
Computers and Technology, 07.04.2020 02:12 Maddi7328
Create a class called Fraction. Provide a constructor that takes 2 integers. Provide methods for:
toString
setFraction
equals
add
subtract
multiple
divide
reduce - make sure you get the previous methods done before worrying about reduce.
The following will be your starting point:
package fractions;
import java. util.*;
public class Fraction
{
private Scanner scan = new Scanner(System. in);
private int num=1;
private int denom=1;
public Fraction()
{
}
public Fraction(int n, int d)
{
// Fill in code (good to use setFraction)
}
public void setFraction(int n, int d)
{
//Fill in code ... don't forget to reduce
}
public Fraction add(Fraction op)
{
//Fill in code ... don't forget to reduce
// Algebra HINT: a/b + c/d = (a*d + b*c)/(b*d)
}
public Fraction subtract(Fraction op)
{
//Fill in code ... don't forget to reduce
// Algebra HINT: a/b - c/d = (a*d - b*c)/(b*d)
}
public Fraction multiply(Fraction op)
{
//Fill in code ... don't forget to reduce
// Algebra HINT: a/b * c/d = (a*c)/ (b*d)
}
public Fraction divide(Fraction op)
{
//Fill in code ... don't forget to reduce
// Algebra HINT: a/b / c/d = (a*d)/ (b*c)
}
private void reduce()
{
// Pseudo code:
// set smaller = minimum ( abs(num), abs(denom));
// Loop through the possible divisors: 2, 3, 4, ... smaller
// For each possible divisor:
// while (num and denom are evenly divisible by divisor)
// {
// num /= divisor;
// denom /= divisor;
// smaller /= divisor;
// }
}
public boolean equals(Fraction f)
{
// Assuming all fractions are reduced
// Fill in code
}
public String toString()
{
// Fill in code
}
public void readin(String label)
{
while (true) // Keep trying if bad input is received
{
System. out. print(label);
String temp = scan. next();
temp = temp. trim(); // get rid of white space at the beginning and end
int index = temp. indexOf('/');
if (index >= 0)
{
String numStr = temp. substring(0, index);
String denomStr = temp. substring(index+1);
int n = Integer. parseInt(numStr);
int d = Integer. parseInt(denomStr);
setFraction(n, d);
return;
}
else
System. out. println("Input Fraction missing / ");
}//Keep trying until you get it right
}
public static void main(String[] args)
{
Fraction f1= new Fraction();
Fraction f2= new Fraction();
Fraction f3=null;
Scanner scan = new Scanner(System. in);
while(true)
{
System. out. println(); // Add a blank line
System. out. print("Enter operation: + - * / q (q ==> quit) : ");
String input = scan. next();
if (input. charAt(0) == 'q')
break; // All done
f1.readin("Enter Fraction 1: ");
f2.readin("Enter Fraction 2: ");
System. out. println("f1 = " + f1);
System. out. println("f2 = " + f2);
if (f1.equals(f2))
System. out. println("f1 and f2 are equal");
else
System. out. println("f1 and f2 are not equal");
switch (input. charAt(0))
{
case '+':
f3 = f1.add(f2);
System. out. println("f1+f2=" + f3);
break;
case '-':
f3 = f1.subtract(f2);
System. out. println("f1-f2=" + f3);
break;
case '*':
f3 = f1.multiply(f2);
System. out. println("f1*f2="+f3);
break;
case '/':
f3 = f1.divide(f2);
System. out. println("f1/f2="+f3);
break;
default:
System. out. println("Illegal command: " + input );
break;
}
}// end of while loop
System. out. println("Bye");
} // end of main
}

