
Computers and Technology, 29.10.2020 07:20 jaueuxsn
C++ Write a program that first asks the user which Temperature scale conversion he/she would like to perform:
1. Convert F to C
2. Convert C to F
3. Quit
What is your choice?
Then it asks the user for input for three real number variables: start_temp, end_temp, temp_incr. It will then produce a two column Fahrenheit to Celsius table or a two column Celsius to Fahrenheit table, depending on the choice.
For choice 1, the first column should be labeled Fahrenheit and the first value the Fahrenheit column is start_temp. The second column should be labeled Celsius, and its value is calculated from the values in the Fahrenheit column using the formula
C = (5.0/9.0)*(F β 32.0).
For choice 2, the table will show the Celsius column first, Fahrenheit column second, and use the formula
F = 9.0/5.0 * C + 32.0
The values for the temps in the first column will be incremented by temp_incr, and end when the table value would exceed the end_temp value. Display all values with 2 decimal of accuracy, justified and aligned.
Requirements:
1) A detailed algorithm
2) Verify all numeric data are acceptable and not character
3) Follow all good programming practices such as descriptive variable names, constants names, proper indentation,...
4) Verify input for correct range and type ( not character)
5) Output should be the same as the sample shown below, I used 30 βββ for the underlining of heading.
Sample Output
Output Sample:
Choose a conversion type:
1. Convert F to C
2. Convert C to F
3. Quit
What is your choice? 1
Enter starting value: 29
Enter ending value: 32
Enter increment value: 0.5
Fahrenheit Celsius
29.00 -1.67
29.50 -1.39
30.00 -1.11
30.50 -0.83
31.00 -0.56
31.50 -0.28
32.00 0.00
Choose a conversion type:
1. Convert F to C
2. Convert C to F
3. Quit
What is your choice? 4
Invalid choice. Try again.
Choose a conversion type:
1. Convert F to C
2. Convert C to F
3. Quit
What is your choice? 2
Enter starting value: 35
Enter ending value: 32
Enter increment value: -1
Celsius Fahrenheit
35.00 95.00
34.00 93.20
33.00 91.40
32.00 89.60
Choose a conversion type:
1. Convert F to C
2. Convert C to F
3. Quit
What is your choice? 2
Enter starting value (integer number): 35
Enter ending value (integer number): 32
Enter increment value (real number): 1
Invalid range. Try again.
Choose a conversion type:
1. Convert F to C
2. Convert C to F
3. Quit
What is your choice? 3
Thank you for your using my program. Program terminated.
THIS IS MY CODE:
#include
#include
using namespace std;
const int CWIDTH = 26;
int main() {
int choice;
double convertFoC, converCtoF;
double starting, endvalue, incrementvalue;
const int CWIDTH = 13;
cin >> choice;
switch (choice)
{
case 4:
cout > starting;
if (starting == 28){
cout > endvalue;
cin >> incrementvalue;
switch (choice) {
case 1:
cout << "Fahrenheit" << setw(17) << "Celsius" << endl;
for (double calcCelsius = starting; calcCelsius <= endvalue; calcCelsius = calcCelsius + incrementvalue)
{
convertFoC = (5.0 / 9.0) * (calcCelsius - 32.0);
cout << showpoint << fixed << setprecision(2) << calcCelsius << setprecision(2) << setw(21) << convertFoC << right;
cout << endl;
}
break;
case 2:
cout << "Celsius" << setw(CWIDTH) << "Fahrenheit\n";
for (double calcFahrenheit = starting; calcFahrenheit <= endvalue; calcFahrenheit = calcFahrenheit + incrementvalue)
{
converCtoF = ((9.0 / 5.0) * calcFahrenheit) + 32.0;
cout << setw(CWIDTH) << showpoint << fixed << setprecision(1) << calcFahrenheit << fixed << setprecision(1) << setw(CWIDTH) << converCtoF << right;
cout << endl;
}
}
}
This is my code but i have a lot of test that i can't get into it.
like the screenshoots that i post here.

