
Computers and Technology, 10.03.2020 08:56 cinthyafleitas
Complete the commented parts in the code below:
#include
#include
#include
// Functions
//
// Function : main
// Inputs : argc - the number of command line parameters
// argv - the parameters
// Outputs : 0 if successful test, -1 if failure
// Function : ???
// ???
int main(int argc, char *argv[]) {
// Local variables
// NOTE: this is where you will want to add some new variables
int int_array1[10], int_array2[10];
unsigned int uint_array1[10];
int i;
//
if (argc < 11)
{
printf("Exiting the program, missing input");
return 0;
}
// Step a - read in the integer numbers to process
for (i=1; i<11; i++) {
int_array1[i-1] = atoi(argv[i]);//converting input to integer
}
// Step b - Convert numbers into positive values by taking their
// absolute values and save them in int_array2.
// Print all numbers in a single line using display_array function
//
// Step c - Convert these positive integers to numbers
// in the range 0,…,128 by implementing the mod operation
// save them back into int_array2.
// Print all numbers in a single line using display_array function
//
// Step d - for each integer in int_array2 print:
// number, number of 1 bits, even or odd
//
// Step e - Cast each element of int_array2 to unsigned short
// and store them into uint_array1.
//
// Step f - Reverse the order of array elements in uint_array1
// using swap_int function.
/*swap_ints(): function should swap the numbers without using
temp variable*/
// Step g - Update each element of uint_array1 by using reverseBits function.
/* reverseBit(): The function should return the number (in
integer format) whose bits are reversed,
i. e., the top bit of the original number is
the bottom bit of the returned number, the
second from the top bit of the original
number is the second to the bottom bit of
the returned number. */
// Step h - Print each element of uint_array1 in a separate line along with
// binary representation of each of the numbers using binaryString function.
/* binaryString():This function should fill the text string
with a binary representation of the number
suitable for printing. You should be using
some bitwise operations to achieve this,
maybe shifting(<<) and and (&)
operation
*/
// Return successfully
return(0);
}

Answers: 3
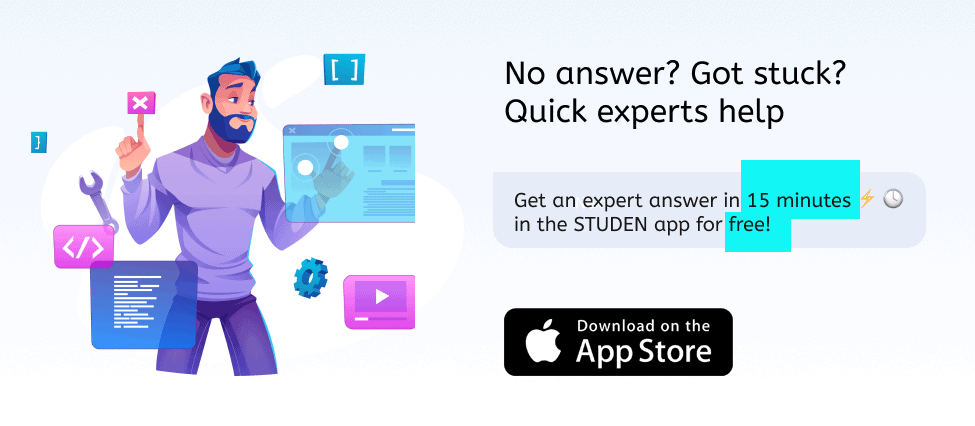
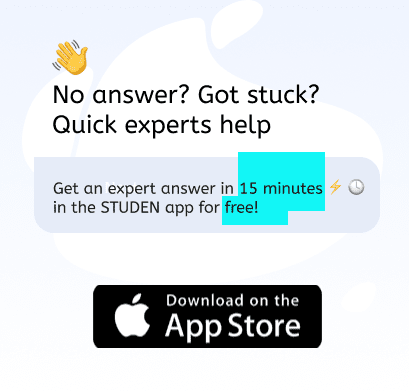
Another question on Computers and Technology

Computers and Technology, 22.06.2019 12:00
The following function returns a string of length n whose characters are all 'x'. give the order of growth (as a function of n) of the running time. recall that concatenating two strings in java takes time proportional to the sum of their lengths. public static string f(int n) { if (n == 0) return ""; if (n == 1) return "x"; return f(n/2) + f(n - n/2); } options: a) constant b) logarithmic c) linear d) linearithmic e)quadratic f)cubic g) exponential
Answers: 2

Computers and Technology, 22.06.2019 13:30
Use the keyword strategy to remember the meaning of the following word. the meaning for the word has been provided. write your keyword and describe the picture you would create in your mind. centurion: a commander in the army of ancient rome. keyword: picture:
Answers: 2

Computers and Technology, 23.06.2019 11:20
Http is the protocol that governs communications between web servers and web clients (i.e. browsers). part of the protocol includes a status code returned by the server to tell the browser the status of its most recent page request. some of the codes and their meanings are listed below: 200, ok (fulfilled)403, forbidden404, not found500, server errorgiven an int variable status, write a switch statement that prints out the appropriate label from the above list based on status.
Answers: 2

Computers and Technology, 24.06.2019 02:30
How to apply the fly in effect to objects on a slide
Answers: 1
You know the right answer?
Complete the commented parts in the code below:
#include
#include
#include...
#include
#include
#include...
Questions
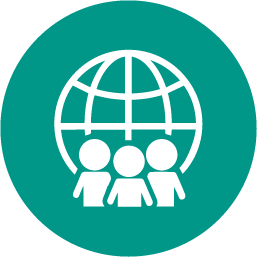

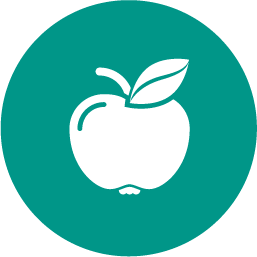
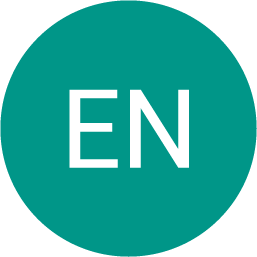

Computers and Technology, 28.08.2019 00:30
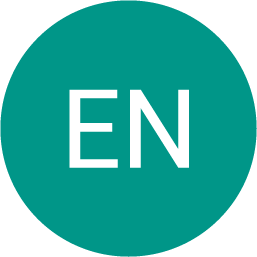


Biology, 28.08.2019 00:30



Mathematics, 28.08.2019 00:30

Mathematics, 28.08.2019 00:30

Mathematics, 28.08.2019 00:30

Mathematics, 28.08.2019 00:30

Mathematics, 28.08.2019 00:30

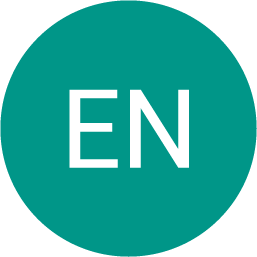
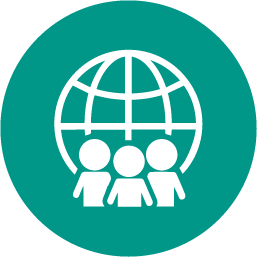
History, 28.08.2019 00:30
