
Computers and Technology, 29.07.2020 01:01 Kaiyla44
In this assignment, you are asked to implement a sorted list collection, called ThingSortedArrayBag where Thing is a class that represents an object of your choice.
Requirements
Implement your Thing class
Start by choosing what sort of list you would like to keep. For example: • To-do list. • Vacation and travel information • Pets • Real estate properties • pretty much any sort of list
You can be creative about your list. It is prohibited to use grocery items, circles, lines, persons, cars, students, bank accounts, points in two-dimensional space, or songs. Please note that you will reuse your Thing class across more than one programming assignment, so try to choose something that interests you. Include the name of your Thing class and its instance variables in your initial plan submission on February, 4th.
Your Thing class must have the following characteristics: • has a meaningful name (not Thing). • has exactly 2 instance variables such that: o one instance variable must be String and this variable will be used as a search key. o one instance variable must be int and this variable will be used to find aggregate information about Things that are stored in the collection class as will be explained later. • All instance variables must be declared as private.
• Implement a constructor for your Thing class that takes two input parameters to initialize the two instance variables. The order of the input parameters must be as follows String, then int. • Implement getters and setters for all the instance variables of your Thing. • Implement toString method that returns a String representation of your Thing class where all the instance variables are in one line and separated by tabs. • Implement the equals method for your Thing where two things are considered equal when they have the same values in both of the instance variables. Note that, the equality of String attributes should be case insensitive. For example, "MATH", "math" and "Math" match each other. In order to compare strings in Java use the String's equalsIgnoreCase method. For example, the following code prints true:
String str1 = "Hello"; String str2 = "hello"; System. out. println(str1.equalsIgnoreCase(str2) );
• Implement the comapreTo method that takes a Thing object as input parameter and returns either 0, -1, or 1 based on whether the input Thing is equal to, larger than, or smaller than the current thing respectively. Note that Things in your collection are to be sorted on the String search key first, then on the int instance variable. For example, if your Thing is a Circle that has color and radius as the String and int instance variables respectively, then: o (red,10) < (Red,20) because: red is equal to Red and 10 < 20. o (Blue, 10) < (red, 30) because Blue < red in alphabetical order ignoring the case.
The Thing class must also implement the Comparable interface. Assume, for example, your Thing is called Circle, then the class header will be as follows:
public class Circle implements Comparable

Answers: 1
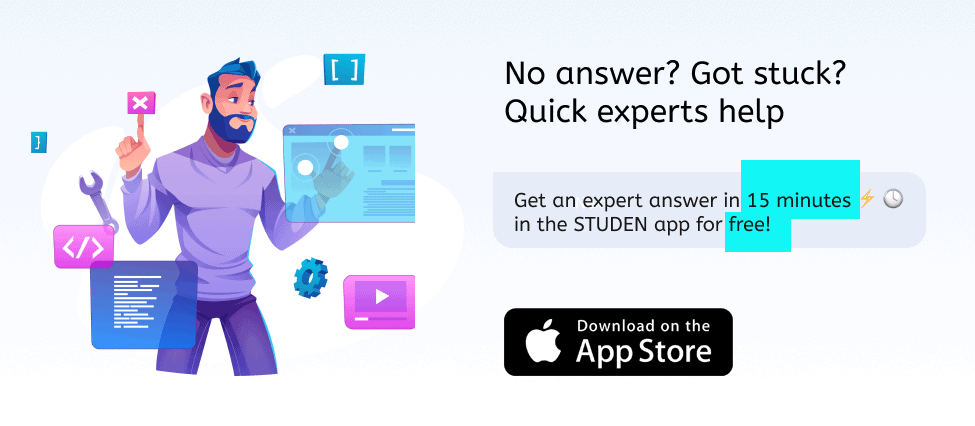
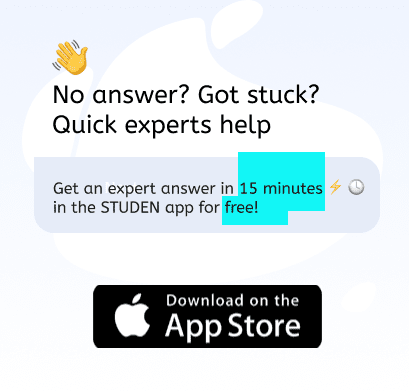
Another question on Computers and Technology

