
Computers and Technology, 02.04.2020 16:59 ansley81
In this problem, you will implement various algorithms operating on binary search trees. We have provided with you a standard implementation of a generic BST in BinarySearchTree. java. Note that this class is an abstract class, which means that some of its methods are not implemented. In previous assignments, you have implemented interfaces which specified methods that you needed to write. Very similarly, an abstract class is a class with some unimplemented methods (it can be thought of somewhat like an interface but with some methods actually implemented). You will need to write a BetterBST class which extends BinarySearchTree. Your BetterBST class can then be treated just like a regular BinarySearchTree, just with some additional functionality. The methods that you will need to implement in BetterBST perform various algorithms on BST instances. For some of these methods, you may find it convenient to implement a private helper method as you did in previous assignments. public int height() - return the height of the BSTpublic T imbalance() - check whether the tree is balanced. A balanced tree is one where every node’s left and right subtrees differ in height by no more than 1. Return the value at first node you find which has a height imbalance greater than 1 between its subtrees, or null if no such node exists (i. e. the tree is balanced). In class, we discussed AVL trees, which enforce this balance condition. public T smallestGreaterThan(T t) - given some generic comparable value t, find the smallest value in the BST that is larger than t. For example, if a binary search tree contains the values 1, 3, and 5, and the function is called with t = 2, it should return 3.public BinarySearchTree mirror() - return a mirrored version of the BST instance to satisfy a reversed BST condition. In a reversed BST condition, for every node, X, in the tree, the values of all the items in its right subtree are smaller than the item in X, and the values of all the items in its left subtree are larger than the item in X. In the mirrored tree, any node that is a left child becomes a right child and vice versa. You should not modify the BST instance itself. Instead, you should create a new BST instance and build it. public void prettyPrint() - "pretty print" the binary tree. For example, given a tree with root = 3, left child = 1, right child = 5, and where the root’s right child has left child = 4, the pretty print could look something like: 3 1 5 4There is no required format for the pretty print, however, it must clearly look like a tree! (Hint: think about how you might use a queue to solve this problem.)Make sure you read the BST code in depth before you start implementing BetterBST. In particular, take note of the various internal methods, nested classes, and instance variables that you can access from BetterBST. We will test this program with our own tester class in a separate file. You should also create a tester class for your own testing purposes. Your tester class will not be graded.

Answers: 3
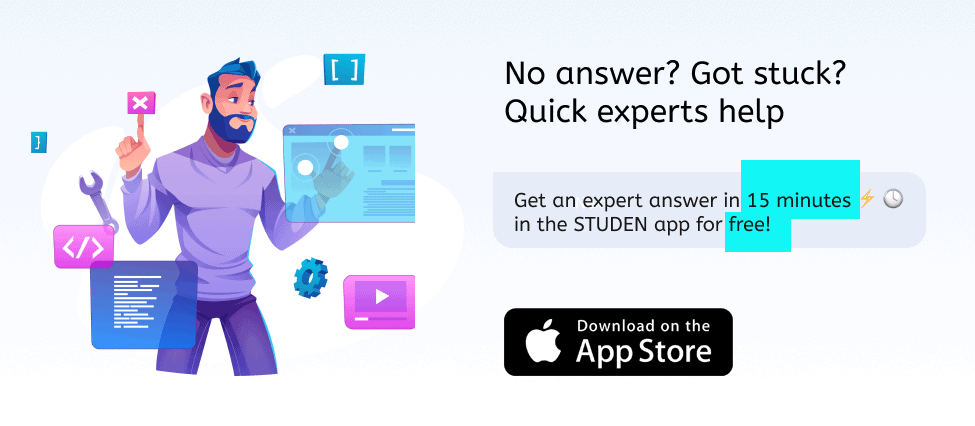
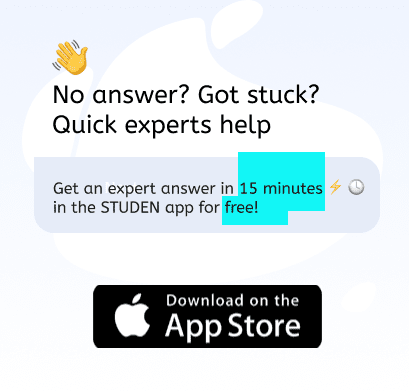
Another question on Computers and Technology

Computers and Technology, 22.06.2019 19:50
Write a car class having two private member variables called tank and speed. write public methods called pumpgas and gofast. the method pumpgas gets an integer for gas that must be pumped. that value needs to be added to tank (no more than 20 gallons). it must return the amount of gas that is purchased ($4 per gallon). the method gofast should increase the speed by 5 each time it is called.write a constructor for the above class that initialized both variables to zero.write a tostring to display both the tank and speed when the car is printed.modify the car class to implement the interface comparable and an interface called carinter having the public methods in carinter.write the main program to create an array of size 5 of type car. create 5 car objects having each location of the array to refer to one of the cars. test the pumpgas, gofast, equals method on the array items. write an enhanced loop to print all the car values (using a tostring written last time).write a generic method to find the minimum of four items. pass int, double, char, string and car objects to test this method.
Answers: 1

Computers and Technology, 23.06.2019 02:30
What is the power dissipated by a resistor with a current of 0.02 a and a resistance of 1,000 ? a. 200 w b. 20 w c. 0.4 w d. 4 w
Answers: 1

Computers and Technology, 23.06.2019 09:30
Write an essay on online collaboration, how to do it, the challenges, resolving the challenges, and consider whether the risks are greater than rewards. ( need )
Answers: 1

Computers and Technology, 23.06.2019 13:00
Which one of the following voltages should never be measured directly with a vom? a. 1200 v b. 500 v c. 800 v d. 100v
Answers: 2
You know the right answer?
In this problem, you will implement various algorithms operating on binary search trees. We have pro...
Questions
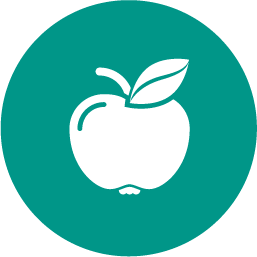

Computers and Technology, 25.12.2019 00:31
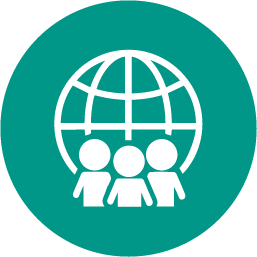

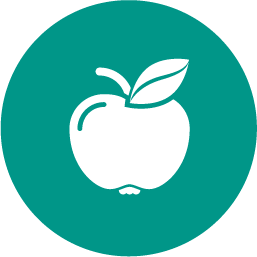

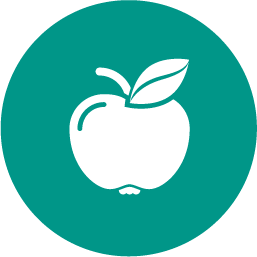



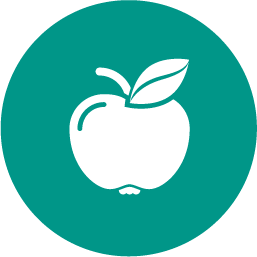





Computers and Technology, 25.12.2019 00:31


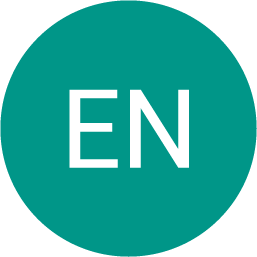