
Computers and Technology, 09.02.2021 07:40 al8ce
I wrote the move function of this phython code:
but it is not working here correctly, Karel is only moving one space.
Here are the directions:
YOUR JOB
Karel doesn’t know how to move! Since we aren’t using the built in Karel commands, we’ll have to make the image of Karel move using Python!
Your job is to implement the move() function.
You do not need to worry about running into walls.
You do not need to modify any of the other code function. The final result should have Karel move forward (east) twice, then move in a square. Karel should end on the first street, fourth avenue, facing south.
HINTS
Your move function should change the X_POS and Y_POS variable based on the direction that Karel is facing. For example, if direction is NORTH, then Karel should move in the negative y direction. If direction is SOUTH, then Karel should move in the positive y direction, etc.
Once you update the X_POS or Y_POS, you can then set a new position by calling the .set_position method.
Karel should move a distance of KAREL_SIZE.
# This program creates Karel without any of the builtin Karel commands,
# it makes Karel from scratch using Python.
#
# In this program, we build Karel's World in Python.
NUM_STREETS = 4
NUM_AVES = 4
POINT_SIZE = 3
WORLD_WIDTH = 275
WORLD_HEIGHT = 275
set_size(WORLD_WIDTH, WORLD_HEIGHT)
STREET_HEIGHT = WORLD_HEIGHT // NUM_STREETS
AVE_WIDTH = WORLD_WIDTH // NUM_AVES
PAUSE_TIME = 1000
# Constants for creating Karel
KAREL_IMG_URL = "https://codehs. com/uploads/9657058ec012105e0c5548c 917c29761"
KAREL_SIZE = STREET_HEIGHT
# Starting position for Karel
X_POS = 0
Y_POS = WORLD_HEIGHT - KAREL_SIZE
# represents angles of rotation
EAST = 0
SOUTH = math. radians(90)
WEST = math. radians(180)
NORTH = math. radians(270)
# Creates Karel's world with Karel in the bottom left corner facing east.
def setup_world():
global direction, karel
# Add the points to the grid
for street in range(NUM_STREETS):
for ave in range(NUM_AVES):
x_center = ave * AVE_WIDTH + AVE_WIDTH / 2
y_center = street * STREET_HEIGHT + STREET_HEIGHT / 2
dot = Circle(POINT_SIZE, x_center, y_center)
add(dot)
# Add Karel to the grid
karel = Image(KAREL_IMG_URL)
karel. set_position(X_POS, Y_POS)
karel. set_size(KAREL_SIZE, KAREL_SIZE)
add(karel)
# Variables to keep track of karel and karel's direction
# Set Karel's initial direction
direction = EAST
def turn_left():
global karel, direction
if direction == EAST:
direction = NORTH
elif direction == WEST:
direction = SOUTH
elif direction == NORTH:
direction = WEST
elif direction == SOUTH:
direction = EAST
else:
print("Error: Karel's Direction is not properly set.")
direction = EAST
karel. set_rotation(direction)
# Copy your code from the last exercise
def turn_right():
global karel, direction
if direction == EAST:
direction = SOUTH
elif direction == WEST:
direction = NORTH
elif direction == NORTH:
direction = EAST
elif direction == SOUTH:
direction = WEST
else:
print("Error: Karel's Direction is not properly set.")
direction = WEST
karel. set_rotation(direction)
# Implement this function!
def move():
global X_POS, Y_POS
if direction == EAST:
karel. set_position(X_POS + KAREL_SIZE, Y_POS)
def move_again():
global X_POS, Y_POS
if direction == EAST:
karel. set_position(X_POS + KAREL_SIZE, Y_POS)
def move_move():
global X_POS, Y_POS
if direction == EAST:
karel. set_position(X_POS + KAREL_SIZE, Y_POS)
setup_world()
move()
move_again()
move_move()

Answers: 1
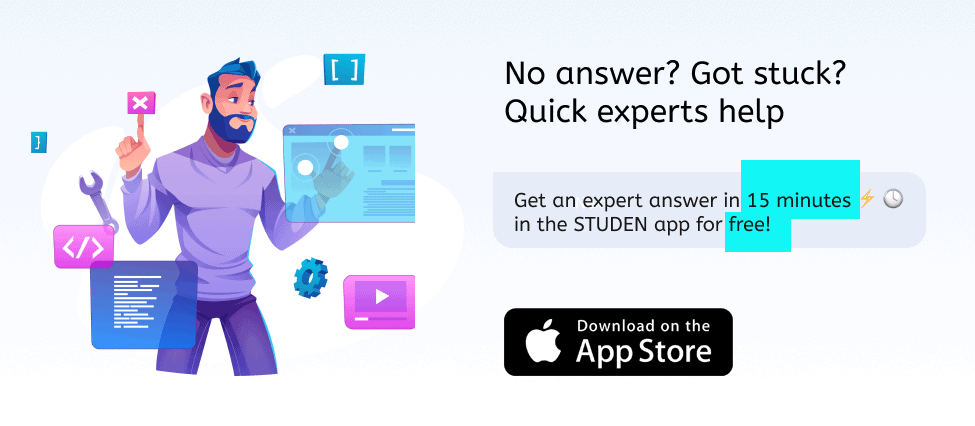
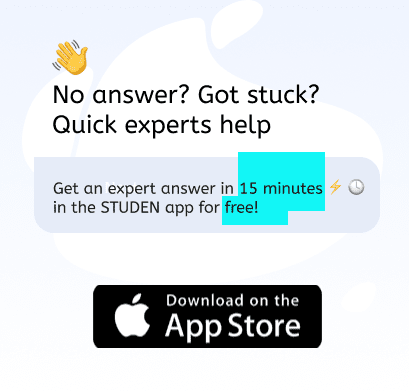
Another question on Computers and Technology

