
Computers and Technology, 19.10.2020 19:01 alandrabell9234
In this session, you will set up your account to implement necessary linked list functions for FIFO CPU scheduling and perform the very basic steps in context-switching. In the next session, you will extend this program to implement other three basic CPU scheduling algorithms (namely, SJF, PR, and RR with a given quantum). To keep it simle, we assume each process has a single CPU burst without any I/O burst and that all processes arrive at the same time in a given order. The order of processes will be given in a simple input file. Each line in the input file consists of three integer numbers: Process Id, Process Priority, CPU Burst time (ms). Download inputl. txt as a sample input file.
Your program will take the name of the scheduling algorithm, related parameters (if any), and an input file name from command line. In general, here how your program should be executed:
prog -alg [FIFOlSJF IPRIRR] [-quantum [integer(ms)]1 -input [input_file_name. txt
In this session, you will just run it as:
> prog -alg FIFo -input input1.txt
For the given input file, the output of your program will be as follows:
Student Name: Your name
Input File Name: input1.txt
CPU Scheduling Alg FIFO
Proces 1 is completed at 5 ms
Proces 2 is completed at 12 ms
Proces 3 is completed at 18 ms
Proces 4 is completed at 22 ms
Average waiting time = 8.75 ms (35/4)
Average Turnaround time-14.25 ms (57/4)
Throughput -e.18 jobs per ms (4/22)
Detailed steps for this session:
Define a very basic PCB structure: struct PCB {int ProcId; int ProcPR, int CPUburst; int Reg[8]; int queueEnterClock, waitingTime; /* other info */ struct PCB *next;}
Define a very basic CPU consisting of 8 integer registers: int CPUreg[8] = {0};
Initialize your linked list: struct PCB *Head=NULL; struct PCB *Tail=NULL;
Initialize your other data: int CLOCK=0; int Total_waiting_time=0; int Total_turnaround_time=0; int Total_job=0;
Open the input file
For each input line,
read a line consisting of three integers: Process Id, Process Priority, CPU Burst time.
dynamically create a struct PCB pointed by PCB,
save the given data into correponding fields of PCB,
set all PCB->Reg[8] to the Process ID, set PCB->queueEnterClock and PCB->waitingTime to 0, then
insert this PCB at the end of the link list.
Close input file
Print your name, the name of scheduling algorithm, and the input file name, as mentioned before.
The above steps will be the same for the next session too
Now implement a FIFO_Scheduling() function and call it to print the order of completed processes.
This function simply removes the PCB from the head of the linked list and performs the followings until the linked list is empty:
Do context-switching:
copy PCB->Reg[8] into CPUreg[8],
suppose some work is done on CPU (e. g, increment each CPUreg by one),
copy CPUreg[8] into PCB->Reg[8]
Data collection for performance metrics
PCB->waitingTime = PCB->waitingTime + CLOCK - PCB->queueEnterClock;
Total_waiting_time = Total_waiting_time + PCB->waitingTime ;
CLOCK = CLOCK + PCB->CPUburst;
Total_turnaround_time = Total_turnaround_time + CLOCK;
Total_job = Total_job + 1;
Free PCB. Since there is no more CPUburst or I/O burst, this process is terminated here! Otherwise, it will be put in a ready or waiting queue.
Finally, print the perfromance metrics mentioned before:
Total_waiting_time / Total_job,
Total_turnaround_time / Total_job,
Total_job / CLOCK
For each input file, copy/paste your screen output into a textfile (say output1.txt)
input1.txt:
1 3 5
2 3 7
3 1 6
4 5 4

