
Computers and Technology, 11.03.2020 01:08 kcameronanderso
I need help in how to call a file in java: (file names are sample_input1.txt, sample_input2.txt, sample_input3.txt)
//These are all the imports you are allowed, don't add any more!
import java. util. Scanner;
import java. io. File;
import java. io. IOException;
class SimpleCompiler {
public static Node fileToQueue(String filename) throws IOException {
//given a file name, open that file in a scanner and create a queue of nodes
//the head of the queue of nodes should be the start of the queue
//the values in the nodes should be the strings read in each time you call
//next() on the scanner
return null;
}
public Node compile(Node input, int numSymbols) {
//Given an input queue of symbols, process the number of symbols
//specified (numSymbols) and update the callStack and symbols
//variables appropriately to reflect the state of the "SimpleCompiler"
//(see below the "do not edit" line for these variables).
//Return the remaining queue items.
//For example, if input is the head of a linked list 3 -> 2 -> +
//and numSymbols=2, you would push 3 and push 2, then return the linked
//list with just the + node remaining.
return null;
}
public static void testThisCode() {
//edit this as much as you want, if you use main without any arguments,
//this is the method that will be run instead of the program
System. out. println("You need to put test code in testThisCode() to run SimpleCompiler with no parameters.");
}
//DON'T EDIT BELOW THIS LINE
//EXCEPT TO ADD JAVADOCS
//don't edit these...
public static final String[] INT_OPS = {"+","-","*","/"};
public static final String[] ASSIGN_OPS = {"=","+=","-=","*=","/="};
//or these...
public CallStack callStack = new CallStack<>();
public LookUpBST symbols = new LookUpBST<>();
public static void main(String[] args) {
//this is not a testing main method, so don't edit this
//edit testThisCode() instead!
if(args. length == 0) {
testThisCode();
return;
}
if(args. length != 2 || !(args[1].equals("false") || args[1].equals("true"))) {
System. out. println("Usage: java SimpleCompiler [filename] [true|false]");
System. exit(0);
}
try {
(new SimpleCompiler()).runCompiler(args[ 0], args[1].equals("true"));
}
catch(IOException e) {
System. out. println(e. toString());
e. printStackTrace();
}
}
//provided, don't change this
public void runCompiler(String filename, boolean debug) throws IOException {
Node input = fileToQueue(filename);
System. out. println("\nProgram: " + Node. listToString(input));
if(!debug) {
while(input != null) {
input = compile(input, 10);
}
}
else {
Scanner s = new Scanner(System. in);
for(int i = 1; input != null; i++) {
System. out. println("\n Step " + i + " \n");
System. out. println("Step Output");
input = compile(input, 1);
System. out. println("Lookup BST");
System. out. println(symbols);
System. out. println("Call Stack");
System. out. println(callStack);
if(input != null) {
System. out. println("Program Remaining");
System. out. println(Node. listToString(input));
}
System. out. println("\nPress Enter to Continue");
s. nextLine();
}
}
}
}

