
Computers and Technology, 17.04.2020 23:14 chem1014
For this exercise, you will be simulating a weather station responsible for recording hourly
temperatures and reporting on certain characteristics (e. g., average temperature) from your recordings.
You will also be expanding on your experience in creating functions and passing them more complex
parameters (i. e., complete array arguments).
Specifications:
DDI&T a C++ program to input, store, and process hourly temperatures for each hour of the day
(i. e., 24 temperatures). Your program should be divided logically into the following parts:
1. In function main() declare an array:
array HourlyTemperatures;
(where NUM_TEMPERATURES should be declared as a constant with the value, 24), that
will be used to hold one temperature for each hour of the day. Declare other variables and
constants as you feel are necessary.
2. Pass the HourlyTemperatures array to a function,
void GetTemperatures(array & Temperatures);
This function must interactively prompt for and input temperatures for each of the 24 hours
in a day (0 through 23). For each temperature that is input, verify that its value lies in the
range of minus 50 degrees and plus 130 degrees (i. e., validate your input values). If any
value is outside the acceptable range, ask the user to re-enter the value until it is within this
range before going on to the next temperature (HINT: use a nested loop (e. g., do-while) that
exits only when a value in the specified range has been entered). Store each validated
temperature in its corresponding element of the 24-element integer array passed as a
parameter to the function.
3. Next pass the filled array to a function,
double ComputeAverageTemp(array & Temperatures);
This function computes the average temperature of all the temperatures in the array and
returns this average to the calling function.
4. Finally, pass your array and the computed average temperature to another function,
void DisplayTemperatures(array & Temperatures,
double AverageTemp);
which displays the values of your temperature array in a columnar format followed by the
values for the high temperature, low temperature, and average temperature for the day.
NOTE: If you want to create separate function(s) to find and return the high and low
temperature values, then feel free to do so!
The resulting output should look like this:
Hour Temperature
00:00 42
01:00 42
. . . . . . . . // Your program output must include all of these too!
22:00 46
23:00 48
High Temperature: 68
Low Temperature: 42
Average Temperature: 57.4
5. Since you have created functions to perform each of the functional steps of your solution,
your main() function should be quite simple. The pseudo code for main() might look
something like this:
int main()
{
// declare local constant(s), variable(s), and array(s).
do
{
// call GetTemperatures(HourlyTemperatures) ;
// call ComputeAverageTemp(HourlyTemperatur es);
// call DisplayTemperatures(HourlyTemperatu res, AverageTemperature);
} while // user wants to process more days of temperatures
return 0;
}
6. Test your program with at least two (2) different sets of temperatures. Make sure you
enter values to adequately test your temperature-validation code (e. g., temperatures below β
50 and above +130 degrees).
Deliverable(s):
Turn in the properly documented source listing of your program and complete outputs from
at least TWO (2) test "runs". Additionally, turn in screen captures of some of your interactive inputs
demonstrating that your program properly detects invalid inputs and prompts the user to re-enter the
temperature(s). Starting with this exercise you must document your solution in accordance with the
examples shown at the back of this syllabus and provided on the Portal.

Answers: 1
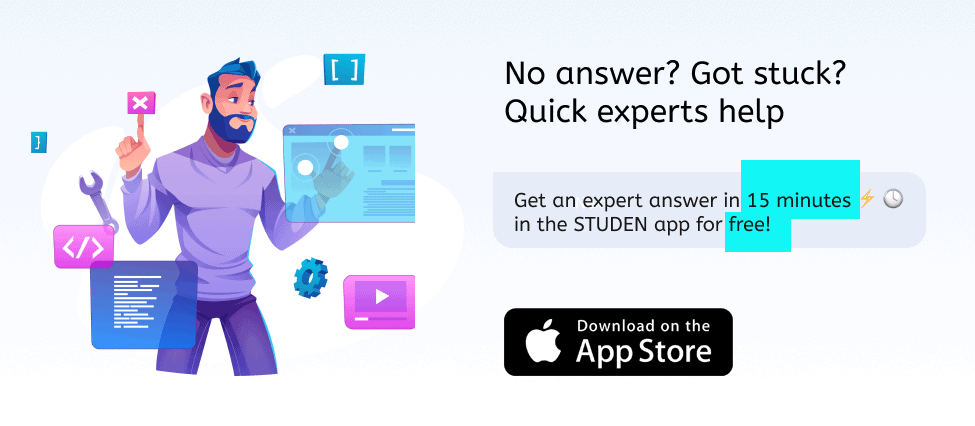
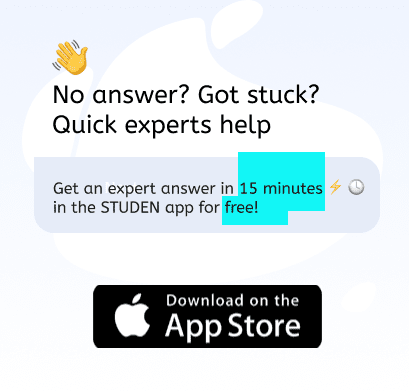
Another question on Computers and Technology

