
Computers and Technology, 04.07.2020 01:01 asiababbie33
Create a function(Python) called `addressbook` that takes as input two dictionaries, `name_to_phone` and `name_to_address`, and combines them into a single dictionary `address_to_all` that contains the phone number of, and the names of all the people who live at, a given address. Specifically, your function should:
1. Have input arguments `addressbook(name_to_phone, name_to_address)`, expecting `name_to_phone` as a dictionary mapping a name (string) to a home phone number (integer or string), and `name_to_address` as a dictionary mapping a name to an address (string).
2. Create a new dictionary `address_to_all` where the keys are all the addresses contained in `name_to_address`, and the value `address_to_all[address]` for `address` is of the format `([name1,name2,...], phone)`, with `[name1,name2,...]` being the list of names living at `address` and `phone` being the home phone number for `address`. **Note**: the *value* we want in this new dictionary is a *tuple*, where the first element of the tuple is a *list* of names, and the second element of the tuple is the phone number. (Remember that while a tuple itself is immutable, a list within a tuple can be changed dynamically.)
3. Handle the case where multiple people at the same address have different listed home phone numbers as follows: Keep the first number found, and print warning messages with the names of each person whose number was discarded.
4. Return `address_to_all`.
For example, typing in
name_to_phone = {'alice': 5678982231, 'bob': '111-234-5678', 'christine': 5556412237, 'daniel': '959-201-3198', 'edward': 5678982231}
name_to_address = {'alice': '11 hillview ave', 'bob': '25 arbor way', 'christine': '11 hillview ave', 'daniel': '180 ways court', 'edward': '11 hillview ave'}
address_to_all = addressbook(name_to_phone, name_to_address)
print(address_to_all)
```
should return
```
Warning: christine has a different number for 11 hillview ave than alice. Using the number for alice.
{'11 hillview ave': (['alice', 'christine', 'edward'], 5678982231), '25 arbor way': (['bob'], '111-234-5678'), '180 ways court': (['daniel'], '959-201-3198')}
```Your message should match exactly as shown above. If more than one person has a different phone number at the same address then use a `,` to separate the names.
Note that the specific order you get these elements back may not be the same, because sets and dictionaries do not preserve order. That is OK!

Answers: 3
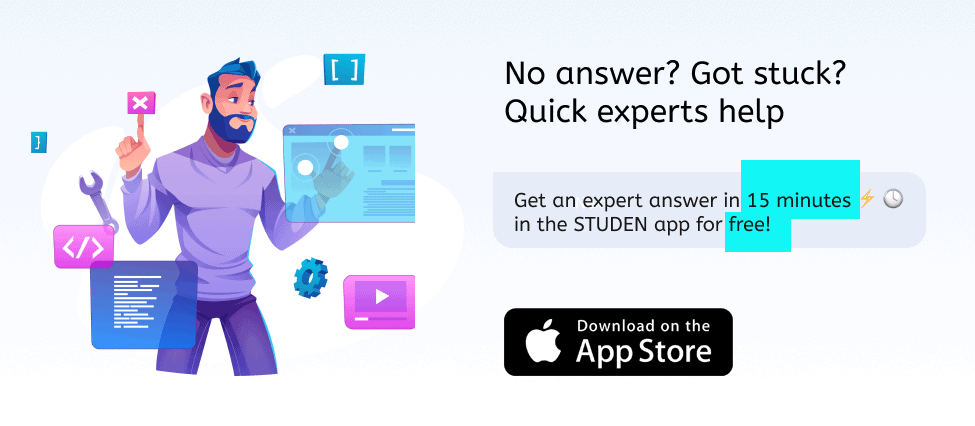
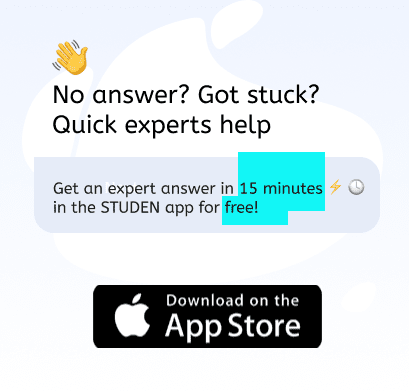
Another question on Computers and Technology

Computers and Technology, 21.06.2019 14:00
What does a sperm cell plus egg cell equal in total?
Answers: 1

Computers and Technology, 22.06.2019 04:30
What kind of software users of all skill levels create web pages that include graphics, video, audio, animation, and other special effects? website authoring website software website publishing website editing
Answers: 1

Computers and Technology, 23.06.2019 12:00
What does the level 1 topic in a word outline become in powerpoint? a. first-level bullet item b. slide title c. third-level bullet item d. second-level bullet item
Answers: 1

Computers and Technology, 24.06.2019 00:40
What is the error in the following pseudocode? module main() call raisetopower(2, 1.5) end module module raisetopower(real value, integer power) declare real result set result = value^power display result end module
Answers: 1
You know the right answer?
Create a function(Python) called `addressbook` that takes as input two dictionaries, `name_to_phone`...
Questions

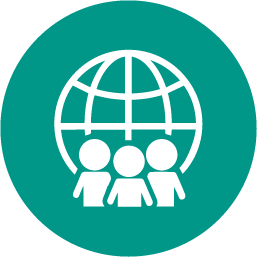
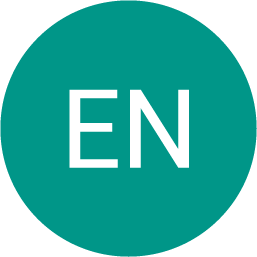
English, 23.03.2021 07:50
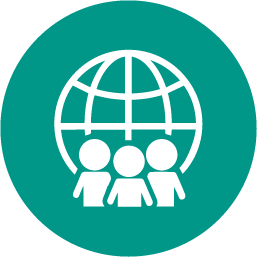
History, 23.03.2021 07:50


Mathematics, 23.03.2021 07:50

Mathematics, 23.03.2021 07:50



Mathematics, 23.03.2021 07:50

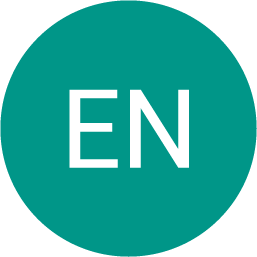
English, 23.03.2021 07:50
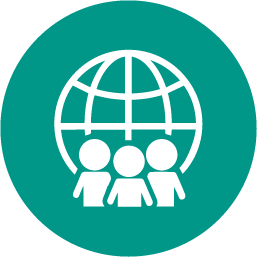
History, 23.03.2021 07:50

Mathematics, 23.03.2021 07:50
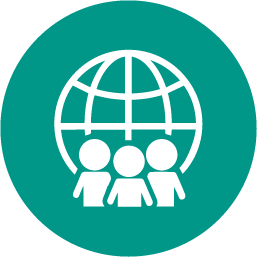
History, 23.03.2021 07:50

Mathematics, 23.03.2021 07:50



Mathematics, 23.03.2021 07:50

Mathematics, 23.03.2021 07:50