Answers: 1
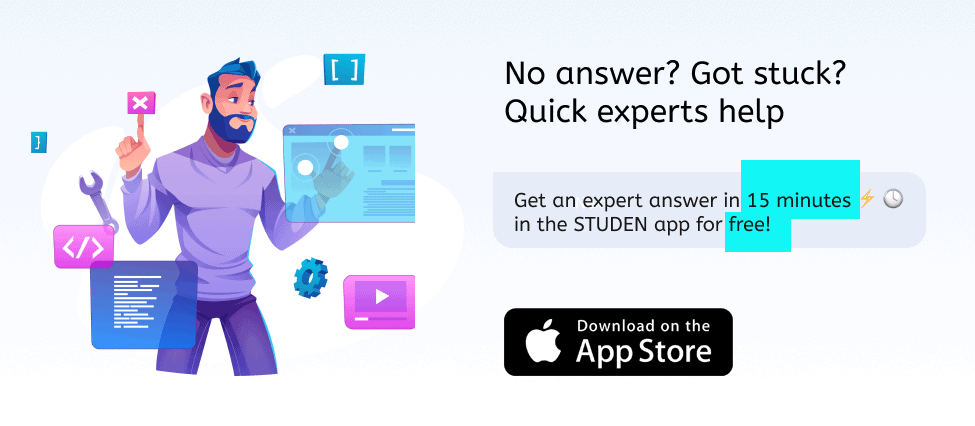
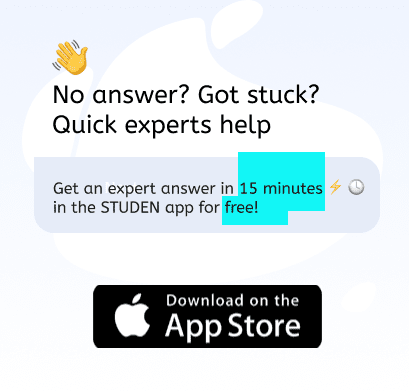
Another question on Computers and Technology

Computers and Technology, 23.06.2019 04:10
2pointswho was mikhail gorbachev? oa. a russian leader who opposed a coupob. a polish leader who founded the labor union "solidarityoc. a soviet leader who called for a closer relationship with the unitedstates, economic reform, and a more open societyd. a soviet leader who called for more oppression in the soviet union
Answers: 3

Computers and Technology, 23.06.2019 09:00
Which is the highest level of the hierarchy of needs model? a. humanity b. intrapersonal c. team d. interpersonal
Answers: 1

Computers and Technology, 24.06.2019 10:00
When writing a business letter, how many times can you use the same merge field in a document? once once, unless using the address block feature unlimited it will depend on the type of document you choose
Answers: 1

Computers and Technology, 24.06.2019 23:00
Systolic pressure is a measure of blood pressure when the ventricles relax and fil with blood ture or false
Answers: 1
You know the right answer?
I need help in how to call a file in java: (file names are sample_input1.txt, sample_input2.txt, sam...
Questions
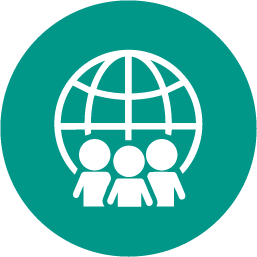
World Languages, 09.02.2021 02:50


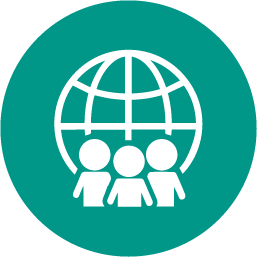
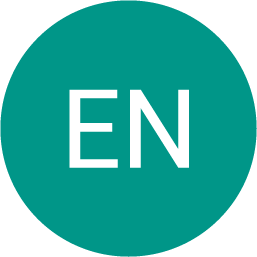

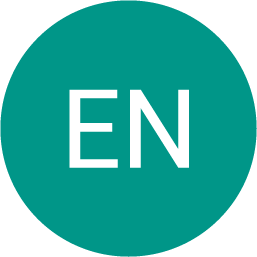

Mathematics, 09.02.2021 02:50
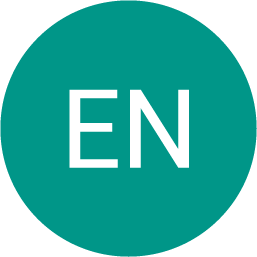

Biology, 09.02.2021 02:50
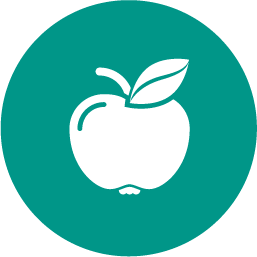

Mathematics, 09.02.2021 02:50



Mathematics, 09.02.2021 02:50


Mathematics, 09.02.2021 02:50


Mathematics, 09.02.2021 02:50