Computers and Technology, 22.06.2019 16:30
Primary tech skills are skills that are necessary for success in online education
Answers: 3

Computers and Technology, 23.06.2019 03:50
Q-1 which of the following can exist as cloud-based it resources? a. physical serverb. virtual serverc. software programd. network device
Answers: 1

Computers and Technology, 23.06.2019 14:30
Select the correct answer. andy received a potentially infected email that was advertising products. andy is at risk of which type of security threat? a. spoofing b. sniffing c. spamming d. phishing e. typo-squatting
Answers: 2

Computers and Technology, 24.06.2019 07:20
Ingrid started speaking about her slide presentation. when she clicked to th"third slide, which had just a picture of an elephant, she forgot what she wassupposed to talk about. what could ingrid do to avoid this situation in thefuture? oa. print handouts for her audience.ob. add presenter's notes to each slide.oc. add a video to each slide.od. save her slide presentation to a flash drive
Answers: 2
You know the right answer?
For this exercise, you will be simulating a weather station responsible for recording hourly
Questions
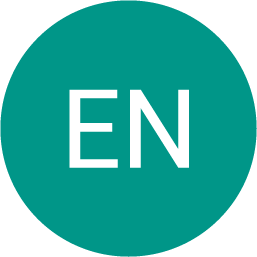
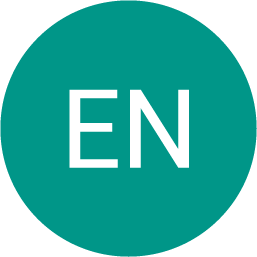
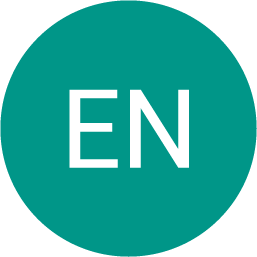
English, 15.05.2021 02:50


Health, 15.05.2021 02:50

Mathematics, 15.05.2021 02:50
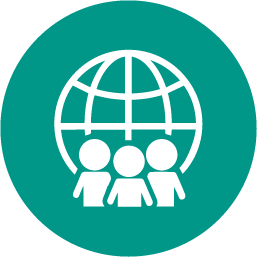
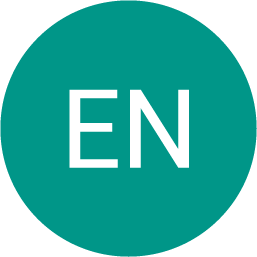
English, 15.05.2021 02:50

Chemistry, 15.05.2021 02:50

Mathematics, 15.05.2021 02:50
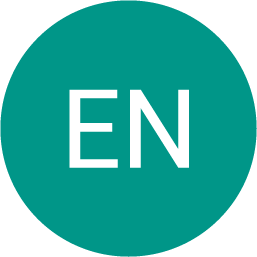
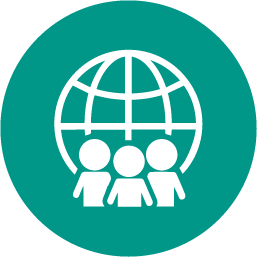

Computers and Technology, 15.05.2021 02:50
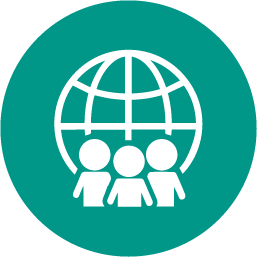

Mathematics, 15.05.2021 02:50

Mathematics, 15.05.2021 02:50
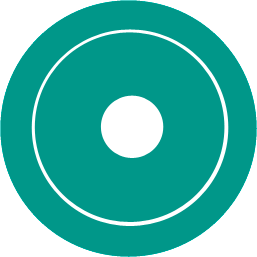
Advanced Placement (AP), 15.05.2021 02:50

Mathematics, 15.05.2021 02:50

Business, 15.05.2021 02:50