
Computers and Technology, 28.07.2020 20:01 vannybelly83
Create an application that reads a file that contains an email list, reformats the data, and writes the cleaned list to another file.
Below are the grading criteria…
Fix formatting. The application should fix the formatting problems.
Write the File. Your application should write a file named prospects_clean. csv.
Use title case. All names should use title case (an initial capital letter with the rest lowercase).
Fix email addresses. All email addresses should be lowercase.
Trim spaces. All extra spaces at the start or end of a string should be removed.
Here is the code work I was working on. It seems that there are errors happening and I get message that file is not found when using this code…
import java. io. IOException;
import java. io. BufferedReader;
import java. io. FileReader;
import java. util. ArrayList;
public class CSVReader {
// Used to read CSV file
public static ArrayList readCSV(String fileName) throws IOException {
String line = " ";
BufferedReader br = null;
ArrayList ar = new ArrayList();
br = new BufferedReader(new FileReader(fileName));
// Reading content from CSV file
while ((line = br. readLine()) != null) {
ar. add(line);
}
// Closing Buffered Reader
br. close();
return ar;
}
}
import java. util. ArrayList;
public class Cleaner {
// Convert to title case
static String convert(String str) {
str = str. toLowerCase();
char[] array = str. toCharArray();
// Modify first element in array.
array[0] = Character. toUpperCase(array[0]);
// Return string.
return new String(array);
}
// CSV cleaner
public ArrayList CSVCleaner(ArrayList fileContent) {
ArrayList cleanerCon = new ArrayList();
String delimiter = ",";
StringBuilder newLine = null;
for (String str : fileContent) {
String[] fullLine = str. split(delimiter);
newLine = new StringBuilder();
for (int i = 0; i < fullLine. length - 1; i++) {
newLine. append(convert(fullLine[i]) + ",");
}
if (EmailValidator. isValidEmail(fullLine[fullLine. length - 1].toLowerCase())) {
newLine. append(fullLine[fullLine. length-1].toLowerCase());
} else {
newLine. append(convert(fullLine[fullLine. length - 1]));
}
cleanerCon. add(newLine. toString());
}
return cleanerCon;
}
}

Answers: 3
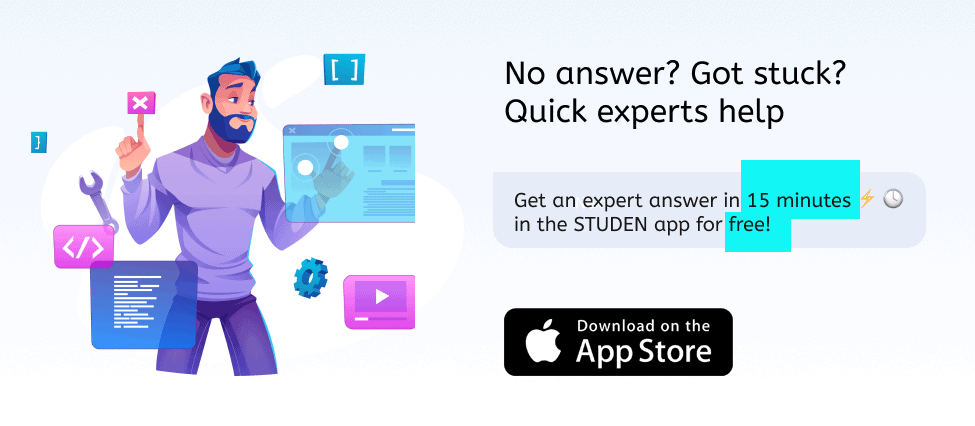
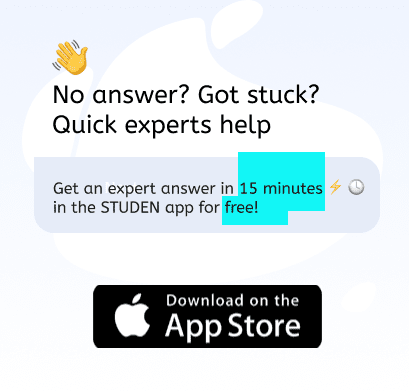
Another question on Computers and Technology

Computers and Technology, 22.06.2019 17:30
Where would you click to edit the chart data?
Answers: 1

