
Computers and Technology, 16.04.2020 16:06 Nowellkwaku
In this lab, you will learn the basics of socket programming for TCP connections in Python: how to create a socket, bind it to a specific address and port, as well as send and receive a HTTP packet. You will also learn some basics of HTTP header format.
You will develop a web server that handles one HTTP request at a time. Your web server should accept and parse the HTTP request, get the requested file from the server’s file system, create an HTTP response message consisting of the requested file preceded by header lines, and then send the response directly to the client. If the requested file is not present in the server, the server should send an HTTP "404 Not Found" message back to the client.
Code
Below you will find the skeleton code for the Web server. You are to complete the skeleton code. The places where you need to fill in code are marked with #Fill in start and #Fill in end. Each place may require one or more lines of code.
Running the Server
Put an HTML file (e. g., HelloWorld. html) in the same directory that the server is in. Run the server program. Determine the IP address of the host that is running the server (e. g., 128.238.251.26). From another host or the same host if you run the server on the local manchine, open a browser and provide the corresponding URL. For example:
http://128.238.251.26:6789/HelloWor ld. html
‘HelloWorld. html’ is the name of the file you placed in the server directory. Note also the use of the port number after the colon. You need to replace this port number with whatever port you have used in the server code. In the above example, we have used the port number 6789. The browser should then display the contents of HelloWorld. html. If you omit ":6789", the browser will assume port 80 and you will get the web page from the server only if your server is listening at port 80.
You can also run the server program on your own PC and access the webserver from the same PC using the IP address for local host (127.0.0.1). For example:
http://127.0.0.1:6789/HelloWorld. html
Then try to get a file that is not present at the server. You should get a "404 Not Found" message.
What to Hand in
You will hand in the complete server code along with the screen shots of your client browser, verifying that you actually receive the contents of the HTML file from the server.
*** the source file (.py) of your web server ***
Skeleton Python Code for the Web Server (Use the code on the next page as the skeleton of your .py file and insert lines of code needed between #Fill in state and #Fill in end. Please note that Python programs get structured through indentation, copy and paste may change the indentation of the following lines. You are suggested to use Python 3.x.
#import socket module
import socket
import sys
serversocket = socket. socket(socket. AF_INET, socket. SOCK_STREAM)
print("Socket Created!!")
try:
#bind the socket
#fill in start
#fill in end
except socket. error as msg:
print("Bind failed. Error Code: " + str(msg[0]) + "Message: " + msg[1])
sys. exit()
print("Socket bind complete")
#start listening on the socket
#fill in start
#fill in end
print('Socket now listening')
while True:
#Establish the connection
connectionSocket, addr = #fill in start #fill in end
print('source address:' + str(addr))
try:
#Receive message from the socket
message = #fill in start #fill in end
print('message = ' + str(message))
#obtian the file name carried by the HTTP request message
filename = message. split()[1]
print('filename = ' + str(filename))
f = open(filename[1:], 'rb')
outputdata = f. read()
#Send the HTTP response header line to the socket
#fill in start
#fill in end
#Send the content of the requested file to the client
connectionSocket. send(outputdata)
#close the connectionSocket
#fill in start
#fill in end
print("Connection closed!")
except IOError:
#Send response message for file not found
#fill in start
#fill in end
#Close the client socket
#fill in start
#fill in end
serverSocket. close()

Answers: 1
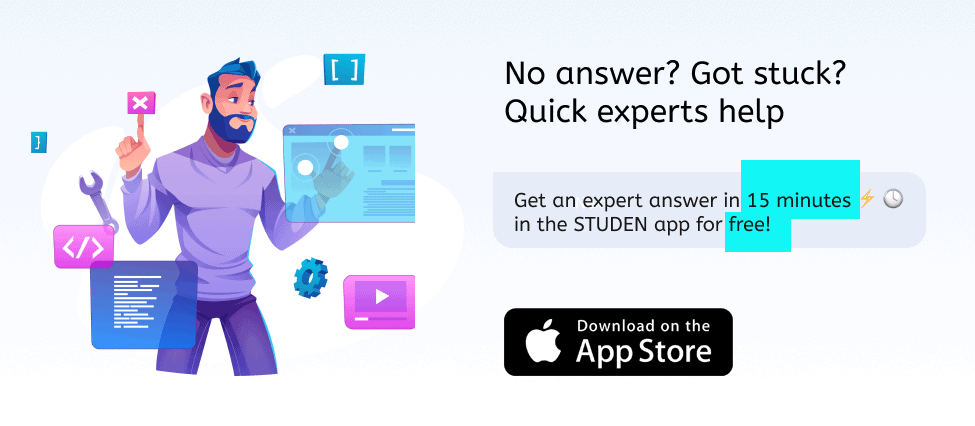
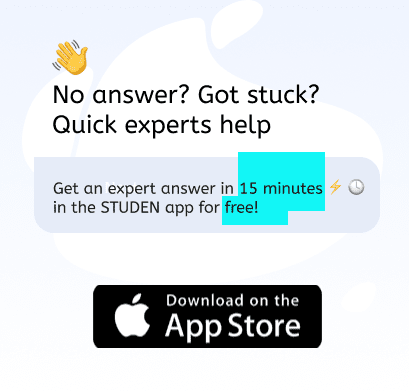
Another question on Computers and Technology

