Modify the program to have a command to delete a reservation.
Modify the program to defi...

Computers and Technology, 07.04.2020 22:03 ElDudoso
Modify the program to have a command to delete a reservation.
Modify the program to define and use a method public void makeSeatReservations(Scanner scnr) so that the program's main() is cleaner. The makeSeatReservations() method should have a Scanner object passed to it to read the user's input.
import java. util. ArrayList;
import java. util. Scanner;
public class SeatReservation {
// Arraylist for seat reservations
private ArrayList allSeats;
public SeatReservation() {
allSeats = new ArrayList();
}
public void makeSeatsEmpty() {
int i;
for (i = 0; i < allSeats. size(); ++i) {
allSeats. get(i).makeEmpty();
}
}
public void printSeats() {
int i;
for (i = 0; i < allSeats. size(); ++i) {
System. out. print(i + ": ");
allSeats. get(i).print();
}
}
public void addSeats(int numSeats) {
int i;
for (i = 0; i < numSeats; ++i) {
allSeats. add(new Seat());
}
}
public Seat getSeat(int seatNum) {
return allSeats. get(seatNum);
}
public void setSeat(int seatNum, Seat newSeat) {
allSeats. set(seatNum, newSeat);
}
// Main method to use SeatReservation methods
public static void main (String [] args) {
Scanner scnr = new Scanner(System. in);
String usrInput = "";
String firstName, lastName;
int amountPaid;
int seatNum;
Seat newSeat;
SeatReservation ezReservations = new SeatReservation();
// Add 5 seat objects
ezReservations. addSeats(5);
// Make all seats empty
ezReservations. makeSeatsEmpty();
while (!usrInput. equals("q")) {
System. out. println();
System. out. print("Enter command (p/r/q): ");
usrInput = scnr. next();
// Print seats
if (usrInput. equals("p")) {
ezReservations. printSeats();
}
// Reserve a seat
else if (usrInput. equals("r")) {
System. out. print("Enter seat num: ");
seatNum = scnr. nextInt();
if ( !(ezReservations. getSeat(seatNum).isEmpty()) ) {
System. out. println("Seat not empty.");
}
else {
System. out. print("Enter first name: ");
firstName = scnr. next();
System. out. print("Enter last name: ");
lastName = scnr. next();
System. out. print("Enter amount paid: ");
amountPaid = scnr. nextInt();
// Create new Seat object and add to the reservations
newSeat = new Seat();
newSeat. reserve(firstName, lastName, amountPaid);
ezReservations. setSeat(seatNum, newSeat);
System. out. println("Completed.");
}
}
// FIXME: Add option to delete reservations
else if (usrInput. equals("q")) { // Quit
System. out. println("Quitting.");
}
else {
System. out. println("Invalid command.");
}
}
}
}

Answers: 1
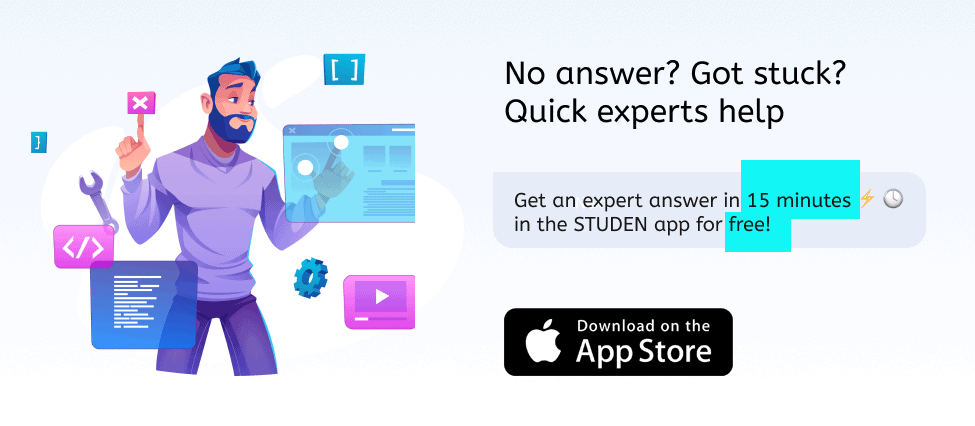
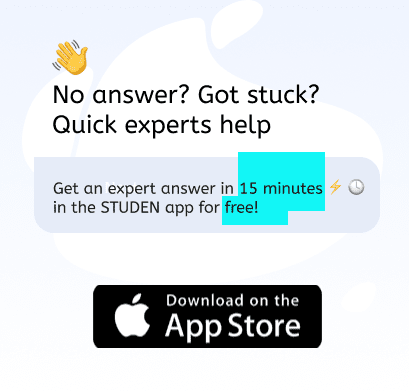
Another question on Computers and Technology