Answers: 3
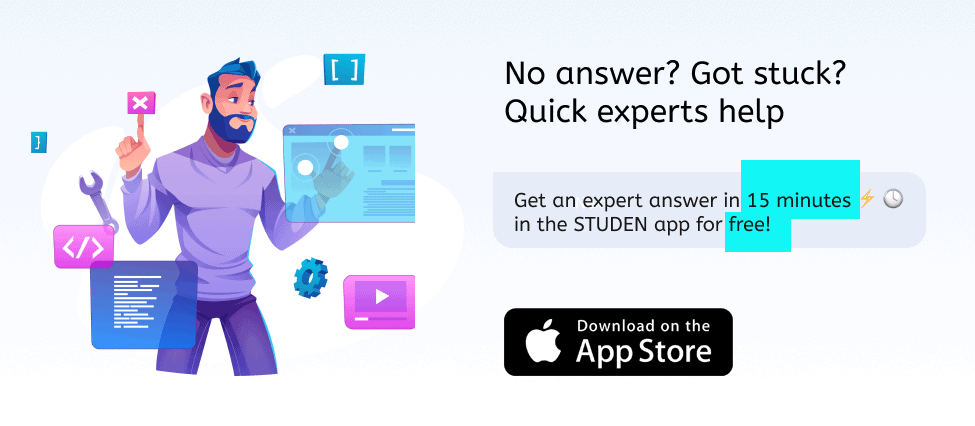
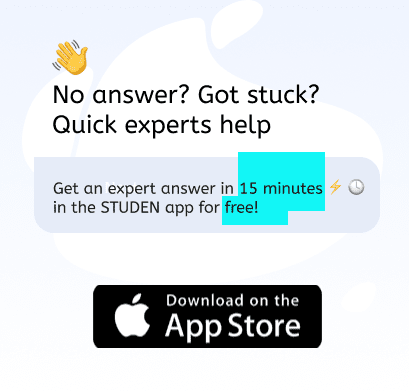
Another question on Computers and Technology

Computers and Technology, 22.06.2019 08:30
Today is the anniversary of me being on yet, i don't need it anymore! here's a picture of my dog wearing a bowtie! my question is, how do i delete my account?
Answers: 1

Computers and Technology, 22.06.2019 19:20
1)consider the following code snippet: #ifndef book_h#define book_hconst double max_cost = 1000.0; class book{public: book(); book(double new_cost); void set_cost(double new_cost); double get_cost() const; private: double cost; }; double calculate_terms(book bk); #endifwhich of the following is correct? a)the header file is correct as given.b)the definition of max_cost should be removed since header files should not contain constants.c)the definition of book should be removed since header files should not contain class definitions.d)the body of the calculate_terms function should be added to the header file.
Answers: 1

Computers and Technology, 22.06.2019 21:30
Nathan wants to create multiple worksheet containing common formatting styles for his team members. which file extension him to save these worksheets? nathan to create multiple worksheets with common styles. he needs to save them with the extension.
Answers: 1

Computers and Technology, 22.06.2019 23:30
Which of the following is not a symptom of chronic fatigue syndrome
Answers: 2
You know the right answer?
C++ Write a program that first asks the user which Temperature scale conversion he/she would like to...
Questions

Mathematics, 08.05.2021 02:30
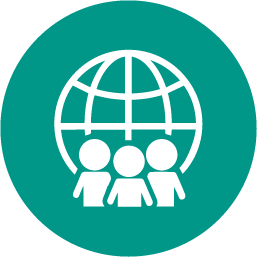
History, 08.05.2021 02:30

Mathematics, 08.05.2021 02:30





Geography, 08.05.2021 02:30
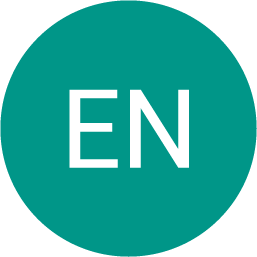

Mathematics, 08.05.2021 02:30

Mathematics, 08.05.2021 02:30
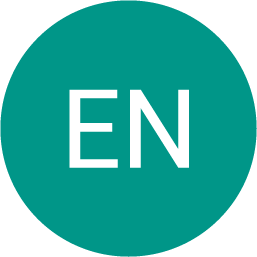

Mathematics, 08.05.2021 02:30
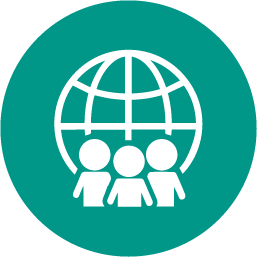

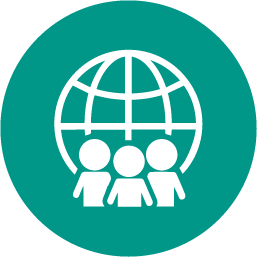
History, 08.05.2021 02:30

Mathematics, 08.05.2021 02:30

Chemistry, 08.05.2021 02:30
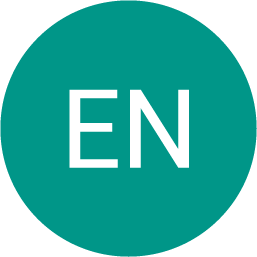