Define a very basic PCB structure:
struct PCB {int ProcId; int ProcPR, int CPUburst; int Reg[...

Computers and Technology, 10.03.2020 00:29 princessss30188
Define a very basic PCB structure:
struct PCB {int ProcId; int ProcPR, int CPUburst; int Reg[8]; int queueEnterClock, waitingTime; /* other info */ struct PCB *next;}
Define a very basic CPU consisting of 8 integer registers: int CPUreg[8] = {0};
Initialize your linked list: struct PCB *Head=NULL; struct PCB *Tail=NULL;
Initialize your other data: int CLOCK=0; int Total_waiting_time=0; int Total_turnaround_time=0; int Total_job=0;
Open the input file
For each input line, read a line consisting of three integers: Process Id, Process Priority, CPU Burst time.
dynamically create a struct PCB pointed by PCB,
save the given data into corresponding fields of PCB,
set all PCB->Reg[8] to the Process ID, set PCB->queueEnterClock and PCB->waitingTime to 0, then
insert this PCB at the end of the link list.
Close input file
Print your name, the name of the scheduling algorithm, and the input file name, as mentioned before.
The above steps will be the same for the next session too
Now implement a FIFO_Scheduling() function and call it to print the order of completed processes.
This function simply removes the PCB from the head of the linked list and performs the followings until the linked list is empty:
Do context-switching:
copy PCB->Reg[8] into CPUreg[8],
suppose some work is done on CPU (e. g, increment each CPUreg by one),
copy CPUreg[8] into PCB->Reg[8]
Data collection for performance metrics
PCB->waitingTime = PCB->waitingTime + CLOCK - PCB->queueEnterClock;
Total_waiting_time = Total_waiting_time + PCB->waitingTime ;
CLOCK = CLOCK + PCB->CPUburst;
Total_turnaround_time = Total_turnaround_time + CLOCK;
Total_job = Total_job + 1;
Free PCB. Since there is no more CPUburst or I/O burst, this process is terminated here! Otherwise, it will be put in a ready or waiting queue.
Finally, print the perfromance metrics mentioned before:
Total_waiting_time / Total_job,
Total_turnaround_time / Total_job,
Total_job / CLOCK
For each input file, copy/paste your screen output into a text file (say output1.txt)
input1.txt:
1 3 5
2 3 7
3 1 6
4 5 4

Answers: 1
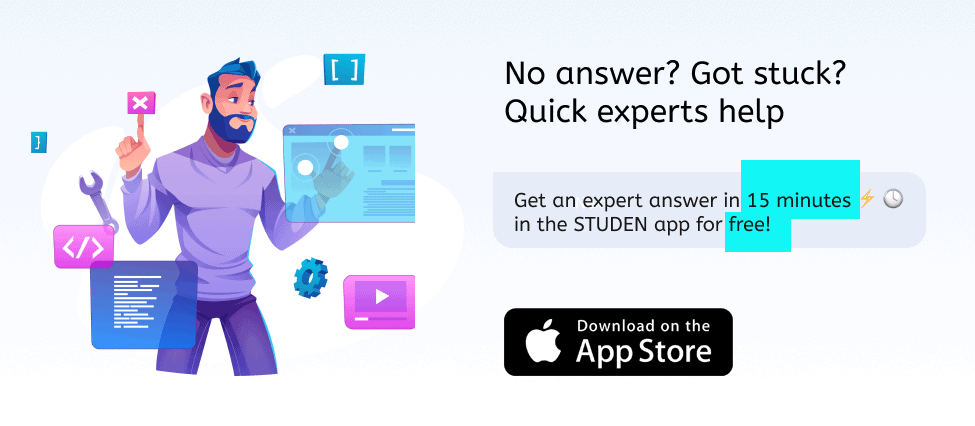
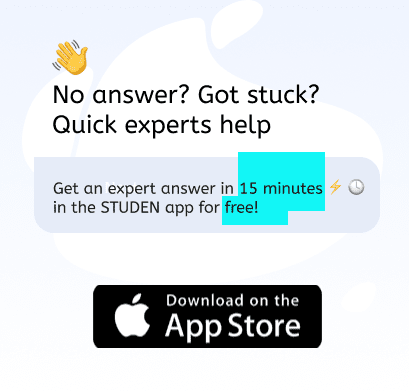
Another question on Computers and Technology

Computers and Technology, 21.06.2019 17:30
What is force? what are the types of force ? explain all with suitable examples
Answers: 1

Computers and Technology, 23.06.2019 19:50
Which feature is selected to practice and save the timing of a presentation
Answers: 1


Computers and Technology, 23.06.2019 23:30
Match the following errors with their definitions. a. #name b. #value c. #ref d. 1. when a formula produces output that is too lengthy to fit in the spreadsheet cell 2. when you enter an invalid cell reference in a formula 3. when you type text in cells that accept numeric data 4. when you type in a cell reference that doesn’t exist
Answers: 1
You know the right answer?
Questions


Mathematics, 18.03.2021 02:10
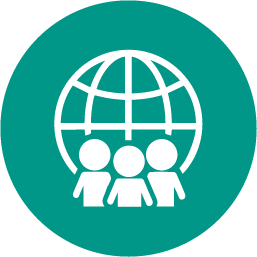
Social Studies, 18.03.2021 02:10



Business, 18.03.2021 02:10




Biology, 18.03.2021 02:10


Mathematics, 18.03.2021 02:10

Mathematics, 18.03.2021 02:10
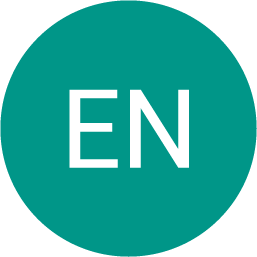
English, 18.03.2021 02:10

Biology, 18.03.2021 02:10

Mathematics, 18.03.2021 02:10

Chemistry, 18.03.2021 02:10

Health, 18.03.2021 02:10
