
Computers and Technology, 20.10.2020 21:01 KitKatKrunchy
You've created a new programming language, and now you've decided to add hashmap support to it. Actually you are quite disappointed that in common programming languages it's impossible to add a number to all hashmap keys, or all its values. So you've decided to take matters into your own hands and implement your own hashmap in your new language that has the following operations:
insert x y - insert an object with key x and value y.
get x - return the value of an object with key x.
addToKey x - add x to all keys in map.
addToValue y - add y to all values in map.
To test out your new hashmap, you have a list of queries in the form of two arrays: queryTypes contains the names of the methods to be called (eg: insert, get, etc), and queries contains the arguments for those methods (the x and y values).
Your task is to implement this hashmap, apply the given queries, and to find the sum of all the results for get operations.
Example
For queryType = ["insert", "insert", "addToValue", "addToKey", "get"] and query = [[1, 2], [2, 3], [2], [1], [3]], the output should be hashMap(queryType, query) = 5.
The hashmap looks like this after each query:
1 query: {1: 2}
2 query: {1: 2, 2: 3}
3 query: {1: 4, 2: 5}
4 query: {2: 4, 3: 5}
5 query: answer is 5
The result of the last get query for 3 is 5 in the resulting hashmap.
For queryType = ["insert", "addToValue", "get", "insert", "addToKey", "addToValue", "get"] and query = [[1, 2], [2], [1], [2, 3], [1], [-1], [3]], the output should be hashMap(queryType, query) = 6.
The hashmap looks like this after each query:
1 query: {1: 2}
2 query: {1: 4}
3 query: answer is 4
4 query: {1: 4, 2: 3}
5 query: {2: 4, 3: 3}
6 query: {2: 3, 3: 2}
7 query: answer is 2
The sum of the results for all the get queries is equal to 4 + 2 = 6.
Input/Output
[execution time limit] 4 seconds (py3)
[input] array. string queryType
Array of query types. It is guaranteed that each queryType[i] is either "addToKey", "addToValue", "get", or "insert".
Guaranteed constraints:
1 ≤ queryType. length ≤ 105.
[input] array. array. integer query
Array of queries, where each query is represented either by two numbers for insert query or by one number for other queries. It is guaranteed that during all queries all keys and values are in the range [-109, 109].
Guaranteed constraints:
query. length = queryType. length,
1 ≤ query[i].length ≤ 2.
[output] integer64
The sum of the results for all get queries.
[Python3] Syntax Tips
# Prints help message to the console
# Returns a string
def helloWorld(name):
print("This prints to the console when you Run Tests")
return "Hello, " + name

Answers: 3
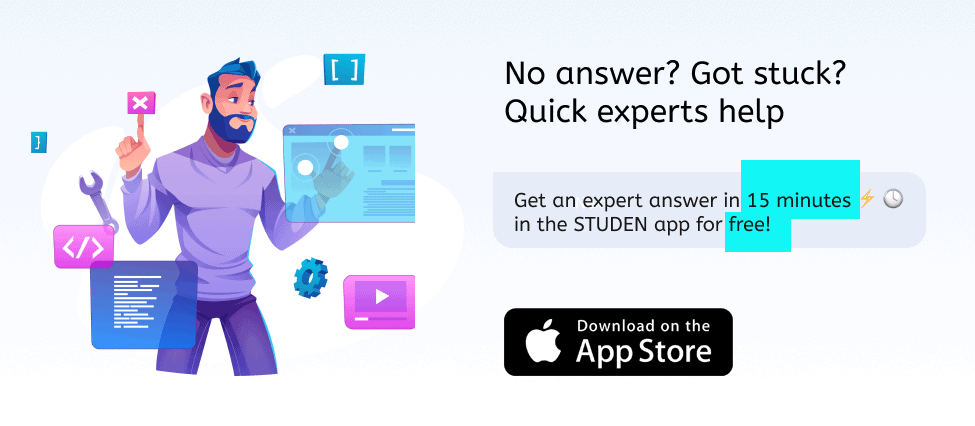
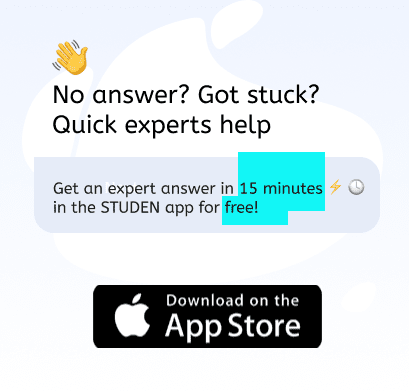
Another question on Computers and Technology

