Language: C
Introduction
For this assignment you will write an encoder and a decoder for a mo...

Computers and Technology, 19.10.2020 22:01 dorothybean
Language: C
Introduction
For this assignment you will write an encoder and a decoder for a modified "book cipher." A book cipher uses a document or book as the cipher key, and the cipher itself uses numbers that reference the words within the text. For example, one of the Beale ciphers used an edition of The Declaration of Independence as the cipher key. The cipher you will write will use a pair of numbers corresponding to each letter in the text. The first number denotes the position of a word in the key text (starting at 0), and the second number denotes the position of the letter in the word (also starting at 0). For instance, given the following key text (the numbers correspond to the index of the first word in the line):
[0] 'Twas brillig, and the slithy toves Did gyre and gimble in the wabe;
[13] All mimsy were the borogoves, And the mome raths outgrabe.
[23] "Beware the Jabberwock, my son! The jaws that bite, the claws that catch!
[36] Beware the Jubjub bird, and shun The frumious Bandersnatch!"
[45] He took his vorpal sword in hand: Long time the manxome foe he sought—
The word "computer" can be encoded with the following pairs of numbers:
35,0 catch
5,1 toves
42,3 frumious
48,3 vorpal
22,1 outgrabe
34,3 that
23,5 Beware
7,2 gyre
Placing these pairs into a cipher text, we get the following:
35,0,5,1,42,3,48,3,22,1,34,3,23,5,7 ,2
If you are encoding a phrase, rather than just a single word, spaces in the original english phrase will also appear in the ciphered text. So, the phrase "all done" (using the above Jabberwocky poem) might appear as:
0,3,1,4,13,1 6,0,46,2,44,2,3,2
Only spaces in the key text should be considered delimiters. All other punctuation in the key text are to be considered part of a key word. Thus the first word in the Jabberwocky poem, 'Twas, will have the following characters and positions for key word 0:
Position 0: '
Position 1: T
Position 2: w
Position 3: a
Position 4: s
Developing the Program
You should approach this assignment in several parts. The first part will be to write a menu driven program that prompts the user for the following actions:
1) Read in the name of a text file to use as a cipher key
2) Create a cipher using the input text file (and save the result to a file)
3) Decode an existing cipher (prompt user for a file to read containing the cipher text)
4) Exit the program
Additional Specifications
In order to introduce some "randomness" in the specific character encoding, you will generate a random number i from 0..9 inclusive (use the last four digits of your G Number as the seed), and use the ith instance of that character found in the text. (If fewer than i instances of the character is found in the text, loop back and continue the search from the beginning of the document.)
Example: Suppose the letter to encode is a 'c'. Using the sentences just above, we find that there are the following 'c' characters:
In order to introduCe some "randomness" in the speCifiC CharaCter enCoding, you will generate a random number i from 0..9 inClusive (use the last four digits of your G Number as the seed), and use the ith instanCe of that CharaCter found in the text.
If the random number returns 6, then you will use the 'c' from the word "inclusive." (Start counting from 0). If the random number returns 2, you would the second c found in the word "specific."
If a given character in the secret message is not found in any word of the text, replace that character with the '#' character in the encoded text (a single '#' character replaces a word/position pair).
Files and filenames will follow the standard CS262 conventions (username, lab section, etc. at top of file, and as part of filename).
Each menu choice (except #4) should call a separate function to perform the operation.
You can assume that the message to encrypt or decrypt will be less than 1500 characters.
Your program will be compiled using a Makefile
Predefined C functions that may be useful
strtok()
strlen()
tolower()
atoi()

