" as input? What is the error when you input a prime number like "3"? Is there any difference in error between "sqrt" and "pow"?
Step 7: Type in "man cbrt" in your Linux window to see the manual page for this function. Near the top of the manual page you will see the function prototype "double cbrt(double x);". If you read the description you will see that the cbrt function expects one double parameter and returns a double value that is the "real cube root of x".
Step 8: Your next task is to extend your program to calculate cube roots two ways and compare their errors. Edit your program and use copy/paste to duplicate your sqrt, pow and error calculations. Next, change sqrt to cbrt, and change the pow exponent to 1.0/3.0. Finally, modify the error calculations accordingly. When you compile and run your program with an input of "42", it should output the following:
sqrt(42)= 6.48074
error= 0
pow(42,1/2)= 6.48074
error= 0
cbrt(42)= 3.47603
error= 0
pow(42,1/3)= 3.47603
error= 0
Step 9: Run your new program several times with different input values. What happens if you input a negative value? What happens if you enter a 5 digit input value? Is sqrt(x) as accurate as pow(x,1/2)? Is cbrt(x) as accurate as pow(x,1/3)? For most applications these small errors are not an issue. On the other hand, if you are calculating the gross domestic product of the country or the trajectory of a rocket to mars, these differences DO matter.

Answers: 3
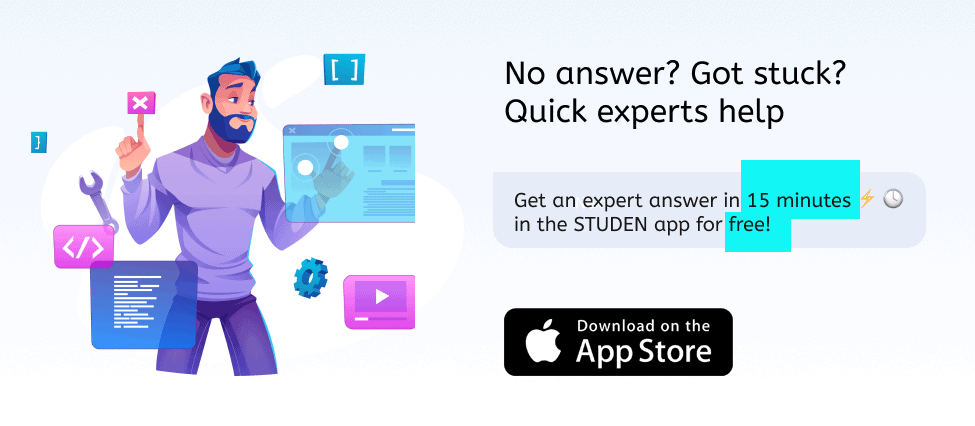
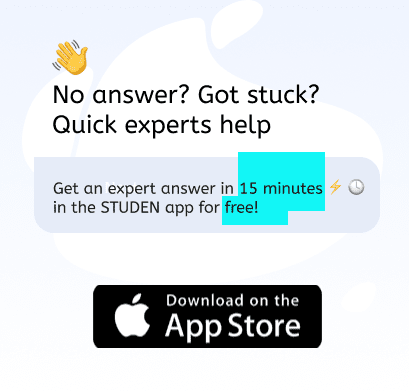
Another question on Computers and Technology

Computers and Technology, 22.06.2019 18:30
If an improvement creates no significant change in a product’s performance, then it is a(n) ? a0 design improvement. (there are no answer choices)
Answers: 1

Computers and Technology, 22.06.2019 18:30
Technician a says that a shop towel should never be used to clean around the flange area before replacing an automatic transmission filter. technician b says that a dimpled transmission fluid pan can be repaired. who is right
Answers: 3

Computers and Technology, 22.06.2019 22:40
When you type the pwd command, you notice that your current location on the linux filesystem is the /usr/local directory. answer the following questions, assuming that your current directory is /usr/local for each question. a. which command could you use to change to the /usr directory using an absolute pathname? b. which command could you use to change to the /usr directory using a relative pathname? c. which command could you use to change to the /usr/local/share/info directory using an absolute pathname? d. which command could you use to change to the /usr/local/share/info directory using a relative pathname? e. which command could you use to change to the /etc directory using an absolute pathname? f. which command could you use to change to the /etc directory using a relative pathname?
Answers: 3

Computers and Technology, 23.06.2019 07:30
What key should you press and hold to select and open multiple files at one time? enter alt control esc
Answers: 1
You know the right answer?
Consider the following C++ program. It prompts the user for a number and calculates and prints the s...
Questions

Mathematics, 28.08.2020 23:01
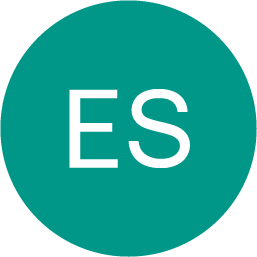
Spanish, 28.08.2020 23:01

Mathematics, 28.08.2020 23:01
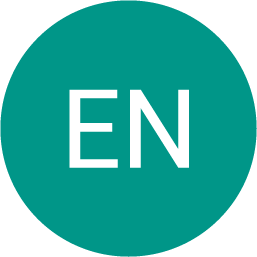

Mathematics, 28.08.2020 23:01


Mathematics, 28.08.2020 23:01
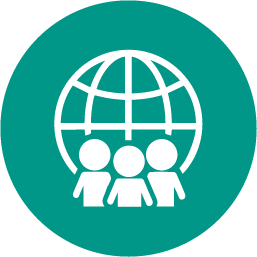


Mathematics, 28.08.2020 23:01

Mathematics, 28.08.2020 23:01

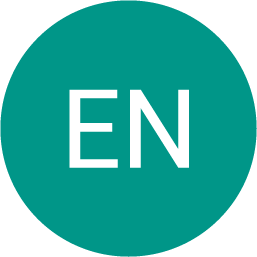
English, 28.08.2020 23:01

Mathematics, 28.08.2020 23:01

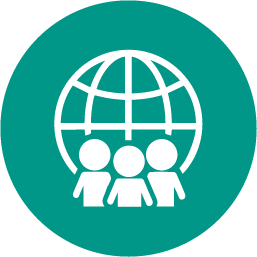
Social Studies, 28.08.2020 23:01

Mathematics, 28.08.2020 23:01


Mathematics, 28.08.2020 23:01
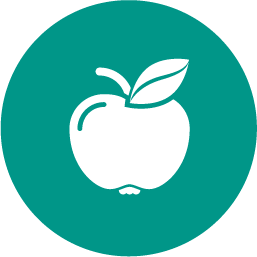
Physics, 28.08.2020 23:01