
Computers and Technology, 05.05.2020 09:33 thebasedgodchri
C++ Project
1) Prompt the user to enter a string of their choosing (Hint: you will need to call the getline() function to read a string consisting of white spaces.) Store the text in a string. Output the string. (1 pt)
Ex:
Enter a sample text:
We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue! You entered: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue!
(2) Implement a PrintMenu() function, which has a string as a parameter, outputs a menu of user options for analyzing/editing the string, and returns the user's entered menu option. Each option is represented by a single character. If an invalid character is entered, continue to prompt for a valid choice. Hint: Implement Quit before implementing other options.
Call PrintMenu() in the main() function. Continue to call PrintMenu() until the user enters q to Quit.
More specifically, the PrintMenu() function will consist of the following steps:
-print the menu
-receive an end user's choice of action (until it's valid)
-call the corresponding function based on the above choice
Ex:
MENU
c - Number of non-whitespace characters
w - Number of words
f - Find text
r - Replace all !'s
s - Shorten spaces
q - Quit
Choose an option:
(3) Implement the GetNumOfNonWSCharacters() function. GetNumOfNonWSCharacters() has a constant string as a parameter and returns the number of characters in the string, excluding all whitespace. Call GetNumOfNonWSCharacters() in the PrintMenu() function.
Ex:
Number of non-whitespace characters: 181
(4) Implement the GetNumOfWords() function. GetNumOfWords() has a constant string as a parameter and returns the number of words in the string. Hint: Words end when a space is reached except for the last word in a sentence. Call GetNumOfWords() in the PrintMenu() function.
Ex:
Number of words:35
(5) Implement the FindText() function, which has two strings as parameters. The first parameter is the text to be found in the user provided sample text, and the second parameter is the user provided sample text. The function returns the number of instances a word or phrase is found in the string. In the PrintMenu() function, prompt the user for a word or phrase to be found and then call FindText() in the PrintMenu() function. Before the prompt, call cin. ignore() to allow the user to input a new string.
Ex:
Enter a word or phrase to be found:
more
"more" instances: 5
(6) Implement the ReplaceExclamation() function. ReplaceExclamation() has a string parameter and updates the string by replacing each '!' character in the string with a '.' character. ReplaceExclamation() DOES NOT output the string. Call ReplaceExclamation() in the PrintMenu() function, and then output the edited string.
Ex.
Edited text: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue.
(7) Implement the ShortenSpace() function. ShortenSpace() has a string parameter and updates the string by replacing all sequences of 2 or more spaces with a single space. ShortenSpace() DOES NOT output the string.

Answers: 2
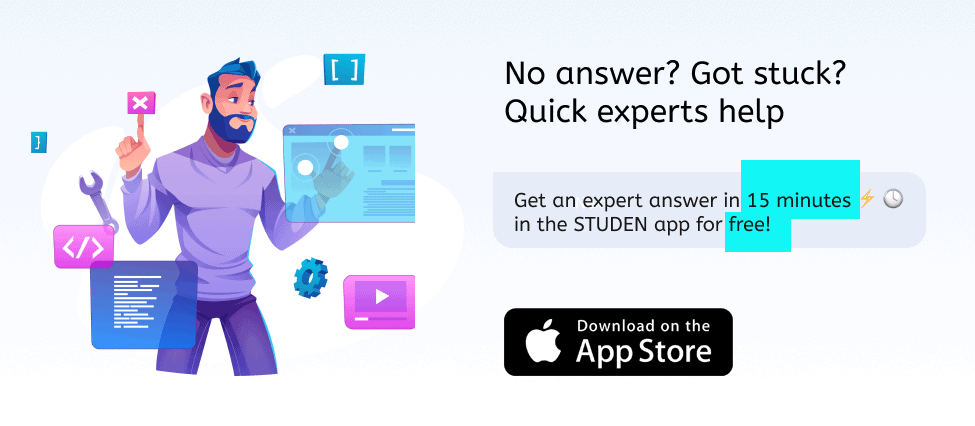
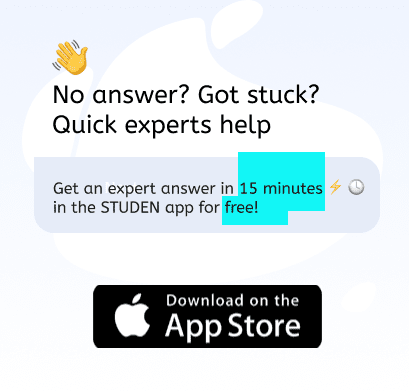
Another question on Computers and Technology

