
Computers and Technology, 20.10.2020 21:01 tynyiaawrightt
This assignment requires you to write a program to analyze a web page HTML file. Your program will read one character at a time from the file specifying the web page, count various attributes in the code for the page, and print a report to the console window giving information about the coding of the page.
Note that a search engine like Google uses at least one technique along similar lines. To calculate how popular (frequently visited or cited) a web page is, one technique is to look at as many other worldwide web pages as possible which are relevant, and count how many links the world's web pages contain back to the target page.
Learning Objectives
To utilize looping structures for the first time in a C++ program
To develop more sophisticated control structures using a combination of selection and looping
To read data from an input file
To gain more experience using manipulators to format output in C++
To consider examples of very simple hypertext markup language (HTML)
Use UNIX commands to obtain the text data files and be able to read from them
Problem Statement
Your program will analyze a few things about the way in which a web page is encoded. The program will ask the user for the name of a file containing a web page description. This input file must be stored in the correct folder with your program files, as discussed in class, and it must be encoded using hypertext markup language, or HTML. The analysis requires that you find the values of the following items for the page:
number of lines (every line ends with the EOLN marker; there will be an EOLN in front of the EOF)
number of tags (includes links and comments)
number of links
number of comments in the file
number of characters in the file (note that blanks and EOLNs do count)
number of characters inside tags
percentage of characters which occur inside tags, out of the total
Here is an example of a very simple HTML file:
Course Web Page
This course is about programming in C++.
Click here
You may assume that the HTML files you must analyze use a very limited subset of basic HTML, which includes only the following "special" items:
tag always starts with '<' and ends with >
link always starts with "
comment always starts with ""
You may assume that a less-than symbol (<) ALWAYS indicates the start of a tag. A tag in HTML denotes an item that is not displayed on the web page, and often gives instructions to the web browser. For example, indicates that the next item is the overall title of the document, and indicates the end of that section. In tags, both upper and lowercase letters can be used.
Note on links and comments: to identify a link, you may just look for an 'a' or 'A' which occurs just after a '<'. To identify a comment, you may just look for a '!' which follows just after a '' (that is, you do not have to check for the two hyphens).
You may assume that these are the only HTML language elements you need to recognize, and that the HTML files you process contain absolutely no HTML syntax errors.
Note: it is both good style because readability is increased, and convenient, to declare named constants for characters that are meaningful, for example
const char TAG OPEN = '
Sample Input Files
Program input is to be read from a text file. Your program must ask the user for interactive input of the file name. You can declare a variable to store the file name and read the user's response
Miscellaneous Tips and Information
You should not read the file more than once to perform the analysis. Reading the file more than once is very inefficient.
The simplest, most reliable and consistent way to check for an end of file condition on a loop is by checking for the fail state, as shown in lectures. The eof function is not as reliable or consistent and is simply deemed "flaky" by many programmers as it can behave inconsistently for different input files and on different platforms.
You may use only while loops on this project if you wish; you are not required to choose amongst while, do while and/or for loops until project 4 and all projects thereafter. Do not create any functions other than main() for this program. Do not use data structures such as arrays. You may only have ONE return statement and NO exit statements in your code. You may only use break statements inside a switch statement in the manner demonstrated in class; do not use break or continue statements inside loops.
#include // used for interactive console I/O
#include // used to format output
#include // used to retrieve data from file
#include // used to convert data to uppercase to simplify comparisons
#include 11 for string class functions
int main() { 1
//constants for analysing HTML attributes
const char EOLN = '\n';
const char COMMENT_MARK = '!';
const char LINK='A';
//constants to format the output
const int PAGEWIDTH = 70;
const char UNDERLINE = '=';
const char SPACE =
const int PCT_WIDTH = 5;
const int PCT_PRECISION = 2;

Answers: 2
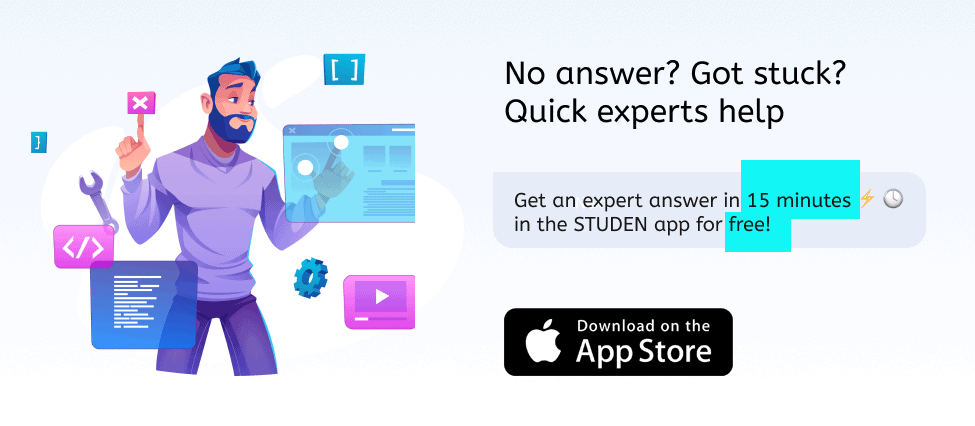
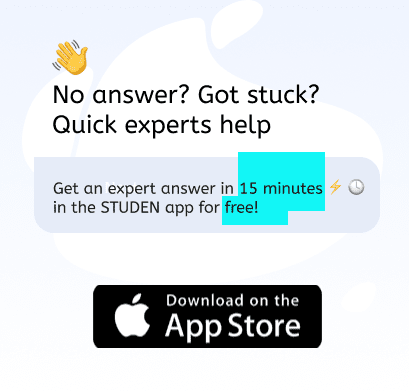
Another question on Computers and Technology

