
Computers and Technology, 12.11.2020 19:10 21villalobosjabez
Write a Python program string_functions. py that defines several functions. Each function re-implements Python's built-in string methods.1. my_compare(str1, str2): Takes two strings as parameters (str1, str2) and returns a -1 if str1 is alphabetically less than str2, returns a 0 if str1 is alphabetically equal to str2, and returns a 1 if str1 is alphabetically greater than str2. 2. is_char_in(string, char): Takes a string and a character as parameters and returns True if the character is in the string or False if it is not.3. is_char_not_in(string, char): Takes a string and a character as parameters and returns True if the character is NOT in the string or False if it is.4. my_count(string, char): Takes a string and a character as parameters and returns an integer count for each occurrence of the character. For example, if the function takes in 'abracadabra' and 'a', the function should return 5.5. my_endswith(string, char): Takes a string and a character as parameters and returns True if the character is the last character in the string or False if it is not. For example, if the function takes in 'quartz' and 'z', the function should return True. If the function takes in 'quartz' and 'q', the function should return False.6. my_find(string, char): Takes a string and a character as parameters and returns the first index from the left where the character is found. If it does not find the character, return -1. For example, if the function takes in ‘programming’ and ‘g’, it should return 3. Do not use str. find() for this.7. my_replace(string, char1, char2): Takes a string and two characters (char1 and char2) as parameters and returns a string with all occurrences of char1 replaced by char2. For example, if the string is ‘bamboozle’ and char1 is ‘b’ and char2 is ‘t’ then your function should return ‘tamtoozle’.8. my_upper(string): Takes a string as a parameter and returns it as a string in all uppercase. For example, 'victory' would be 'VICTORY'. Hint: Use the ord(c) function to get the ASCII / Unicode code-point. For example, ord('a') returns the integer 97. Use chr(i) function to convert an integer back to a character. For example, chr(97) returns the string 'a'. You cannot use the built-in string function str. upper() for this.9. my_lower(string): Takes a string as a parameter and returns it as a string in all lowercase. Hint: Similar to the previous item, use ord() and chr(). Do not use str. lower() for this. Extra Credit (10 points): my_title(string): Takes a string and returns a string with the first character capitalized for every word. For example, if the input to the function is "I like Python a lot", the function should return the string "I Like Python A Lot".Note- You may not use Python's built-in string methods - e. g., str. find(), str. replace(), str. lower(), lstrip(), startswith(), endswith(), join() … - to implement yours. However, you *should* use them to test your functions to see if they produce the same results. You can do this in the main or in another defined function which is called by the main. You can use all other Python functions.

Answers: 2
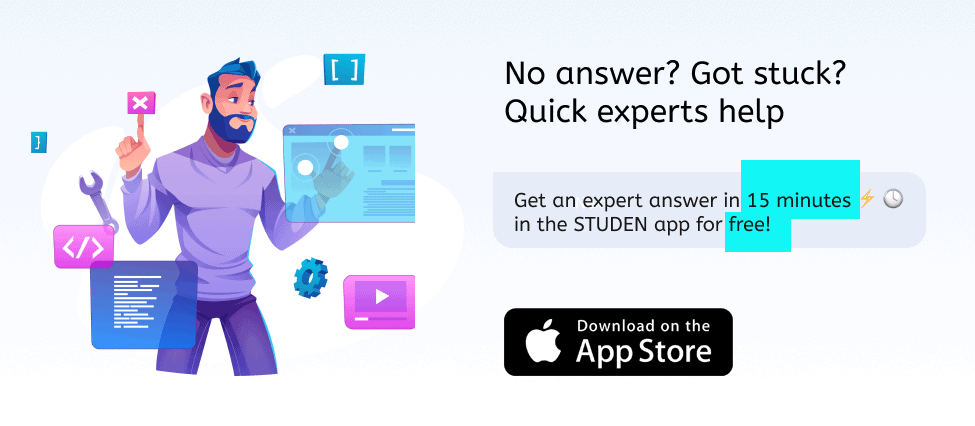
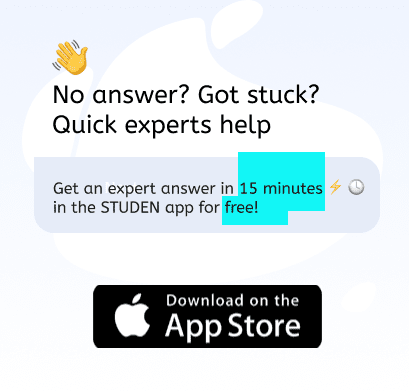
Another question on Computers and Technology


Computers and Technology, 22.06.2019 18:00
Suppose an astronomer discovers a large, spherical-shaped body orbiting the sun. the body is composed mostly of rock, and there are no other bodies sharing its orbit. what is the best way to categorize this body? a. planet b. moon c. comet d. asteroid
Answers: 1

Computers and Technology, 22.06.2019 23:30
Define a function printfeetinchshort, with int parameters numfeet and numinches, that prints using ' and " shorthand. ex: printfeetinchshort(5, 8) prints: 5' 8"
Answers: 1

Computers and Technology, 23.06.2019 09:50
Allison and her group have completed the data entry for their spreadsheet project. they are in the process of formatting the data to make it easier to read and understand. the title is located in cell a5. the group has decided to merge cells a3: a7 to attempt to center the title over the data. after the merge, allison points out that it is not centered and looks bad. where would the title appear if allison unmerged the cells in an attempt to fix the title problem?
Answers: 2
You know the right answer?
Write a Python program string_functions. py that defines several functions. Each function re-impleme...
Questions

Mathematics, 24.04.2021 03:30

Mathematics, 24.04.2021 03:30
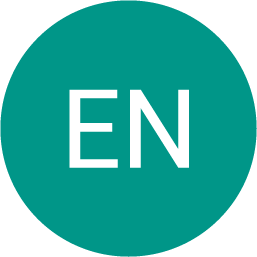

Biology, 24.04.2021 03:30


Mathematics, 24.04.2021 03:30

Mathematics, 24.04.2021 03:30
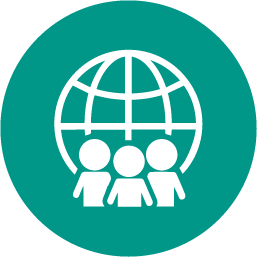
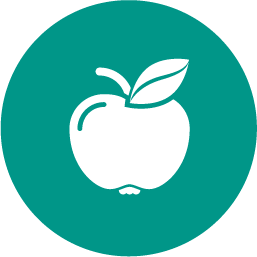
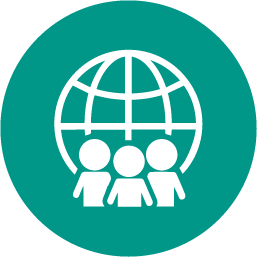

Chemistry, 24.04.2021 03:30


Mathematics, 24.04.2021 03:30


Mathematics, 24.04.2021 03:30

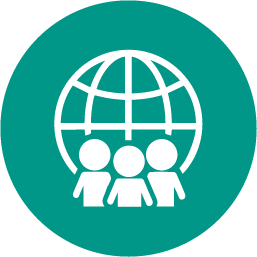
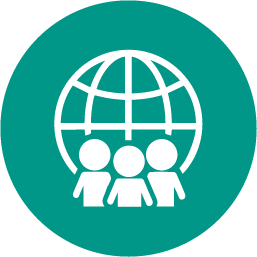

Biology, 24.04.2021 03:30