
Computers and Technology, 07.04.2020 20:25 takaylawynder
For this question, we will use the heap-supporting functions as seen in the lecture slides as building blocks for this assignment to build a new heap implementation. The heap implementation shown in the lecture slides is an example of a min-heap, in which the smallest element is at the root and all elements in child trees are larger than the value at the root. We can also construct max-heap data structures in which the largest element in the heap is at the root and all elements in child trees are smaller than the root.
The objective is to define SCHEME functions to manipulate a heap which:
1. maintain a binary tree as a heap,
2. use a generic (first order) order relation,
3. provides functions which can determine if a heap is empty? as well as heap-insert, heap-remove, and combine-heaps
(a) Define a SCHEME procedure, named (heap-insert f x H), which adds element x to heap H using the first-order relation f to determine which element belongs at the root of each (sub)tree.
For instance, if we wanted the same behavior as the heaps in the lecture slides (min-heap), we would use the "less than" function as our first-order relation:
(heap-insert < 100 (heap-insert < 10 (list))) (10 () (100 () ()))
If, instead, we wanted a max-heap implementation, where the largest element is at the root of the heap, we would use the "greater than" function as our first-order relation.
(heap-insert > 100 (heap-insert > 10 (list))) (100 () (10 () ()))
Note, you must use the same first-order relation for all of the heap procedures applied to a particular heap structure
HELPER FUNCTIONS
(define (create-heap value left right)
(list value left right))
(define (heap-root H) (car H))
(define (left T) (cadr T))
(define (right T) (caddr T))
(define (heap-insert f x H)
...)

Answers: 2
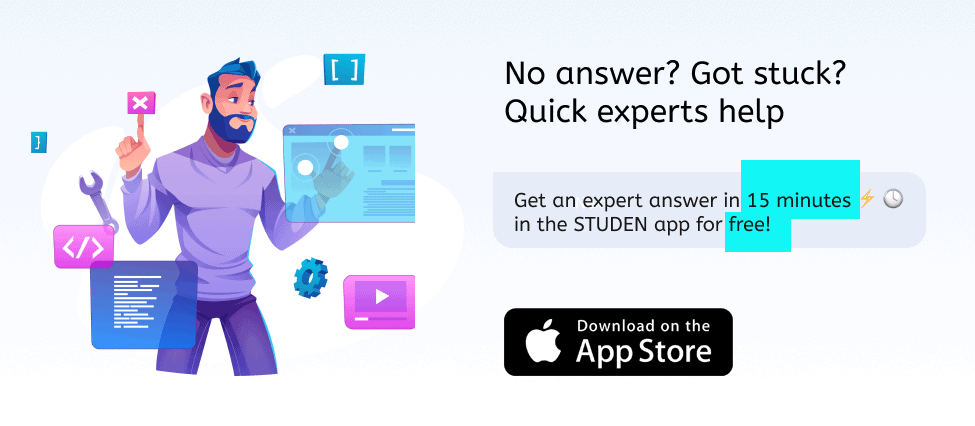
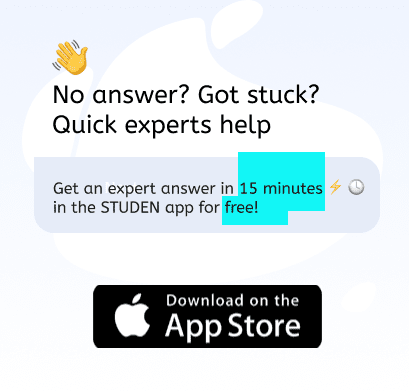
Another question on Computers and Technology

Computers and Technology, 22.06.2019 15:00
This is not a factor that you should use to determine the content of your presentation. your audience your goals your purpose your technology
Answers: 1

Computers and Technology, 23.06.2019 06:00
Respond to the following in three to five sentences. select the workplace skill, habit, or attitude described in this chapter that you believe is most important for being a successful employee.
Answers: 1

Computers and Technology, 23.06.2019 10:30
How would you categorize the software that runs on mobile devices? break down these apps into at least three basic categories and give an example of each.
Answers: 1

Computers and Technology, 24.06.2019 00:20
Describe a data structures that supports the stack push and pop operations and a third operation findmin, which returns the smallest element in the data structure, all in o(1) worst-case time.
Answers: 2
You know the right answer?
For this question, we will use the heap-supporting functions as seen in the lecture slides as buildi...
Questions

Mathematics, 28.01.2020 21:57
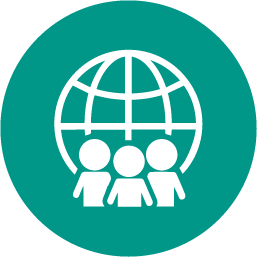
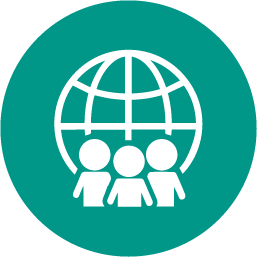
History, 28.01.2020 21:57
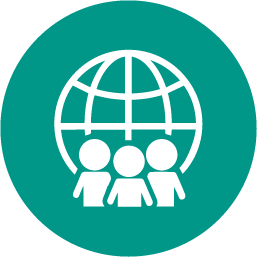
History, 28.01.2020 21:57
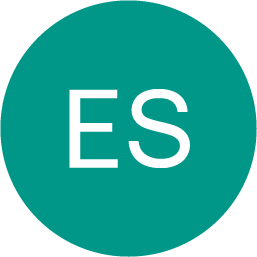
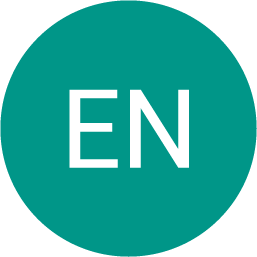
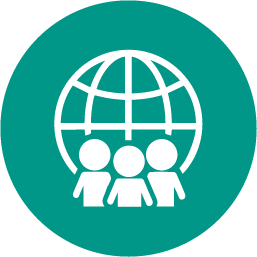
Social Studies, 28.01.2020 21:57

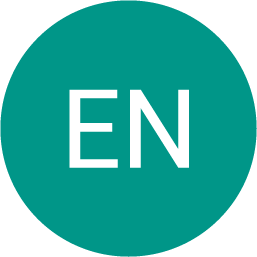
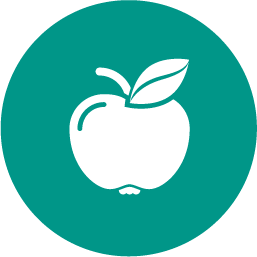

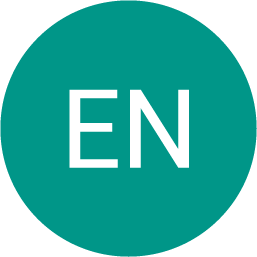

Mathematics, 28.01.2020 21:58
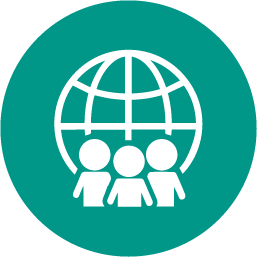
History, 28.01.2020 21:58

Mathematics, 28.01.2020 21:58

Business, 28.01.2020 21:58


Mathematics, 28.01.2020 21:58
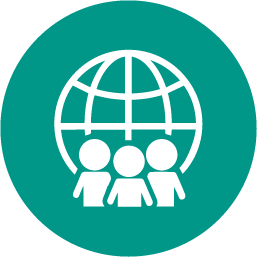
History, 28.01.2020 21:58