Computers and Technology, 22.06.2019 22:30
Who needs to approve a change before it is initiated? (select two.) -change board -client or end user -ceo -personnel manager -project manager
Answers: 1

Computers and Technology, 23.06.2019 04:31
Acloud service provider uses the internet to deliver a computing environment for developing, running, and managing software applications. which cloud service model does the provider offer? a. iaas b. caas c. maas d. paas e. saas
Answers: 1

Computers and Technology, 23.06.2019 15:20
An ou structure in your domain has one ou per department, and all the computer and user accounts are in their respective ous. you have configured several gpos defining computer and user policies and linked the gpos to the domain. a group of managers in the marketing department need different policies that differ from those of the rest of the marketing department users and computers, but you don't want to change the top-level ou structure. which of the following gpo processing features are you most likely to use? a, block inheritance b, gpo enforcement c, wmi filtering d, loopback processing
Answers: 3

Computers and Technology, 24.06.2019 00:50
3. what is the output of the following statements? temporary object1; temporary object2("rectangle", 8.5, 5); temporary object3("circle", 6, 0); temporary object4("cylinder", 6, 3.5); cout < < fixed < < showpoint < < setprecision(2); object1.print(); object2.print(); object3.print(); object4.print(); object1.set("sphere", 4.5, 0); object1.print();
Answers: 1
You know the right answer?
Questions

Mathematics, 18.03.2021 04:20


Mathematics, 18.03.2021 04:20


Mathematics, 18.03.2021 04:20
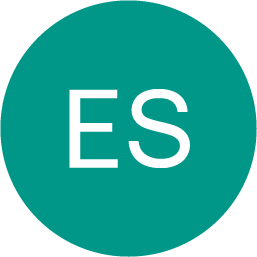
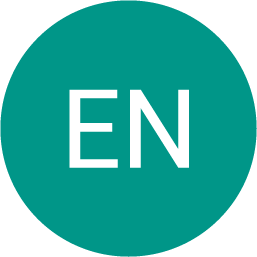


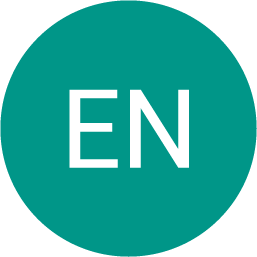

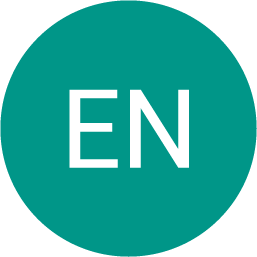

Mathematics, 18.03.2021 04:20

Chemistry, 18.03.2021 04:20
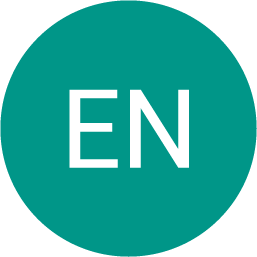
English, 18.03.2021 04:20


Biology, 18.03.2021 04:20

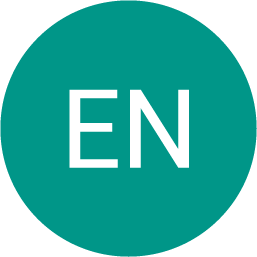