
Computers and Technology, 18.11.2020 01:50 girlieredc
PLEASE HELP! I can figure out how to do it, but not how to get it to fit into the template. And this is Java.
Part A:
Open the file BloodData. java that includes fields that hold a blood type (the four blood types are O, A, B, and AB) and an Rh factor (the factors are + and –). Create a default constructor that sets the fields to “O” and “+”, and an overloaded constructor that requires values for both fields. Include get and set methods for each field. Open the file TestBloodData. java and click the Run button to demonstrate that each method works correctly.
Part B:
Open the file Patient. java which includes an ID number, age, and BloodData. Provide a default constructor that sets the ID number to “0”, the age to 0, and the BloodData to “O” and “+”. Create an overloaded constructor that provides values for each field. Also provide get methods for each field. Open TestPatient. java. Click the Run button at the bottom of your screen to demonstrate that each method works correctly.
Has to fit the below template
BloodData. java
public class BloodData
{
// declare private variables here
public BloodData()
{
// add default constructor values here
}
public BloodData(String bType, String rh)
{
// add constructor logic here
}
public void setBloodType(String bType)
{
// add method code here
}
public String getBloodType()
{
// add method code here
}
public void setRhFactor(String rh)
{
// add method code here
}
public String getRhFactor()
{
// add method code here
}
}
Patient. java
public class Patient
{
// declare private variables here
public Patient()
{
// add default constructor values here
}
public Patient(String id, int age, String bType, String rhFactor)
{
// add constructor logic here
}
public String getId()
{
// write method code here
}
public void setId(String id)
{
// write method code here
}
public int getAge()
{
// write method code here
}
public void setAge(int age)
{
// write method code here
}
public BloodData getBloodData()
{
// write method code here
}
public void setBloodData(BloodData b)
{
// write method code here
}
}
TestBloodData. java
public class TestBloodData
{
public static void main(String[] args)
{
BloodData b1 = new BloodData();
BloodData b2 = new BloodData("A", "-");
display(b1);
display(b2);
b1.setBloodType("AB");
b1.setRhFactor("-");
display(b1);
}
public static void display(BloodData b)
{
System. out. println("The blood is type " + b. getBloodType() + b. getRhFactor());
}
}
TestPatient. java
public class TestPatient
{
public static void main(String[] args)
{
Patient p1 = new Patient();
Patient p2 = new Patient("1234", 35, "B", "+");
BloodData b2 = new BloodData("A", "-");
display(p1);
display(p2);
p1.setId("3456");
p1.setAge(42);
BloodData b = new BloodData("AB", "-");
p1.setBloodData(b);
display(p1);
}
public static void display(Patient p)
{
BloodData bt = p. getBloodData();
System. out. println("Patient #" + p. getId() + " age: " + + p. getAge() +
"\n The blood is type " + bt. getBloodType() + bt. getRhFactor());
}
}

Answers: 1
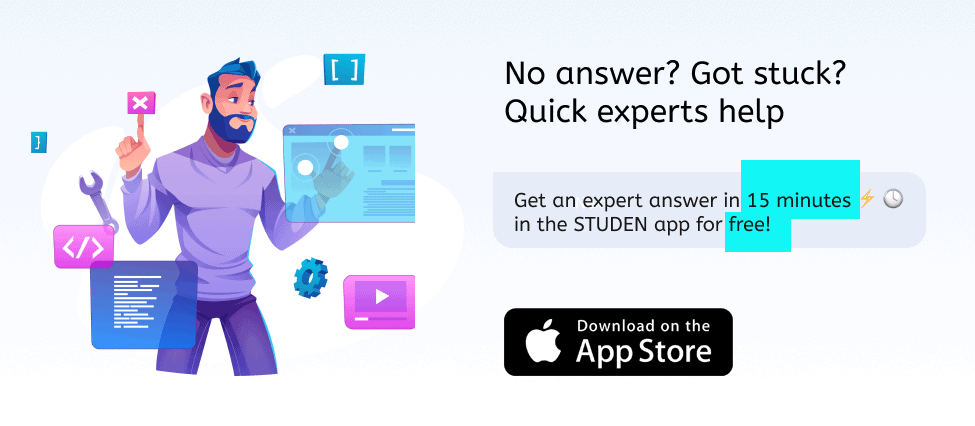
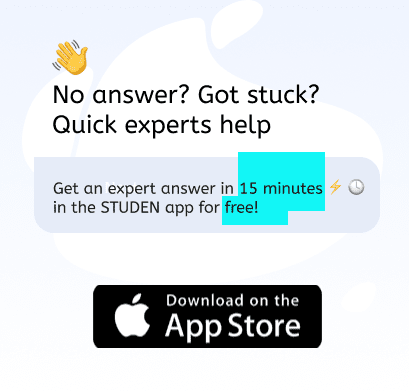
Another question on Computers and Technology

