
Computers and Technology, 05.11.2020 19:10 ArelysMarie
Write a program that will sort an array of data using the following guidelines - DO NOT USE VECTORS, COLLECTIONS, SETS or any other data structures from your programming language.
C++
Ask the user for the number of elements, not to exceed SORT_MAX_SIZE = 16 (put appropriate input validation)
Ask the user for the type of data they will enter - Dollar or CIS22C_Dollar objects from Lab 1.
Use your Dollar and CIS22C_Dollar classes from Lab 1 as-is without making any changes. If you do make changes to be able to complete this lab, then there will be a small penalty.
Based on the input, create an appropriate array for the data to be entered.
Write a helper function called RecurMergeSort such that: It is a standalone function not part of any class of Lab 1, Takes in the same type of parameters as any standard MergeSort with recursion behavior, i. e. void MergeSort(Dollar arr[], int size) Prints out how the array looks every time a recursive step returns back to its caller You might need to pass in a third parameter which identifies the array to be printed - this is language dependent.
In your main, allow the user to enter data of their own choosing up to their chosen array size. There is no sample output - you are allowed to provide user interactivity as you see fit but programs will be graded for clarity of interaction.
Then sort the array using your sort function of step 5.
Take screenshots to be uploaded with the project. In your main, make sure that the output is being written to console as well as an output file at the same time.
Do not copy and paste text from your console to create the output file.
Ensure input and any other data validations as needed and provide descriptive prompts with emphasis on usability. Upload your classes from Lab 1 and new code files for Lab 3, output file and the screenshots in one zip file.
The Dollar and CISDOLLAR class are as follows:
class Dollar // Dollar class
{
private:
int noteValue;
int coinValue;
string currencyName;
public:
Dollar() {}; // default constructor
Dollar(int note, int coin) { // parameterized constructor
noteValue = note;
coinValue = coin;
}
~Dollar() { // copy constructor
};
// setters and getters.
int getNoteValue() {
return noteValue;
}
void setNoteValue(int x) {
noteValue = x;
}
int getCoinValue() {
return coinValue;
}
void setCoinValue(int x) {
coinValue = x;
}
Dollar operator +(Dollar obj) {
Dollar obj2;
obj2.noteValue = noteValue + obj. noteValue;
obj2.coinValue = coinValue + obj. coinValue;
return obj2;
}
Dollar operator -(Dollar obj) {
Dollar obj2;
obj2.noteValue = this->noteValue - obj. noteValue;
obj2.coinValue = this->coinValue - obj. coinValue;
return obj2;
}
bool operator ==(Dollar obj) {
if ((this->noteValue == obj. noteValue) && (this->coinValue == obj. coinValue))
return true;
else
return false;
}
double dollarToCsdollar(int note, int coin) // converter from Dollar to CIS22CDollar
{
double amount = (note + coin * 0.01);
return amount * 1.36;
}
double dollarAmount(int note, int coin) //Total calculator for Dollar
{
cout << "Dollars:" << (note + coin * 0.01);
return (note + coin * 0.01);
}
};
class CIS22CDollar :public Dollar { // CIS22CDollar class, derived from Dollar Class;
private:
int noteValue;
int coinValue;
double conversionFactor;
double convertTo22C()
{
conversionFactor = 1.36;
return conversionFactor;
}
double convertToDollar() {
conversionFactor = 0.74;
return conversionFactor;
}
public:
CIS22CDollar() {}; //default constructor
CIS22CDollar(int note, int coin) { // parameterized constructor
noteValue = note;
coinValue = coin;
};
~CIS22CDollar() {}; // copy constructor
// Setters and getters.
int getNoteValue() {
return noteValue;
}
void setNoteValue(int x) {
noteValue = x;
}
int getCoinValue() {
return coinValue;
}
void setCoinValue(int y) {
coinValue = y;
}
double conversionToDollar(int note, int coin) { // converter to DOLLAR class
double amount = (note + coin * 0.001);
return amount * 0.74;
}
double cisDollarAmount(int note, int coin) { // total number of cents which is used later in code.
(note + coin * 0.01);
}
};