Computers and Technology, 23.06.2019 02:50
Define a class named movie. include private fields for the title,year, and name of the director. include three public functions withprototypes void movie: : settitle(cstring); , voidmovie: : setyear(int); , void movie: : setdirector(string); . includeanother function that displays all the information about a movie.write a main() function that declares a movie object namedmyfavoritemovie. set and display the object's fields.this is what i have but know its wrong since it will notcompile: #include#includeusing namespace std; //class declarationclass movie{private: string movietitle ; string movieyear; string directorname; public: void settitle(string title); void setyear(string year); void setdirector(string director); void displayinfo(); }; //class implementationvoid movie: : settitle(string title){ movietitle = title; cout< < "what is the title of themovie? "< > temp; myfavoritemovie.settitle(temp); cout< < "enter movie year"< > temp; myfavoritemovie.setyear(temp); cout< < "enter director'sname"< > temp; myfavoritemovie.setdirector(temp); //display all the data myfavoritemovie.displayinfo(); system("pause"); return 0; this code is not entirely mine someone on cramster edited my firstcode but then i try manipulating the new code and i still get acompile error message : \documents\visual studio 2008\projects\movie\movie\movie.cpp(46) : error c2679: binary '< < ' : no operator found which takes aright-hand operand of type 'std: : string' (or there is no acceptableconversion)c: \program files (x86)\microsoft visual studio9.0\vc\include\ostream(653): could be'std: : basic_ostream< _elem,_traits> & std: : operator< < > (std: : basic_ostream< _elem,_traits> & ,const char *)w
Answers: 1

Computers and Technology, 23.06.2019 08:00
Michael has written an e-mail to his employees that describes a new product special that will be introduced to the customers next week. by taking time to make sure the e-mail is well written, logical, and organized, michael has made sure his message has the characteristics of a) effective communicationb) ineffective communicationc) barriers to communicationd) workplace communication
Answers: 2

Computers and Technology, 23.06.2019 12:00
If you embed a word table into powerpoint, what happens when you make edits to the embedded data? a. edits made to embedded data change the data in the source file; however, edits made to the source file will not be reflected in the embedded data. b. edits made to embedded data will change the data in the source file, and edits made to the source file will be reflected in the embedded data. c. edits made to embedded data don't change the data in the source file, nor will edits made to the source file be reflected in the embedded data. d. edits made to embedded data don't change the data in the source file; however, edits made to the source file will be reflected in the embedded data.
Answers: 1
You know the right answer?
Create an application that reads a file that contains an email list, reformats the data, and writes...
Questions

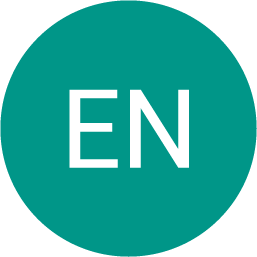



Mathematics, 27.07.2019 08:30


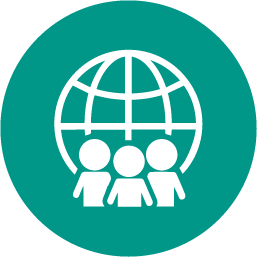
History, 27.07.2019 08:30
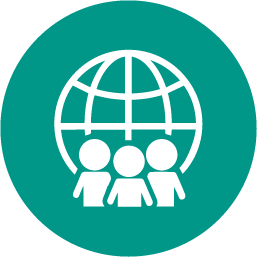
History, 27.07.2019 08:30
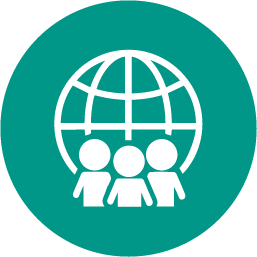


Mathematics, 27.07.2019 08:30

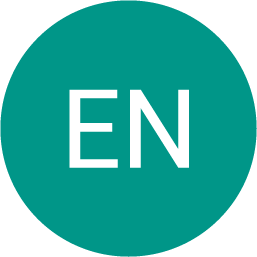
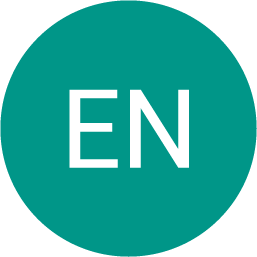


Chemistry, 27.07.2019 08:30
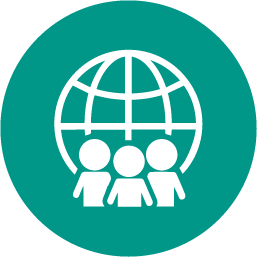

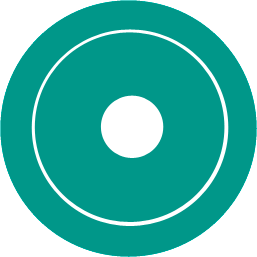
Advanced Placement (AP), 27.07.2019 08:30