
Computers and Technology, 14.10.2020 01:01 levicorey846
Add the methods insert and pop to the Array class. These methods should use the strategies discussed in this chapter, including adjusting the length of the array, if necessary. The insert method expects a position and an item as arguments and inserts the item at the given position. If the position is greater than or equal to the arrayâs logical size, the method inserts the item after the last item currently available in the array. The pop method expects a position as an argument and removes and returns the item at that position. The pop methodâs precondition is 0 <= index < size(). The remove method should also reset the vacated array cell to the fill value. A main() has been provided to test the implementation of the insert and pop methods.
File: arrays. py
"""
class Array(object):
"""Represents an array."""
def __init__(self, capacity, fillValue = None):
"""Capacity is the static size of the array.
fillValue is placed at each position."""
self. items = list()
self. logicalSize = 0
# Track the capacity and fill value for adjustments later
self. capacity = capacity
self. fillValue = fillValue
for count in range(capacity):
self. items. append(fillValue)
def __len__(self):
"""-> The capacity of the array."""
return len(self. items)
def __str__(self):
"""-> The string representation of the array."""
return str(self. items)
def __iter__(self):
"""Supports traversal with a for loop."""
return iter(self. items)
def __getitem__(self, index):
"""Subscript operator for access at index.
Precondition: 0 <= index < size()"""
if index < 0 or index >= self. size():
raise IndexError("Array index out of bounds")
return self. items[index]
def __setitem__(self, index, newItem):
"""Subscript operator for replacement at index.
Precondition: 0 <= index < size()"""
if index < 0 or index >= self. size():
raise IndexError("Array index out of bounds")
self. items[index] = newItem
def size(self):
"""-> The number of items in the array."""
return self. logicalSize
def grow(self):
"""Increases the physical size of the array if necessary."""
# Double the physical size if no more room for items
# and add the fillValue to the new cells in the underlying list
for count in range(len(self)):
self. items. append(self. fillValue)
def shrink(self):
"""Decreases the physical size of the array if necessary."""
# Shrink the size by half but not below the default capacity
# and remove those garbage cells from the underlying list
newSize = max(self. capacity, len(self) // 2)
for count in range(len(self) - newSize):
self. items. pop()

Answers: 2
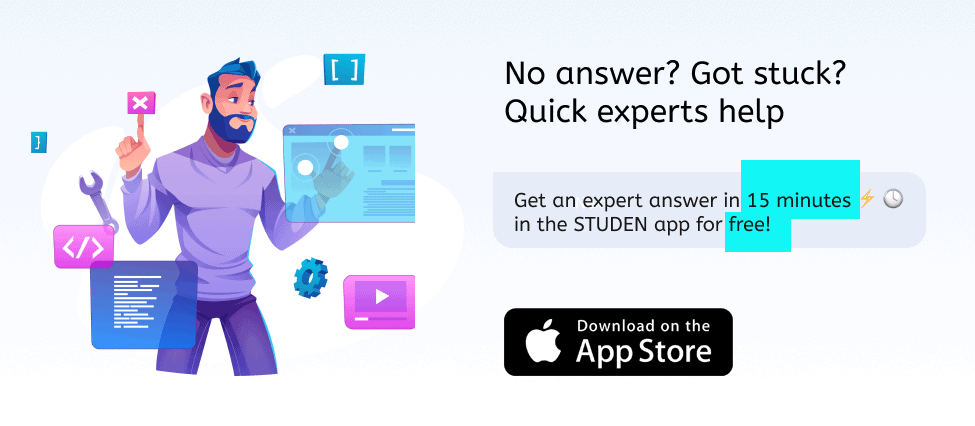
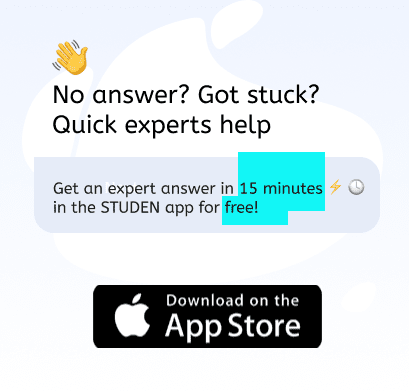
Another question on Computers and Technology

Computers and Technology, 23.06.2019 04:00
Write a method that takes in an array of point2d objects, and then analyzes the dataset to find points that are close together. be sure to review the point2d api. in your method, if the distance between any pair of points is less than 10, display the distance and the (x,y)s of each point. for example, "the distance between (3,5) and (8,9) is 6.40312." the complete api for the point2d adt may be viewed at ~pf/sedgewick-wayne/algs4/documentation/point2d.html (links to an external site.)links to an external site.. try to write your program directly from the api - do not review the adt's source code.
Answers: 1

Computers and Technology, 24.06.2019 20:30
Drums installed, you would apply clicks of the parking brake to obtain a slight drag on both rear wheels
Answers: 1

Computers and Technology, 25.06.2019 01:00
Your computer will organize files into order. alphabetical chronological size no specific
Answers: 2

Computers and Technology, 25.06.2019 04:30
Which relativos possible when two tables share the same primary key? a.) one-to-one b.) one-to-many c.) many-to-one d.) many-to-many
Answers: 2
You know the right answer?
Add the methods insert and pop to the Array class. These methods should use the strategies discussed...
Questions

Mathematics, 13.11.2019 18:31

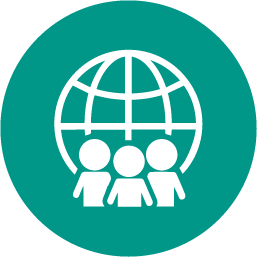




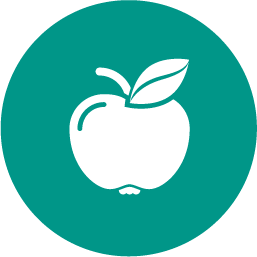
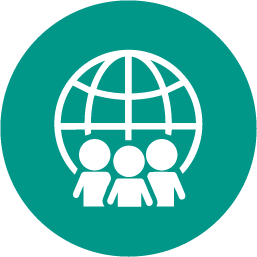
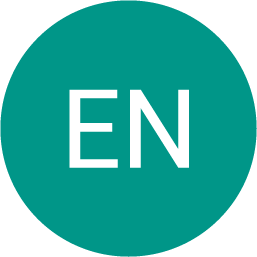

Mathematics, 13.11.2019 18:31

Biology, 13.11.2019 18:31

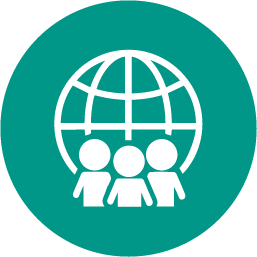
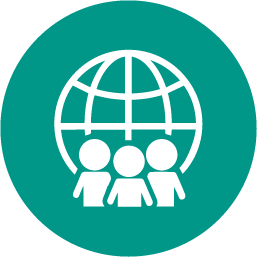
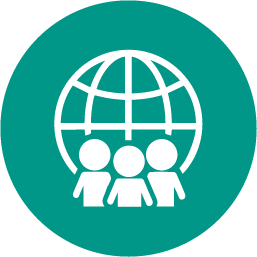

Mathematics, 13.11.2019 18:31

Chemistry, 13.11.2019 18:31