Answers: 3
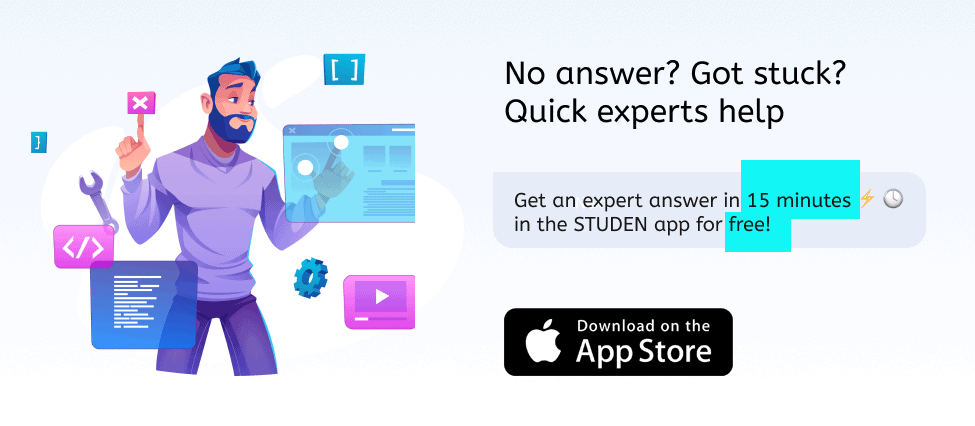
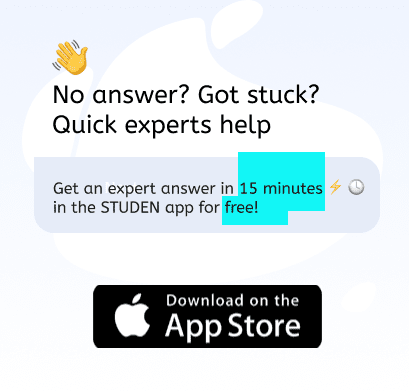
Another question on Computers and Technology

Computers and Technology, 22.06.2019 08:10
Ihave a music player on my phone. i can buy songs, add them to playlists and play them. obviously it would be redundant to store each song in each playlist; each playlist is just a list of pointers to the songs. for this lab you will simulate this behavior. your program will need to have options to: add songs to the system library (you will store the text of the first line of the song, rather than the audio) add playlists add songs to a playlist list playlists play a playlist list all of the songs in the library with a count of how many times each song has been played remove a song from a playlist remove a playlist remove a song from the library (and thus from all playlists that contain it) note that we will not be checking many error cases. in real programming this would be bad, you should usually try to recognize and respond to as many types of errors as you can. in the context of class we are trying to acquaint you with as many concepts as possible, so for the sake of educational efficiency we will not be checking most errors in this lab, you may assume that your user provides correct input. you may add all appropriate error testing if you wish, but we will not be testing for it.
Answers: 2

Computers and Technology, 24.06.2019 00:30
Setting up a home network using wireless connections is creating a a. vpn b. lan c. wan d. mini-internet
Answers: 2

Computers and Technology, 24.06.2019 15:40
In the above figure, what type of cylinder arrangement is shown in the figure above? a. l-type b. v-type c. in-line d. horizontal pls make sure its right if its rong im grounded for 3months
Answers: 1

Computers and Technology, 24.06.2019 23:30
Does anyone have the problem where you try to watch a video to get your answer but it brings up a thing asking your gender to make ads relevant but it doesn't load? btw i won't be able to see the answer so use the comments .
Answers: 1
You know the right answer?
In this session, you will set up your account to implement necessary linked list functions for FIFO...
Questions

Computers and Technology, 12.01.2021 21:10

Mathematics, 12.01.2021 21:10


Mathematics, 12.01.2021 21:10
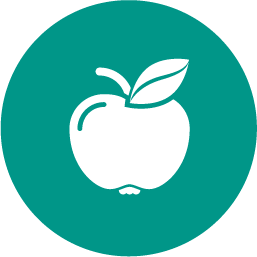
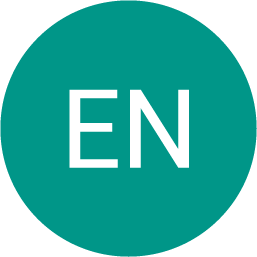
English, 12.01.2021 21:10

Engineering, 12.01.2021 21:10
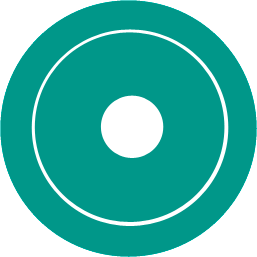
Advanced Placement (AP), 12.01.2021 21:10
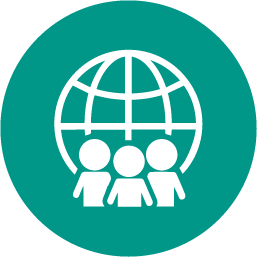

Chemistry, 12.01.2021 21:10

Mathematics, 12.01.2021 21:10

Mathematics, 12.01.2021 21:10

Law, 12.01.2021 21:10

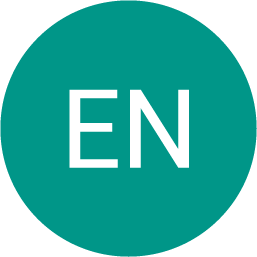
English, 12.01.2021 21:10



