Object Oriented Tests
For this challenge, you are going to build a mock comments section.
De...

Computers and Technology, 12.01.2021 17:30 lily2998
Object Oriented Tests
For this challenge, you are going to build a mock comments section.
Design
We're going to focus on two aspects:
Users
Users come in 3 flavors, normal users, moderators, and admins. Normal users can only create new comments, and edit the their own comments. Moderators have the added ability to delete comments (to remove trolls), while admins have the ability to edit or delete any comment.
Users can log in and out, and we track when they last logged in
Comments
Comments are simply a message, a timestamp, and the author.
Comments can also be a reply, so we'll store what the parent comment was.
Your Challenge
This is challenge is not about building a fully functional API, but more about focusing on the design from an object-oriented point-of-view.
We've provided the basic API (which is incomplete), which we would like you to complete being aware of the following Object-Oriented Programming concepts:
Encapsulation of Properties
All classes should have no publicly accessible fields
You should make sure you at least "hide" the required fields, for example, using _name instead of _name. Alternatively, feel free to use a better solution as extra credit.
The method-based API is provided. These must be completed as-is.
Additional methods are allowed, though remember to keep read-only properties read-only.
Instantiation
Classes should be instantiated with properties (as provided), to create instances with values already assigned.
User/Moderator/Admin defaults:
Should be marked as not logged in
Should return null for the last logged in at property
Comment defaults:
Should set the current timestamp for the created at property upon instantiation
Replied To is optional, and should be null if not provided.
Inheritance & Access Control
Note: for the sake of simplicity, you can simply treat object equality as "equal", though more complete solutions will also pass.
User
Users can be logged in and out.
When logging in, set the lastLoggedInAt timestamp. Do not modify this timestamp when logging out
Users can only edit their own comments
Users cannot delete any comments
Moderator is a User
Moderators can only edit their own comments
Moderators can delete any comments
Admin is both a User and a Moderator
Admins can edit any comments
Admins can delete any comments
Composition
Comments contain a reference to the User who created it (author)
Comments optionally contain a reference to another comment (repliedTo)
When converting to a string (toString), the following format is used:No replied to:
«message» by «author. name»
With replied to:
«message» by «author. name» (replied to «repliedTo. author. name»)
// Code
class User {
constructor(name) {}
isLoggedIn() {}
getLastLoggedInAt() {}
logIn() {}
logOut() {}
getName() {}
setName(name) {}
canEdit(comment) {}
canDelete(comment) {}
}
class Moderator {}
class Admin {}
class Comment {
constructor(author, message, repliedTo) {}
getMessage() {}
setMessage(message) {}
getCreatedAt() {}
getAuthor() {}
getRepliedTo() {}
toString() {}
}
//Sample tests:
var should = require('should');
describe('OOP Tests', function() {
it('example tests', function() {
var user = new User("User 1");
user. getName().should. eql('User 1', 'User name is set correctly');
var mod = new Moderator("Moderator");
mod. should. be. an. instanceof(User, 'Moderator is a User');
});
});

