
Computers and Technology, 31.01.2021 22:50 MarishaTucker
Please help!
Consider this implementation of the class Card, that stores information related to a cards monetary value, name, condition, and number in a set of collectible cards:
public class Card
{
private double value;
private String name;
private int setNum; //each card has its own, unique setNum
private String condition;
public Card (String nom, String cond, double val, int numSet){
name = nom;
condition = cond;
value = val;
setNum = numSet;
}
public String getName(){
return name;
}
public String getCondition(){
return condition;
}
public double getValue(){
return value;
}
public int getSetNum(){
return setNum;
}
public String toString()
{
return name + ":: " + setNum;
}
}
A subsequent class CardCollection has been created with the instance variable ArrayList collection that stores a collection of cards.
import java. util. ArrayList;
public class CardCollection
{
ArrayList collection;
double totalVal;
public CardCollection(ArrayList myColl)
{
collection = myColl;
}
public double totalValue()
{
// code intentionally left blank
// you will write this method in #1
}
public String checkPerfect()
{
// code intentionally left blank
// you will write this method in #2
}
public ArrayList orderNumerically()
{
System. out. println("Before sort: " + collection);
// code intentionally left blank
System. out. println("After sort: " + sortedCards);
return collection;
}
}
The driver class CardRunner is shown below:
import java. util. ArrayList;
public class CardRunner
{
public static void main (String[] args)
{
ArrayList x = new ArrayList ();
// you will write this code in #3
x. add(new Card("Downey", "new", 48.72, 7));
x. add(new Card("Jones", "perfect", 200, 5));
x. add(new Card("Smith", "perfect", 24.8, 3));
x. add(new Card("Peek", "good", 13.5, 1));
System. out. println("\nThe total value of my collection is: $" + // #4 );
System. out. println("\nMy perfect cards are: " + // code intentionally left blank);
System. out. println("\n" + // code intentionally left blank);
}
}
1) Write a method totalValue() for CardCollection which returns the total value of all cards in a card collection.
2) Write a method checkPerfect() for CardCollection that prints the name of the Cards in a collection that are in "perfect" condition. 3) Instantiate a CardCollection object which could be used to access methods from the CardCollection class.
4) Complete this programming statement from CardRunner
System. out. println("\nThe total value of my collection is: $" + // #4 );

Answers: 1
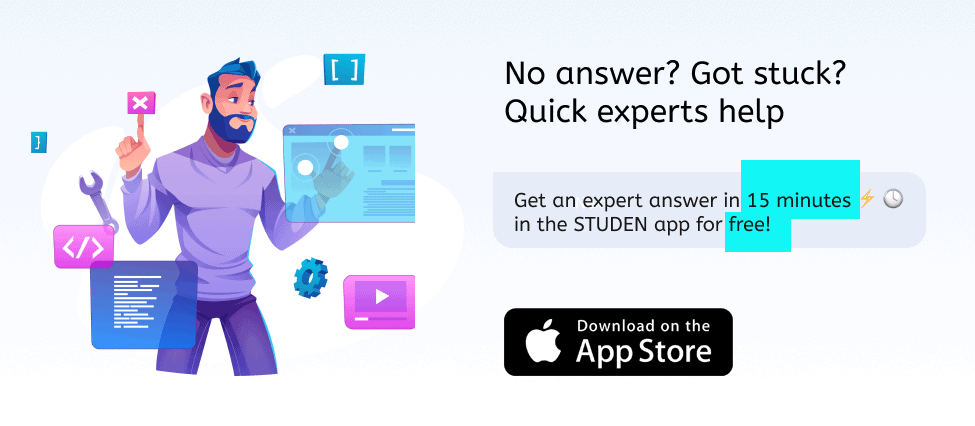
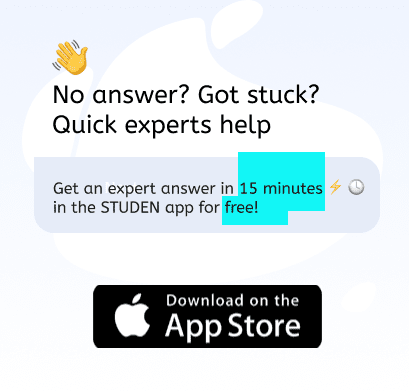
Another question on Computers and Technology

Computers and Technology, 22.06.2019 22:40
When you type the pwd command, you notice that your current location on the linux filesystem is the /usr/local directory. answer the following questions, assuming that your current directory is /usr/local for each question. a. which command could you use to change to the /usr directory using an absolute pathname? b. which command could you use to change to the /usr directory using a relative pathname? c. which command could you use to change to the /usr/local/share/info directory using an absolute pathname? d. which command could you use to change to the /usr/local/share/info directory using a relative pathname? e. which command could you use to change to the /etc directory using an absolute pathname? f. which command could you use to change to the /etc directory using a relative pathname?
Answers: 3

Computers and Technology, 23.06.2019 17:00
1. which of the following is not an example of an objective question? a. multiple choice. b. essay. c. true/false. d. matching 2. why is it important to recognize the key word in the essay question? a. it will provide the answer to the essay. b. it will show you a friend's answer. c. it will provide you time to look for the answer. d. it will guide you on which kind of answer is required.
Answers: 1

Computers and Technology, 24.06.2019 02:00
What is a loop? a. a collection of function definitions at the top of a program b. a line of code that defines a variable and assigns it a value c. a program that opens the turtle graphics window d. a block of code that repeats a specific number of times
Answers: 1

You know the right answer?
Please help!
Consider this implementation of the class Card, that stores information related to a c...
Questions
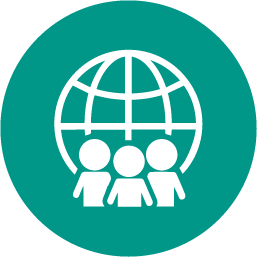
History, 03.05.2021 03:30
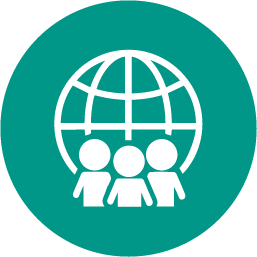



Mathematics, 03.05.2021 03:30


Chemistry, 03.05.2021 03:30

Mathematics, 03.05.2021 03:30




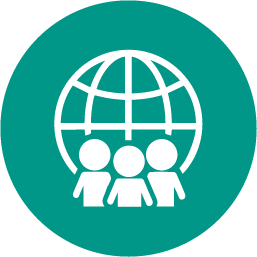
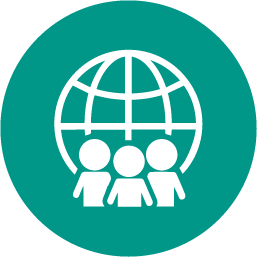
History, 03.05.2021 03:30

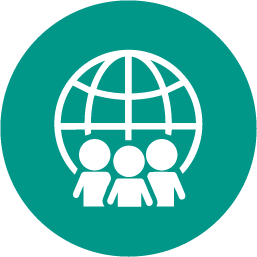
World Languages, 03.05.2021 03:30

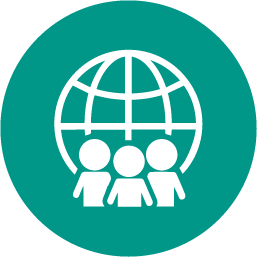
History, 03.05.2021 03:30
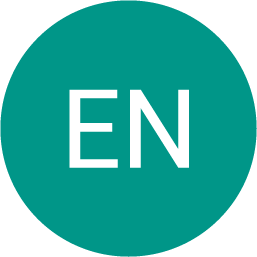