
Computers and Technology, 01.07.2020 15:01 patriciaceaser2980
Requirements:
The goal of assignment 3 is to reinforce the dynamic classes in C++. Specifically, the assignment is to implement the polynomial class from chapter 4.6 on page 212. But the additional requirement is to implement the class using a dynamic array to store the coefficients. The class needs to have a destructor, copy constructor, and an overloaded assignment operator. The following files are provided for your reference. You should start by modifying the header file first.
// FILE: poly0.h
// CLASS PROVIDED:
// class polynomial (in the namespace main_savitch_3)
// A polynomial has one variable x, real number coefficients, and
// non-negative integer exponents. Such a polynomial can be viewed
// as having the form:
// A[n]*x^n + A[n-1]*x^(n-1) + ... A[2]*x^2 + A[1]*x + A[0]
// where the A[n] are the real number coefficients and x^i represents
// the variable x raised to the i power. The coefficient A[0] is
// called the "constant" or "zeroth" term of the polynomial.
// // NOTES TO STUDENT:
// 1. This version works by storing the coefficients in
// a fixed array. The coefficient for the x^k term is stored
// in location [k] of the fixed-size array. Later we will modify
// the implementation to use a dynamic array.
// 2. Note that two functions have been implemented as inline functions
// in this file (the degree and operator() functions).
// 3. An implementation of the make_gif function is available for
// students to use at www. cs. colorado. edu/~main/projects/polygif. cxx.//
// MEMBER CONSTANTS
// const static size_t CAPACITY
// The size of the fixed array to store the coefficients.
// const static size_t MAX_EX = CAPACITY - 1;
// The maximum exponent permitted.
CONSTRUCTOR for the polynomial class
// polynomial(double c = 0.0, unsigned int exponent = 0)
// PRECONDITION: exponent <= MAX_EX.
// POSTCONDITION: This polynomial has been create with all zero
// coefficients, except for coefficient c for the specified exponent.
// When used as a default constructor (using default values for
// both arguments), the result is a polynomial with all zero
// coefficients.//
// MODIFICATION MEMBER FUNCTIONS for the polynomial class
// void add_to_coef(double amount, unsigned int exponent)
// PRECONDITION: exponent <= MAX_EX.
// POSTCONDITION: Adds the given amount to the coefficient of the
// specified exponent.
void assign_coef(double coefficient, unsigned int exponent)
// PRECONDITION: exponent <= MAX_EX.
// POSTCONDITION: Sets the coefficient for the specified exponent.//
// void clear( )
// POSTCONDITION: All coefficients of this polynomial are set to zero.
CONSTANT MEMBER FUNCTIONS for the polynomial class
// double coefficient(unsigned int exponent) const
// POSTCONDITION: Returns coefficient at specified exponent of this
// polynomial.
// NOTE: for exponents > MAX_EX, the return value is always zero.
unsigned int degree( ) const
// POSTCONDITION: The function returns the value of the largest exponent
// with a non-zero coefficient.
// If all coefficients are zero, then the function returns zero.//
// polynomial derivative( ) const
// POSTCONDITION: The return value is the first derivative of this
// polynomial.//
// double eval(double x) const
// POSTCONDITION: The return value is the value of this polynomial with the
// given value for the variable x.
unsigned int next_term(unsigned int e) const
// POSTCONDITION: The return value is the next exponent n which is LARGER
// than e such that coefficient(n) != 0.
// If there is no such term, then the return value is zero.//
// unsigned int previous_term(unsigned int e) const
// POSTCONDITION: The return value is the next exponent n which is SMALLER
// than e such that coefficient(n) != 0.
// If there is no such term, then the return value is UINT_MAX
// from .
CONSTANT OPERATORS for the polynomial class
// double operator( ) (double x) const
// Same as the eval member function.

