
Computers and Technology, 14.04.2020 22:33 zylovesnesha
In this problem, you will write three methods to:
1) Create a string array containing permutations of strings from two String array parameters.
2) Concatenate three String arrays using the method from part (a).
3) Print a String array, using an enhanced for loop (for-each).
For the examples given below, first is an array of first names, middle is an array of middle names, and last is an array of last names.
Assume that ALL names are different.
(a) Write the method makeNames that creates and returns a String array of new names based on the method's two-parameter arrays, array1 and array2.
The method creates new names in the following fashion: for each string in array1, concatenate a String from array2. Add a character space between the two strings:
array[i] + " " + array2[j]
In the example below the array names contains 20 names including "David A", "David B", "David C",..., "Lucy E".
String[] first = {"David", "Mike", "Katie", "Lucy" };
String[] middle = "A", "B", "C", "D", "E");
String[] names = makeNames (first, middle);
If one of the parameter arrays has size 0, return the other parameter array.
In the example below the array names contains 4 names: "David", "Mike", "Katie", "Lucy".
String[] first = {"David", "Mike", "Katie", "Lucy" };
String[] middle = {};
String names = makeNames (first, middle);
Use the method header:
public static String[] makeNames (String[] arrayı, String[] array2)
(b) Write an overloaded method makeNames that creates and returns a new String array of new names based on three input String arrays. makeNames will make new names using the method from part (a) without using loops. Each element in the returned array will be a concatenation of three strings: a string from the first parameter, a string from the second parameter, and a string from the third parameter.
In the example below the array names contains 40 names including "David A Green", "David B Green", "David C Green", ..., "Lucy E Wong".
String[] first = {"David", "Mike", "Katie", "Lucy" };
String[] middle = {"A", "B", "C", "D", "E");
String[] last = {"Green", "Wong"};
String[] names - makeNames (first, middle, last);
In the example below the array names contains 20 names including "David A", "David B", "David C", ..., "Lucy E".
String[] first = { "David", "Mike", "Katie", "Lucy" };
String[] middle = {"A", "B", "C", "D", "E");
String[] last = {};
String[] names - makeNames (first, middle, last);
Use the method header:
public static String[] makeNames (String[] arrayı, String[ array2, String[] array3)
Do not use loops to implement the method. Instead, call the method you have written in the part (a) of this problem. Assume that the method you wrote in part (a) works as intended. Credit will not be given for this part of the problem if you reimplement any part of the code you wrote in part (a).

Answers: 2
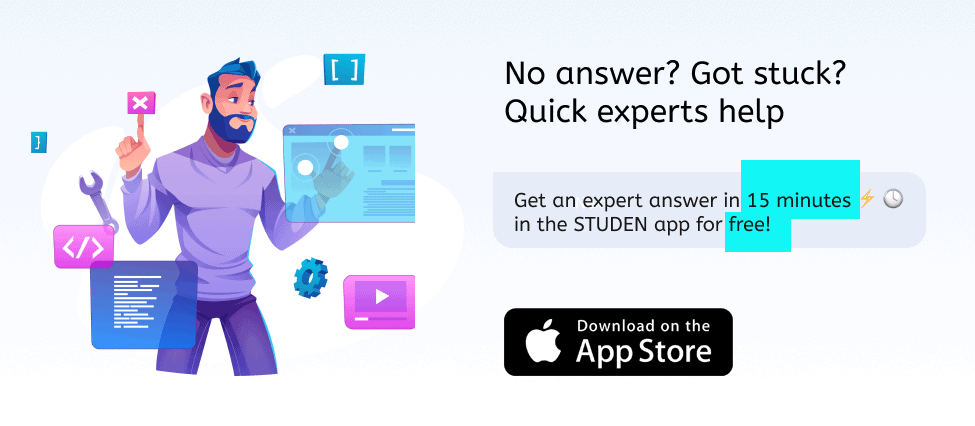
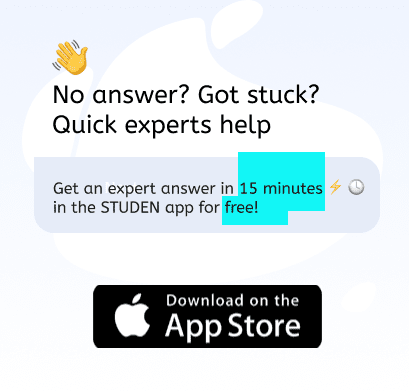
Another question on Computers and Technology

Computers and Technology, 22.06.2019 17:30
Ou listened to a song on your computer. did you use hardware or software?
Answers: 2

Computers and Technology, 23.06.2019 22:30
Apart from confidential information, what other information does nda to outline? ndas not only outline confidential information, but they also enable you to outline .
Answers: 1

Computers and Technology, 24.06.2019 13:30
In the rgb model, which color is formed by combining the constituent colors? a) black b) brown c) yellow d) white e) blue
Answers: 1

Computers and Technology, 24.06.2019 22:00
According to your study unit, what is the main reason that improved human relations skills may improve your grades?
Answers: 1
You know the right answer?
In this problem, you will write three methods to:
1) Create a string array containing permuta...
1) Create a string array containing permuta...
Questions

Mathematics, 20.12.2019 06:31
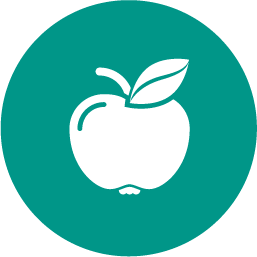
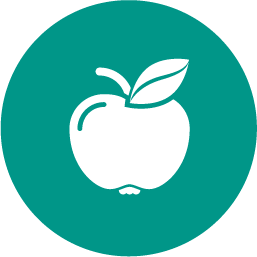



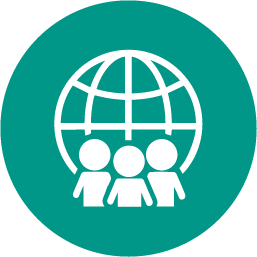




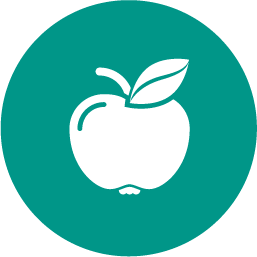

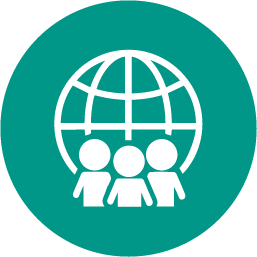


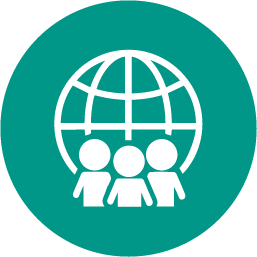