Computers and Technology, 22.06.2019 08:10
Alook-up table used to convert pixel values to output values on a monitor. essentially, all pixels with a value of 190 or above are shown as white (i.e. 255), and all values with a value of 63 or less are shown as black (i.e. 0). in between the pixels are scaled so that a pixel with a value p is converted to a pixel of value 2/127 −+3969). if a pixel has a value of 170 originally, what value will be used to display the pixel on the monitor? if a value of 110 is used to display the pixel on the monitor, what was the original value of the pixel?
Answers: 1

Computers and Technology, 22.06.2019 08:50
Can online classes such as gradpoint track your ip location like if im taking a final and i give somebody else my account and they take the final for me will it show where they are taking the final from? and can this be countered with a vpn
Answers: 1

Computers and Technology, 22.06.2019 16:30
The most common battery cable terminal is a that provides a large surface contact area with the ability to tighten the terminal onto the battery post using a nut and bolt.
Answers: 2

Computers and Technology, 22.06.2019 19:30
Avariable definition defines the name of a variable that will be used in a program, as well as
Answers: 3
You know the right answer?
PLEASE HELP! I can figure out how to do it, but not how to get it to fit into the template. And this...
Questions
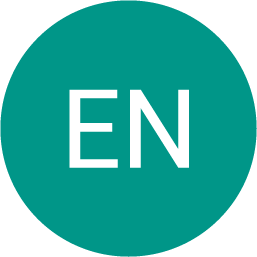
English, 07.10.2019 15:00
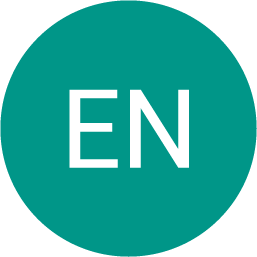
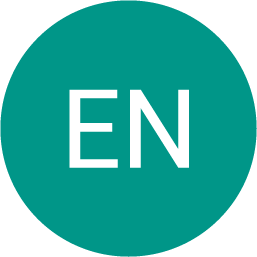
English, 07.10.2019 15:00

Mathematics, 07.10.2019 15:00
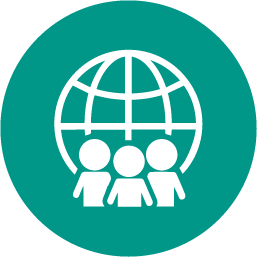

Mathematics, 07.10.2019 15:00
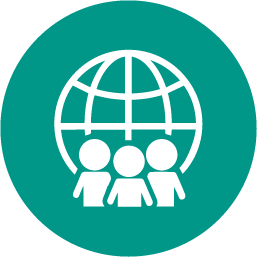
History, 07.10.2019 15:00


Mathematics, 07.10.2019 15:00

Chemistry, 07.10.2019 15:00
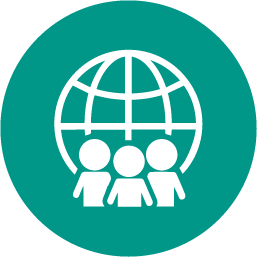
History, 07.10.2019 15:00

Mathematics, 07.10.2019 15:00

Mathematics, 07.10.2019 15:00

Biology, 07.10.2019 15:00
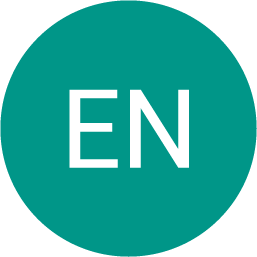
English, 07.10.2019 15:00

Mathematics, 07.10.2019 15:10
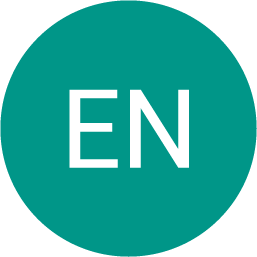

Mathematics, 07.10.2019 15:10

Mathematics, 07.10.2019 15:10