Computers and Technology, 22.06.2019 14:40
For this assignment you have to write a c program that will take an infix expression as input and display the postfix expression of the input. after converting to the postfix expression, the program should evaluate the expression from the postfix and display the result. what should you submit? write all the code in a single file and upload the .c file. compliance with rules: ucf golden rules apply towards this assignment and submission. assignment rules mentioned in syllabus, are also applied in this submission. the ta and instructor can call any students for explaining any part of the code in order to better assess your authorship and for further clarification if needed. problem: we as humans write math expression in infix notation, e.g. 5 + 2 (the operators are written in-between the operands). in computer's language, however, it is preferred to have the operators on the right side of the operands, ie. 5 2 +. for more complex expressions that include parenthesis and multiple operators, a compiler has to convert the expression into postfix first and then evaluate the resulting postfix write a program that takes an "infix" expression as input, uses stacks to convert it into postfix expression, and finally evaluates it. it must support the following operations: + - / * ^ % ( example infix expression: (7-3)/(2+2) postfix expression: 7 3 2 2 result: rubric: 1) if code does not compile in eustis server: 0. 2) checking the balance of the parenthesis: 2 points 3) incorrect postfix expression per test case: -2 points 4) correct postfix but incorrect evaluation per test case: -i points 5) handling single digit inputs: maximum 11 points 6) handling two-digit inputs: 100 percent (if pass all test cases)
Answers: 3

Computers and Technology, 23.06.2019 01:30
Which tab is used to change the theme of a photo album slide show? a. design b. view c. transitions d. home
Answers: 1

Computers and Technology, 23.06.2019 01:50
Free points just awnser this. what should i watch on netflix
Answers: 2

Computers and Technology, 23.06.2019 02:00
Consider the following function main: int main() { int alpha[20]; int beta[20]; int matrix[10][4]; . . } a. write the definition of the function inputarray that prompts the user to input 20 numbers and stores the numbers into alpha. b. write the definition of the function doublearray that initializes the elements of beta to two times the corresponding elements in alpha. make sure that you prevent the function from modifying the elements of alpha. c. write the definition of the function copyalphabeta that stores alpha into the first five rows of matrix and beta into the last five rows of matrix. make sure that you prevent the function from modifying the elements of alpha and beta. d. write the definition of the function printarray that prints any onedimensional array of type int. print 15 elements per line. e. write a c11 program that tests the function main and the functions discussed in parts a through d. (add additional functions, such as printing a two-dimensional array, as needed.)
Answers: 3
You know the right answer?
In this lab, you will learn the basics of socket programming for TCP connections in Python: how to c...
Questions
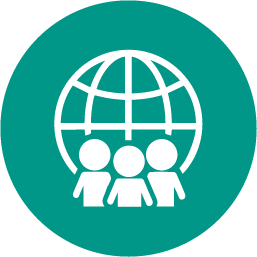
Social Studies, 09.04.2021 18:10
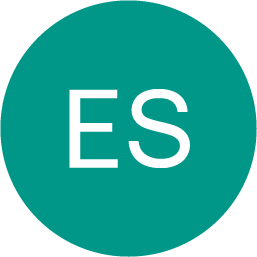
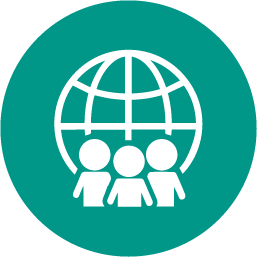

Mathematics, 09.04.2021 18:10

Biology, 09.04.2021 18:10


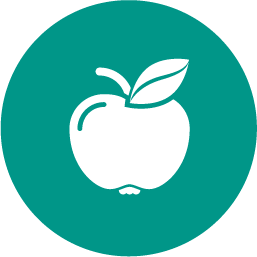

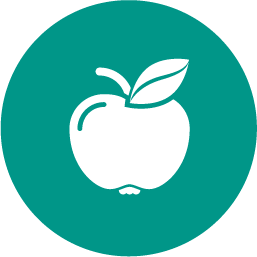

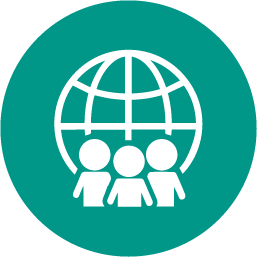

Mathematics, 09.04.2021 18:10
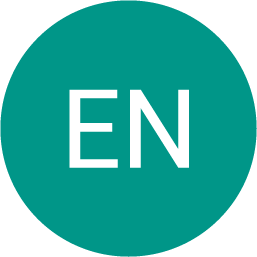



Mathematics, 09.04.2021 18:10

Mathematics, 09.04.2021 18:10