Answers: 2
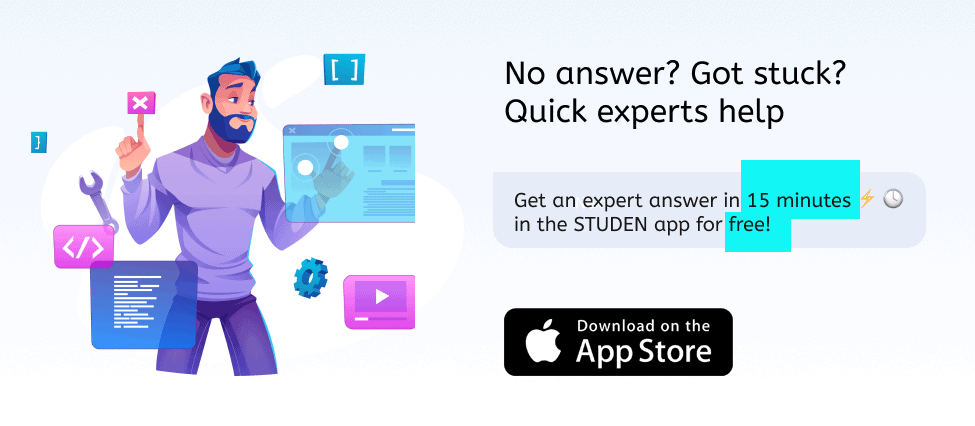
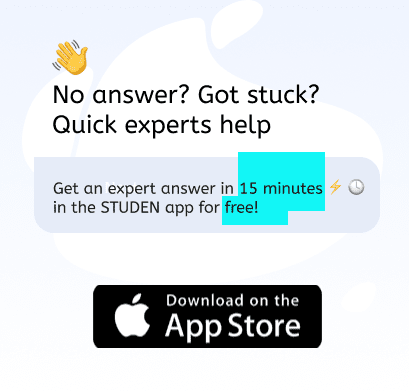
Another question on Computers and Technology

Computers and Technology, 22.06.2019 16:30
Corey set up his presentation for delivery to his team.the information he had to convey was critical to their job performance.he knew he would need a lot of time to explain each point
Answers: 3

Computers and Technology, 24.06.2019 02:10
Consider the usual algorithm to convert an infix expression to a postfix expression. suppose that you have read 10 input characters during a conversion and that the stack now contains these symbols: (5 points) | | | + | | ( | bottom |_*_| now, suppose that you read and process the 11th symbol of the input. draw the stack for the case where the 11th symbol is
Answers: 2

Computers and Technology, 24.06.2019 05:30
Someone plzz me which of these defines a social search? a. asking a search engine a question that is answered by a real person on the other sideb. modifying search results based on popularity of a web pagec.modifying search results based on a ranking of a web page
Answers: 2

Computers and Technology, 24.06.2019 16:30
You may see the term faq on websites which stands for frequently asked questions this is an example of which type of mnemonic? a) poem b) acronym c) acrostic d) abbreviation ken has dipped many dark chocolate marshmallows (which you remember the metric system distance units in decreasing order: kilometers, hectometer, decameter, centimeter, millimeter) is an example of which type of mnemonic? a) poem b) acronym c) acrostic d) abbreviation !
Answers: 1
You know the right answer?
Questions

Business, 18.01.2020 04:31








Mathematics, 18.01.2020 04:31

Mathematics, 18.01.2020 04:31
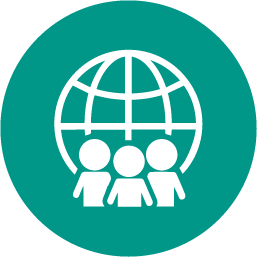
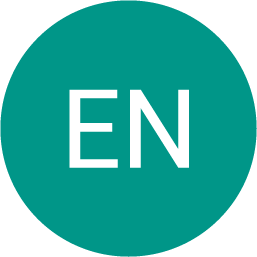
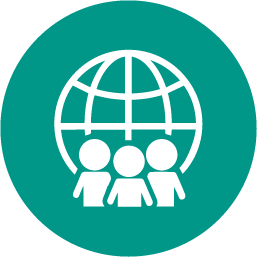

Mathematics, 18.01.2020 04:31


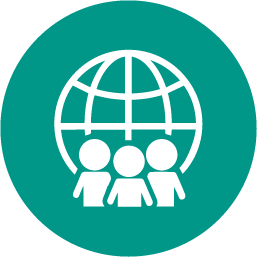
History, 18.01.2020 04:31
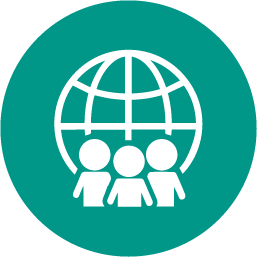
History, 18.01.2020 04:31

Biology, 18.01.2020 04:31

Mathematics, 18.01.2020 04:31