Answers: 3
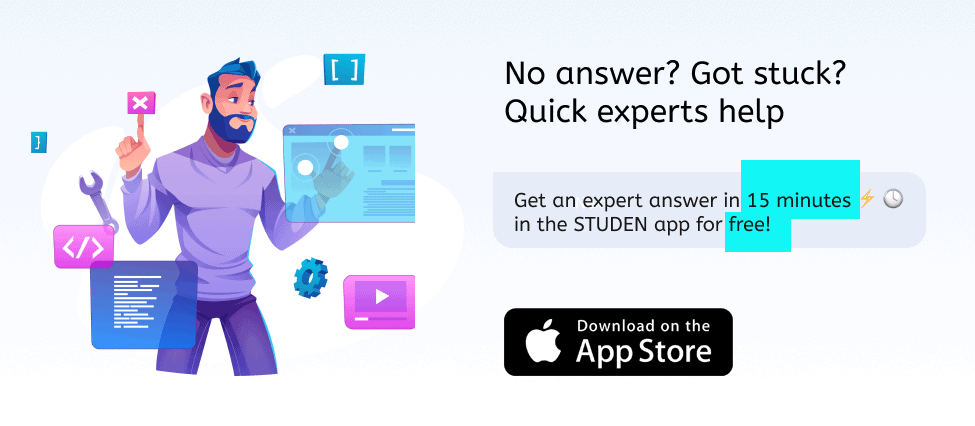
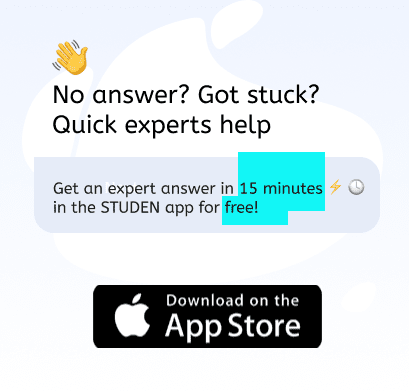
Another question on Computers and Technology

Computers and Technology, 22.06.2019 01:30
What “old fashioned” features of checking accounts is p2p replacing
Answers: 3

Computers and Technology, 22.06.2019 10:30
You have a large, late-model pick-up truck with a rear seat. the pick-up truck weighs 6,500 pounds. the florida seat belt law
Answers: 1

Computers and Technology, 22.06.2019 20:10
Assume that minutes is an int variable whose value is 0 or positive. write an expression whose value is "undercooked" or "soft-boiled" or "medium-boiled" or "hard-boiled" or "overcooked" based on the value of minutes. in particular: if the value of minutes is less than 2 the expression's value is "undercooked"; 2-4 would be a "soft-boiled", 5-7 would be "medium-boiled", 8-11 would be "hard-boiled" and 12 or more would be a "overcooked".
Answers: 1

Computers and Technology, 23.06.2019 19:30
2. fluorine and chlorine molecules are blamed fora trapping the sun's energyob forming acid rainoc producing smogod destroying ozone molecules
Answers: 2
You know the right answer?
Questions

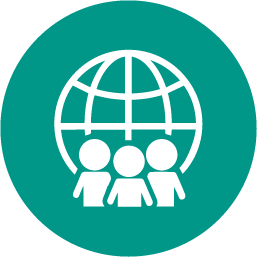
History, 20.08.2020 22:01



Mathematics, 20.08.2020 22:01

Mathematics, 20.08.2020 22:01

Mathematics, 20.08.2020 22:01

Mathematics, 20.08.2020 22:01


Mathematics, 20.08.2020 22:01

Mathematics, 20.08.2020 22:01

Mathematics, 20.08.2020 22:01
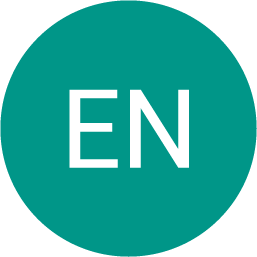
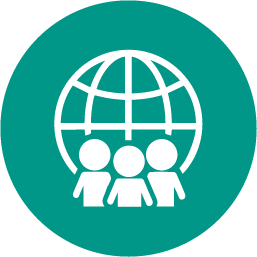
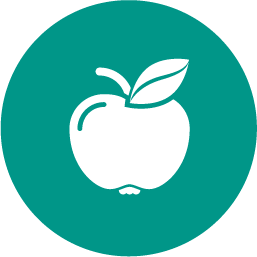

Mathematics, 20.08.2020 22:01

Mathematics, 20.08.2020 22:01


Mathematics, 20.08.2020 22:01

Mathematics, 20.08.2020 22:01