Computers and Technology, 22.06.2019 11:00
The isometric projection camera technique provides an illusion of perspective by using things like parallax scrolling to create the illusion of 3d in a 2d game
Answers: 3

Computers and Technology, 22.06.2019 19:00
If your accelerator suddenly gets stuck what should you do
Answers: 2

Computers and Technology, 22.06.2019 22:00
Consider the following declarations (1, 2, 3, 5, 7)class bagtype{public: void set(string, double, double, double, double); void print() const; string getstyle() const; double getprice() const; void get(double, double, double, double); bagtype(); bagtype(string, double, double, double, double); private: string style: double l; double w; double h; double price; }; a.) write the definition of the number function set so that private members are set according to the parametersb.) write the definition of the member function print that prints the values of the data membersc.) write the definition of the default constructor of the class bagtype so that the private member variables are initialized to "", 0.0, 0.0, 0.0, 0.0, respectively d.) write a c++ statement that prints the value of the object newbag.e.) write a c++ statement that declares the object tempbag of type bagtype, and initialize the member variables of tempbag to "backpack", 15, 8, 20 and 49.99, respectively
Answers: 3

Computers and Technology, 22.06.2019 22:40
In this lab, you complete a python program that calculates an employee's annual bonus. input is an employee's first name, last name, salary, and numeric performance rating. if the rating is 1, 2, or 3, the bonus rate used is .25, .15, or .1 respectively. if the rating is 4 or higher, the rate is 0. the employee bonus is calculated by multiplying the bonus rate by the annual salary.
Answers: 1
You know the right answer?
This assignment requires you to write a program to analyze a web page HTML file. Your program will r...
Questions

Mathematics, 06.07.2019 00:00
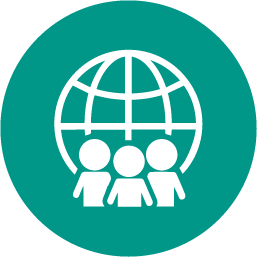
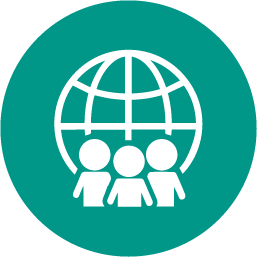
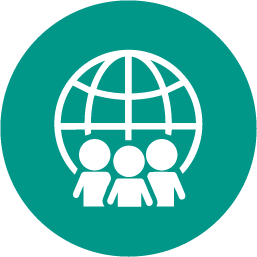
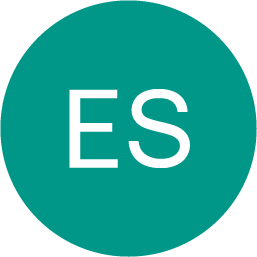
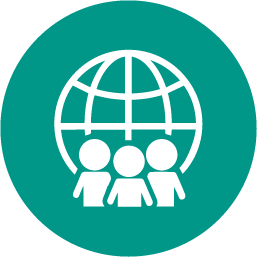
History, 06.07.2019 00:00


Chemistry, 06.07.2019 00:00


Mathematics, 06.07.2019 00:00
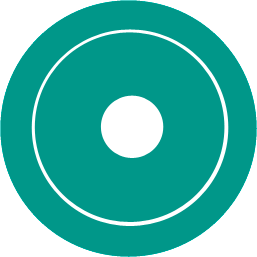
Advanced Placement (AP), 06.07.2019 00:00
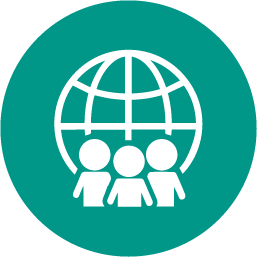
History, 06.07.2019 00:00
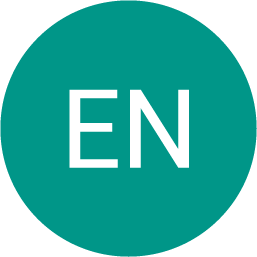

Mathematics, 06.07.2019 00:00

Computers and Technology, 06.07.2019 00:00
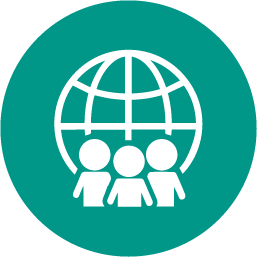
History, 06.07.2019 00:00

Mathematics, 06.07.2019 00:00
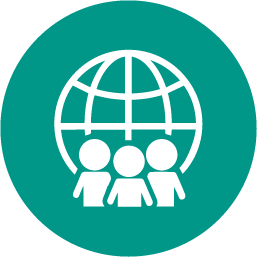


Computers and Technology, 06.07.2019 00:00