
Computers and Technology, 08.12.2020 02:30 brenda0014
I keep receiving an error for out of bounds index 0. How do I solve this?
// read 2 strings from the command line; each string will contain an integer
// let's call those integers, num1 and num2
// write a loop that goes from num1 and num2 looking for prime numbers
// if a number if prime then store it in an array of integers
// pass this array to a method that returns the sum of all the numbers in the array; you will also need to tell this method how many numbers are in the array
// output only the sum of primes
// you may assume a maximum of not more than 1000 numbers in the array
import java. util.*;
public class Program {
// go thru the array primes which has size elements
// total the integer values in primes
// return this total
// the first argument is the array of prime numbers
// the second argument is the number of primes in this array
public static int sumArray(int[] primes, int n) {
int total = 0;
for (int i = 0; i < n; i++) {
total += primes[i];
}
return total;
}
// this method takes an integer, n, and determines if it is prime
// returns true if it is and false if it isn't
// a number is prime if it is only evenly divisible by 1 and itself
// 1 is NOT considered prime
static boolean isPrime(int n) {
// Corner case
if (n <= 1)
return false;
// Check from 2 to n-1
for (int i = 2; i < n; i++)
if (n % i == 0)
return false;
return true;
}
public static void main(String[] args) {
// define the constant MAX which represents the largest size of the primes array
final int MAX = 1000;
int num1, num2, numPrime = 0;
int[] primes = new int[MAX];
// convert 1st command line argument from a string to an integer
num1 = Integer. parseInt(args[0]);
num2 = Integer. parseInt(args[1]);
// get num2 from the command line
// loop through all integers from num1 to num2
// store those that are prime in the primes array
// keep track of the number of primes - numPrime
for (int i = num1; i <= num2; i++) {
if (isPrime(i)) {
primes[numPrime++] = i;
}
}
// output the sum of all the primes
System. out. println(sumArray(primes, numPrime));
}
}

Answers: 1
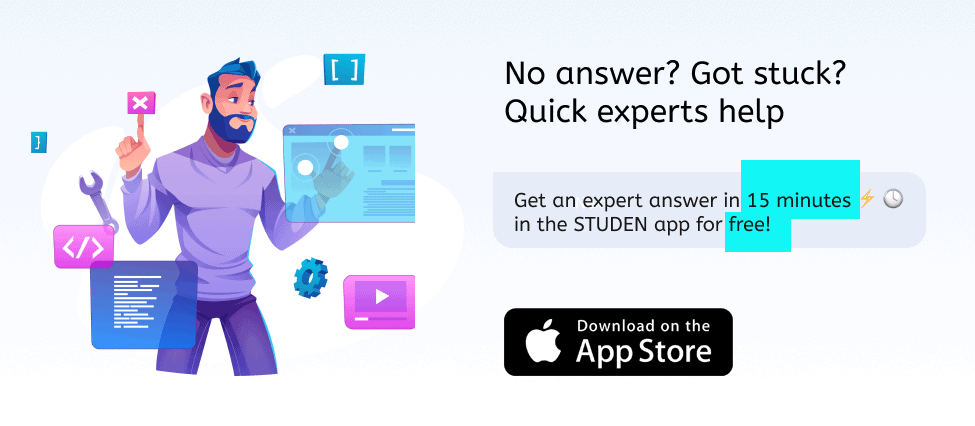
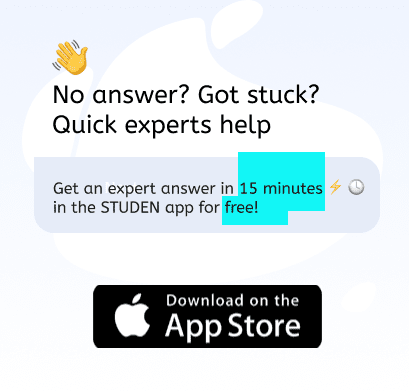
Another question on Computers and Technology

Computers and Technology, 22.06.2019 01:30
How will you cite information that is common knowledge in your research paper?
Answers: 1

Computers and Technology, 24.06.2019 02:00
How are we able to create photographs differently than 100 years ago? explain your answer in relation to your photograph you selected.
Answers: 1

Computers and Technology, 25.06.2019 08:00
When date is processed into a meaningful form, i becomes
Answers: 1

Computers and Technology, 25.06.2019 22:20
Lenny wants to use his coworker's computer system. when he arrives at his coworker's workstation, the screen is blank. what could the issue be? the computer is not turned on. there is no internet connection. the keyboard is not functioning. the computer is in sleep mode.
Answers: 2
You know the right answer?
I keep receiving an error for out of bounds index 0. How do I solve this?
// read 2 strings from th...
Questions
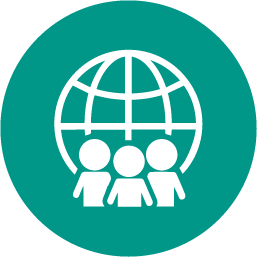










Mathematics, 02.07.2019 13:00
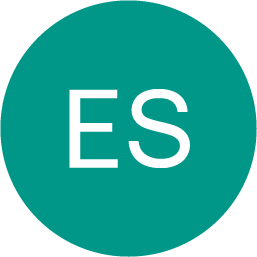
Spanish, 02.07.2019 13:00






Mathematics, 02.07.2019 13:00


Computers and Technology, 02.07.2019 13:00