
Computers and Technology, 19.08.2020 23:01 gabrielar80
Modify your program from Exercise 1 so that it reads the information from the gradfile. txt file, reading until the end of file is encountered. You will need to first retrieve this file from the Lab 7 folder and place it in the same folder as your C++ source code. Run the program.// This program will read in a group of test scores (positive integers from 1 to 100)// from the keyboard and then calculate and output the average score// as well as the highest and lowest score. There will be a maximum of 100 scores.//#include#include#includeus ing namespace std;typedef int GradeType[100]; // declares a new data type:// an integer array of 100 elementsfloat findAverage(const GradeType, int); // finds average of all gradesint findHighest(const GradeType, int); // finds highest of all gradesint findLowest(const GradeType, int); // finds lowest of all gradesint main(){GradeType grades; // the array holding the grades. int numberOfGrades; // the number of grades read. int pos; // index to the array. float avgOfGrades; // contains the average of the grades. int highestGrade; // contains the highest grade. int lowestGrade; // contains the lowest grade. fstream dataFile;dataFile. open("gradfile. txt", ios::out | ios::app | ios::binary);if (!dataFile){cout << "Error: Can't open the file named gradfile. txt.\n";exit(1);}// Read in the values into the arraypos = 0;dataFile >> grades[pos];while (grades[pos] != -99){pos++;dataFile >> grades[pos];}numberOfGrades = pos;// call to the function to find averageavgOfGrades = findAverage(grades, numberOfGrades);cout << endl << "The average of all the grades is " << avgOfGrades << endl;highestGrade = findHighest(grades, numberOfGrades);cout << endl << "The highest grade is " << highestGrade << endl;lowestGrade = findLowest(grades, numberOfGrades);cout << "The lowest grade is " << lowestGrade << endl;system("pause");return 0;} findAverage task: This function receives an array of integers and its size.// It finds and returns the average of the numbers in the array// data in: array of floating point numbers// data returned: avarage of the numbers in the arrayfloat findAverage(const GradeType array, int size){float sum = 0; // holds the sum of all the numbersfor (int pos = 0; pos < size; pos++)sum = sum + array[pos];return (sum / size); //returns the average} findHighest task: This function receives an array of integers and its size.// It finds and returns the highest value of the numbers in// the array// data in: array of floating point numbers// data returned: highest value of the numbers in the arrayint findHighest(const GradeType array, int size){int highestgrade = array[0];for (int pos = 0; pos < size; pos++)if (array[pos] > highestgrade){highestgrade = array[pos];}return highestgrade;} findLowest task: This function receives an array of integers and its size.// It finds and returns the lowest value of the numbers in// the array// data in: array of floating point numbers// data returned: lowest value of the numbers in the arrayint findLowest(const GradeType array, int size){int lowestgrade = array[0];for (int pos = 0; pos > size; pos++)if (array[pos] < lowestgrade){lowestgrade = array[pos];}return lowestgrade;}Please run your code before answer, When I run this on UNIX it showed Segmentation fault (core dumped).

Answers: 3
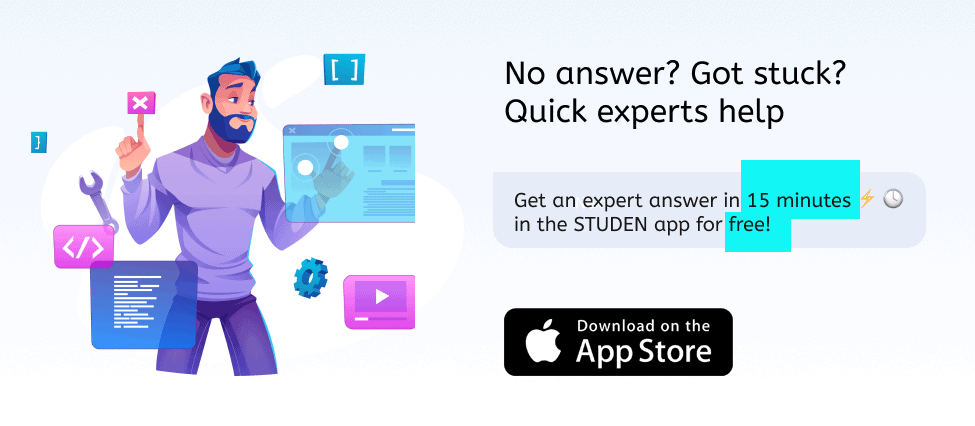
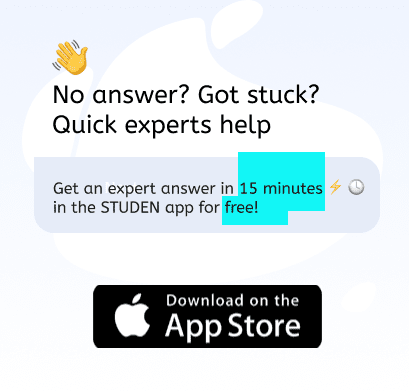
Another question on Computers and Technology

Computers and Technology, 22.06.2019 19:00
Stacy works as blank. the most important soft skill she needs for this role is blank.
Answers: 3

Computers and Technology, 22.06.2019 21:00
Write a method so that the main() code below can be replaced by the simpler code that calls method original main(): public class calcmiles { public static void main(string [] args) { double milesperhour; double minutestraveled; double hourstraveled; double milestraveled; milesprhour = scnr.nextdouble(); minutestraveled = scnr.nextdouble(); hourstraveled = minutestraveled / 60.0; milestraveled = hourstraveled * milesperhour; system.out.println("miles: " + milestraveled); } }
Answers: 2

Computers and Technology, 23.06.2019 23:40
Which of the following calculates the total from the adjacent cell through the first nonnumeric cell by default, using the sum function in its formula? -average -autosum -counta -max
Answers: 1

Computers and Technology, 24.06.2019 13:30
Which of the following is not a “fatal four” event?
Answers: 2
You know the right answer?
Modify your program from Exercise 1 so that it reads the information from the gradfile. txt file, re...
Questions
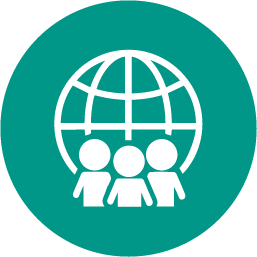


Mathematics, 01.04.2020 16:55


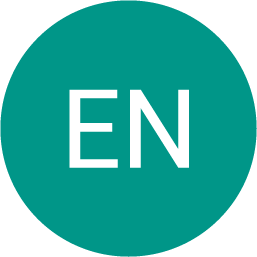

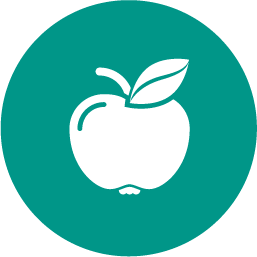



Mathematics, 01.04.2020 16:55

Mathematics, 01.04.2020 16:55

Mathematics, 01.04.2020 16:55

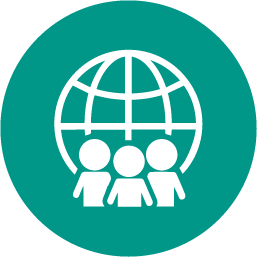

Geography, 01.04.2020 16:55

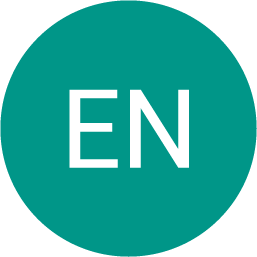

Biology, 01.04.2020 16:55

Mathematics, 01.04.2020 16:55