Computers and Technology, 22.06.2019 00:30
Which of the following methods could be considered a “best practice” in terms of informing respondents how their answers to an on-line survey about personal information will be protected? respondents are informed that investigators will try to keep their participation confidential; however, confidentiality cannot be assured. respondents are informed that a research assistant will transfer all the research data to a password-protected computer that is not connected to the internet, via a usb flashdrive. the computer is located in a research team member’s office. the investigator uses the informed consent process to explain her institution’s method for guaranteeing absolute confidentiality of research data. the investigator uses the informed consent process to explain how respondent data will be transmitted from the website to his encrypted database without ever recording respondents’ ip addresses, but explains that on the internet confidentiality cannot be absolutely guaranteed.
Answers: 1

Computers and Technology, 22.06.2019 10:40
5. illustrate how fine-line inventory classification can be used with product and market segments. what are the benefits and considerations when classifying inventory by product, market, and product/market?
Answers: 2

Computers and Technology, 23.06.2019 09:30
You wanted to look up information about alzheimer's, but you were unsure if it was spelled "alsheimer's" or "alzheimer's." which advanced search strategy would be useful? a) a boolean search b) using a wild card in your search c) trying different search engines d) doing a search for "alsheimer's not alzheimer's" asap. ill give brainlist.
Answers: 1

Computers and Technology, 24.06.2019 02:00
Which steps will open the system so that you can enter a question and do a search for
Answers: 1
You know the right answer?
You've created a new programming language, and now you've decided to add hashmap support to it. Actu...
Questions
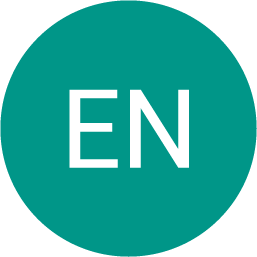

Mathematics, 28.10.2019 20:31

Arts, 28.10.2019 20:31


Geography, 28.10.2019 20:31

Mathematics, 28.10.2019 20:31

Mathematics, 28.10.2019 20:31
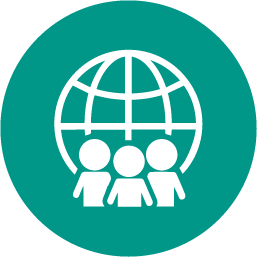
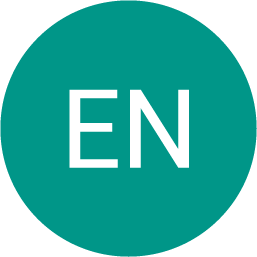
English, 28.10.2019 20:31

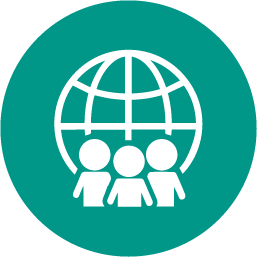

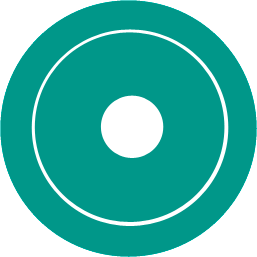
Advanced Placement (AP), 28.10.2019 20:31
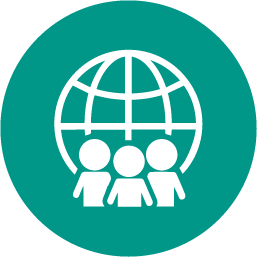
History, 28.10.2019 20:31

Biology, 28.10.2019 20:31


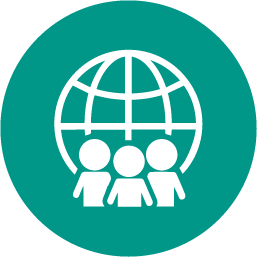
History, 28.10.2019 20:31
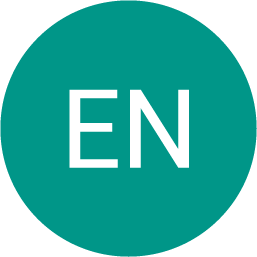
English, 28.10.2019 20:31