Answers: 3
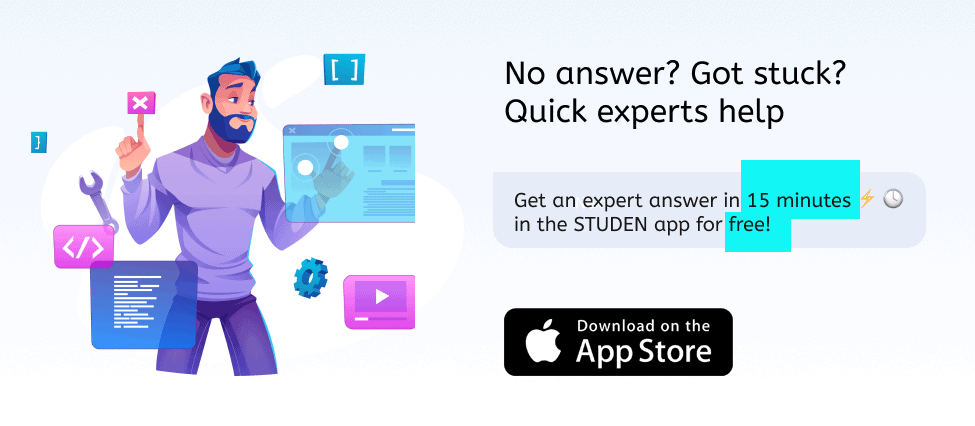
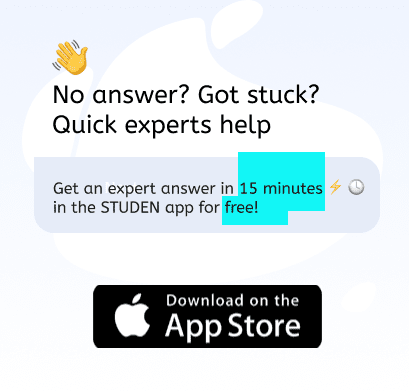
Another question on Computers and Technology

Computers and Technology, 22.06.2019 18:00
Which of the following physical laws can make the flow of water seem more realistic? a. motion b. gravity c. fluid dynamics d. thermodynamics
Answers: 2

Computers and Technology, 23.06.2019 08:00
What is a scenario where records stored in a computer frequently need to be checked
Answers: 2

Computers and Technology, 24.06.2019 03:30
It is not necessary to develop strategies to separate good information and bad information on the internet. true or false
Answers: 1

Computers and Technology, 24.06.2019 13:30
What process should be followed while giving a reference? sam has given a reference of his previous manager in his resume. sam should him in advance that the potential employers will him.
Answers: 1
You know the right answer?
Create a class called Fraction. Provide a constructor that takes 2 integers. Provide methods for:
Questions

Mathematics, 14.12.2020 22:30



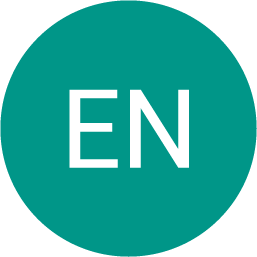


Arts, 14.12.2020 22:30
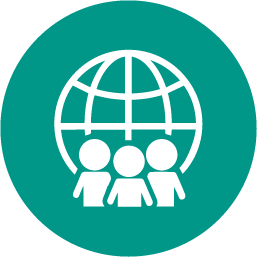

Chemistry, 14.12.2020 22:30


Chemistry, 14.12.2020 22:30
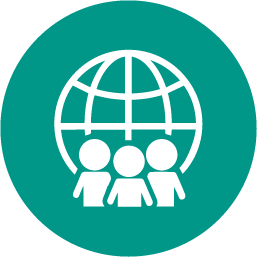
History, 14.12.2020 22:30
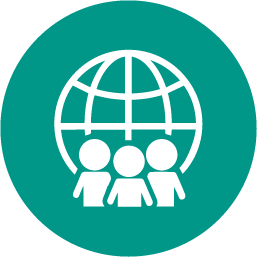

Mathematics, 14.12.2020 22:30
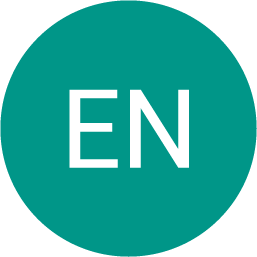
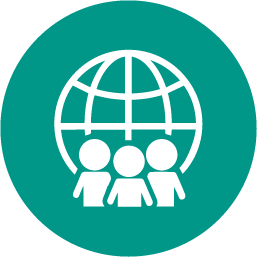


Mathematics, 14.12.2020 22:30
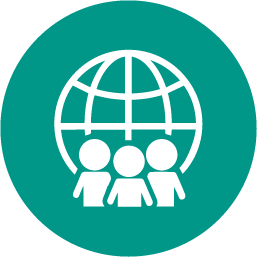