Answers: 1
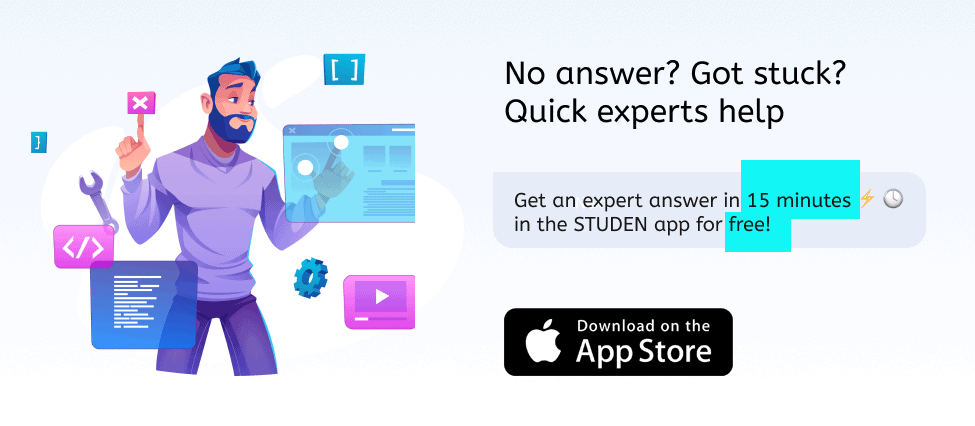
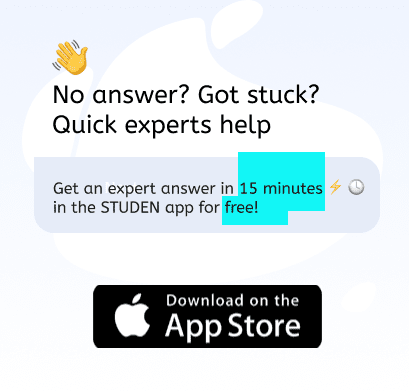
Another question on Computers and Technology

Computers and Technology, 22.06.2019 03:00
You install teamviewer on your workstation at home so that you can ac ess it when on the road. how can you be assured that unknown users cant access your computer through team viewer?
Answers: 2

Computers and Technology, 22.06.2019 15:00
Which of the following statements tests if students have a grade of 70 or above, as well as fewer than five absences? a: if(grade > = 70 and daysabsent < = 5): b: if(grade > = 70 or daysabsent < = 5): c: if(grade > 70 and daysabsent < = 5): d: if(grade > 70 or daysabsent < = 5): i took the test the answer is a
Answers: 1

Computers and Technology, 22.06.2019 17:40
Gabe wants to move text from one document to another document. he should copy the text, paste the text, and open the new document highlight the text, select the cut command, move to the new document, make sure the cursor is in the correct location, and select the paste command select the save as command, navigate to the new document, and click save highlight the text, open the new document, and press ctrl and v
Answers: 1

Computers and Technology, 23.06.2019 17:10
Ac++an of of pi. in , pi is by : pi = 4 β 4/3 + 4/5 β 4/7 + 4/9 - 4/11 + 4/13 - 4/15 + 4/17 . ., to pi (9 ). , if 5 to pi,be as : pi = 4 - 4/3 + 4/5 - 4/7 + 4/9 = 4 β 1. + 0.8 - 0. + 0. = 3.. atoofbe to pi?
Answers: 2
You know the right answer?
Requirements:
The goal of assignment 3 is to reinforce the dynamic classes in C++. Specifically, th...
Questions
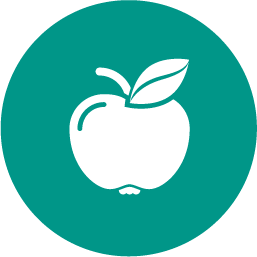



Biology, 02.03.2020 23:25


Mathematics, 02.03.2020 23:25

Computers and Technology, 02.03.2020 23:25
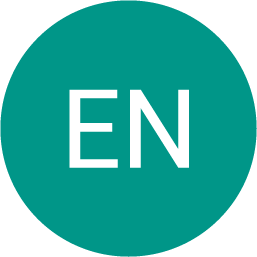
English, 02.03.2020 23:25

Mathematics, 02.03.2020 23:25

Mathematics, 02.03.2020 23:25



Mathematics, 02.03.2020 23:26

Computers and Technology, 02.03.2020 23:26

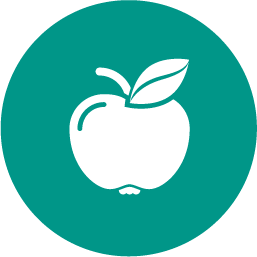

Mathematics, 02.03.2020 23:26

Mathematics, 02.03.2020 23:26