Computers and Technology, 22.06.2019 11:00
When building customer relationships through email what should you not do? question 2 options: utilize proper grammar, spelling, and punctuation type in all capital letters use hyperlinks rather than attachments respond to all emails within 24 hours
Answers: 1

Computers and Technology, 22.06.2019 20:40
Assume that there is a 4% rate of disk drive failure in a year. a. if all your computer data is stored on a hard disk drive with a copy stored on a second hard disk drive, what is the probability that during a year, you can avoid catastrophe with at least one working drive? b. if copies of all your computer data are stored on three independent hard disk drives, what is the probability that during a year, you can avoid catastrophe with at least one working drive?
Answers: 1

Computers and Technology, 23.06.2019 01:30
In deadlock avoidance using banker’s algorithm, what would be the consequence(s) of: (i) a process declaring its maximum need as maximum possible for each resource. in other words, if a resource a has 5 instances, then each process declares its maximum need as 5. (ii) a process declaring its minimum needs as maximum needs. for example, a process may need 2-5 instances of resource a. but it declares its maximum need as 2.
Answers: 3

Computers and Technology, 24.06.2019 23:00
What is a current gdp and what is a real gdp?
Answers: 1
You know the right answer?
In this assignment, you are asked to implement a sorted list collection, called ThingSortedArrayBag...
Questions



Mathematics, 08.12.2020 18:50
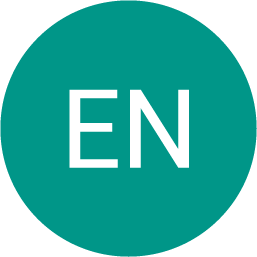
English, 08.12.2020 18:50

Chemistry, 08.12.2020 18:50

Mathematics, 08.12.2020 18:50
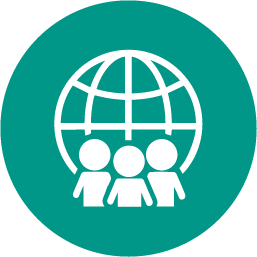
Social Studies, 08.12.2020 18:50

Mathematics, 08.12.2020 18:50
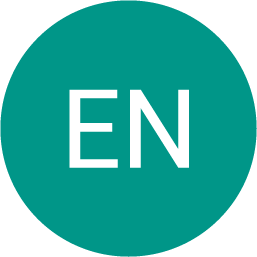

Mathematics, 08.12.2020 18:50
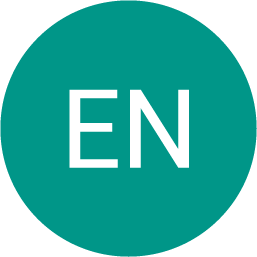
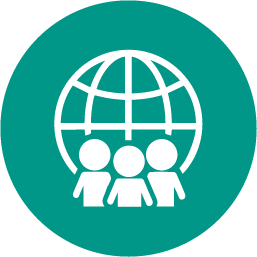
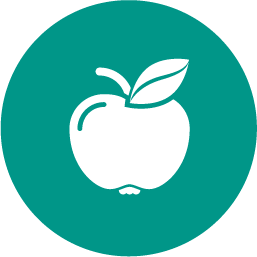
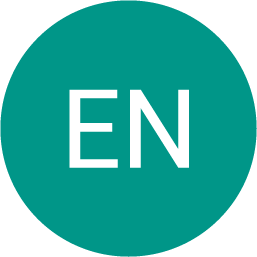
English, 08.12.2020 18:50


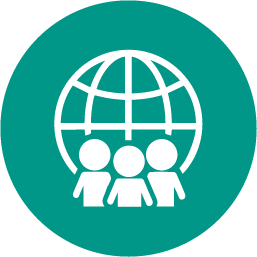

Mathematics, 08.12.2020 18:50


Mathematics, 08.12.2020 18:50