Answers: 2
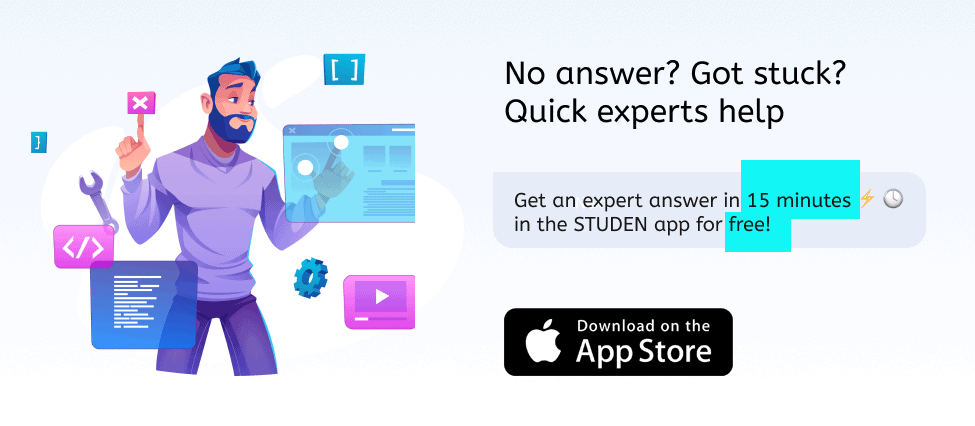
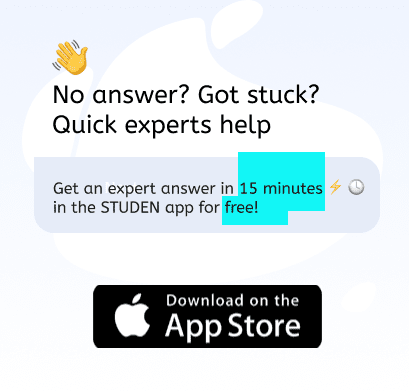
Another question on Computers and Technology

Computers and Technology, 22.06.2019 23:30
To check spelling errors in a document, the word application uses the to determine appropriate spelling. internet built-in dictionary user-defined words other text in the document
Answers: 1

Computers and Technology, 23.06.2019 01:30
Jason works as an accountant in a department store. he needs to keep a daily record of all the invoices issued by the store. which file naming convention would him the most? a)give the file a unique name b)name the file in yymmdd format c)use descriptive name while naming the files d)use capital letters while naming the file
Answers: 3

Computers and Technology, 23.06.2019 06:20
What is a point-in-time measurement of system performance?
Answers: 3

Computers and Technology, 23.06.2019 14:30
Select the correct answer. which step can possibly increase the severity of an incident? a. separating sensitive data from non-sensitive data b. immediately spreading the news about the incident response plan c. installing new hard disks d. increasing access controls
Answers: 2
You know the right answer?
Write a program that will sort an array of data using the following guidelines - DO NOT USE VECTORS,...
Questions

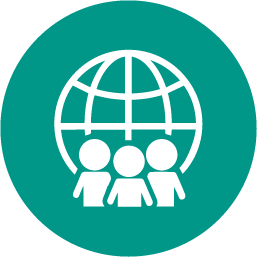
History, 30.01.2021 01:00
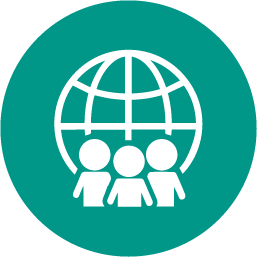
History, 30.01.2021 01:00

Chemistry, 30.01.2021 01:00
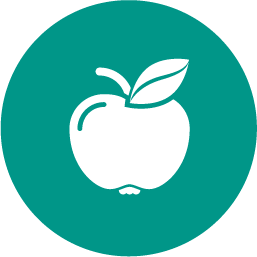

Chemistry, 30.01.2021 01:00


Mathematics, 30.01.2021 01:00



Mathematics, 30.01.2021 01:00

Mathematics, 30.01.2021 01:00

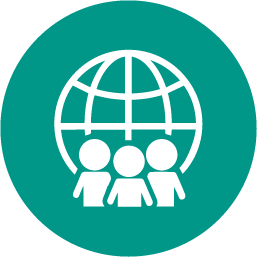

Mathematics, 30.01.2021 01:00

Mathematics, 30.01.2021 01:00

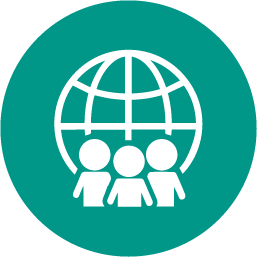