Computers and Technology, 22.06.2019 05:00
Which two editions of windows 7 support 64 bit cpus? choose two out of professional, business, starter, or home premium.
Answers: 1

Computers and Technology, 22.06.2019 11:30
Write a function so that the main program below can be replaced by the simpler code that calls function original main program: miles_per_hour = float( minutes_traveled = float( hours_traveled = minutes_traveled / 60.0 miles_traveled = hours_traveled * miles_per_hour print('miles: %f' % miles_traveled) sample output with inputs: 70.0 100.0 miles: 116.666667
Answers: 3


Computers and Technology, 23.06.2019 18:00
File account.java (see previous exercise) contains a definition for a simple bank account class with methods to withdraw, deposit, get the balance and account number, and return a string representation. note that the constructor for this class creates a random account number. save this class to your directory and study it to see how it works. then write the following additional code: 1. suppose the bank wants to keep track of how many accounts exist. a. declare a private static integer variable numaccounts to hold this value. like all instance and static variables, it will be initialized (to 0, since itΓ’β¬β’s an int) automatically. b. add code to the constructor to increment this variable every time an account is created. c. add a static method getnumaccounts that returns the total number of accounts. think about why this method should be static - its information is not related to any particular account. d. file testaccounts1.java contains a simple program that creates the specified number of bank accounts then uses the getnumaccounts method to find how many accounts were created. save it to your directory, then use it to test your modified account class.
Answers: 3
You know the right answer?
C++ Project
1) Prompt the user to enter a string of their choosing (Hint: you will need...
1) Prompt the user to enter a string of their choosing (Hint: you will need...
Questions

Mathematics, 22.10.2020 01:01

Mathematics, 22.10.2020 01:01

Mathematics, 22.10.2020 01:01

Mathematics, 22.10.2020 01:01
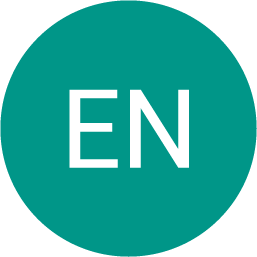
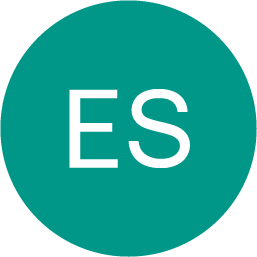
Spanish, 22.10.2020 01:01
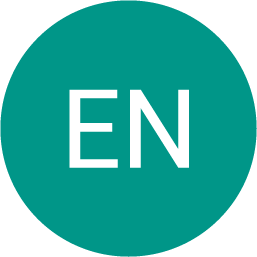
English, 22.10.2020 01:01
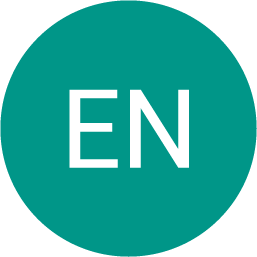

Biology, 22.10.2020 01:01

Mathematics, 22.10.2020 01:01

Medicine, 22.10.2020 01:01


Mathematics, 22.10.2020 01:01

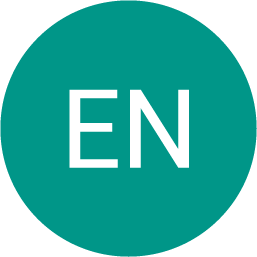

Mathematics, 22.10.2020 01:01

Biology, 22.10.2020 01:01

Mathematics, 22.10.2020 01:01

Mathematics, 22.10.2020 01:01