Computers and Technology, 22.06.2019 03:10
Write a program that begins by reading in a series of positive integers on a single line of input and then computes and prints the product of those integers. integers are accepted and multiplied until the user enters an integer less than 1. this final number is not part of the product. then, the program prints the product. if the first entered number is negative or 0, the program must print “bad input.” and terminate immediately. next, the program determines and prints the prime factorization of the product, listing the factors in increasing order. if a prime number is not a factor of the product, then it must not appear in the factorization. sample runs are given below. note that if the power of a prime is 1, then that 1 must appear in t
Answers: 3

Computers and Technology, 22.06.2019 15:50
The file sales data.xlsx contains monthly sales amounts for 40 sales regions. write a sub that uses a for loop to color the interior of every other row (rows 3, 5, etc.) gray. color only the data area, columns a to m. (check the file colors in excel.xlsm to find a nice color of gray.)
Answers: 2

Computers and Technology, 23.06.2019 01:20
Me with this program in c++ ! computers represent color by combining sub-colors red, green, and blue (rgb). each sub-color's value can range from 0 to 255. thus (255, 0, 0) is bright red. (130, 0, 130) is a medium purple. (0, 0, 0) is black, (255, 255, 255) is white, and (40, 40, 40) is a dark gray. (130, 50, 130) is a faded purple, due to the (50, 50, 50) gray part. (in other word, equal amounts of red, green, blue yield gray).given values for red, green, and blue, remove the gray part. ex: if the input is 130 50 130, the output is: 80 0 80. thus, find the smallest value, and then subtract it from all three values, thus removing the gray.
Answers: 3

Computers and Technology, 23.06.2019 14:30
Select the correct answer. sean is a computer programmer. he has programmed an application for toddlers that plays nursery rhymes. however, a logic error has occurred in the program. which problem is a likely consequence of the error? a. the program crashes every time the user wants to play the nursery rhymes. b. the program crosses its buffer boundaries and overwrites an adjacent program. c. the program plays a different nursery rhyme than the one the user intended to play. d. the program shows different structures in its programming language code. e. the program introduces new viruses every time the user plays a nursery rhyme.
Answers: 1
You know the right answer?
I wrote the move function of this phython code:
but it is not working here correctly, Karel is only...
Questions

Geography, 07.12.2020 16:10

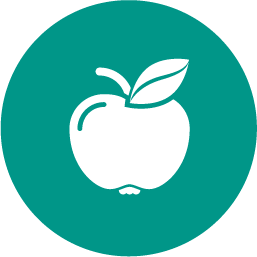
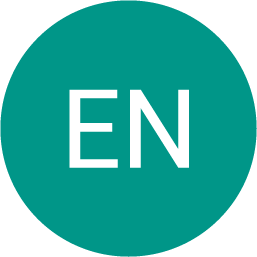

Mathematics, 07.12.2020 16:10
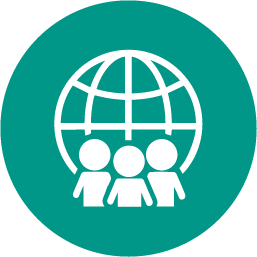
Social Studies, 07.12.2020 16:10

Mathematics, 07.12.2020 16:10
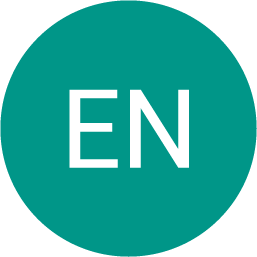

Mathematics, 07.12.2020 16:10
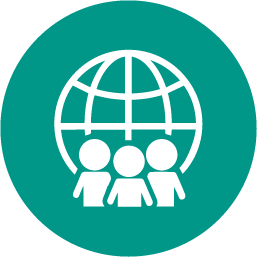
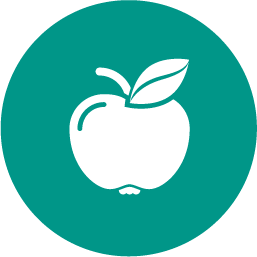
Physics, 07.12.2020 16:10


Mathematics, 07.12.2020 16:10
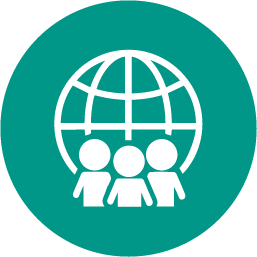

Biology, 07.12.2020 16:10

Mathematics, 07.12.2020 16:10

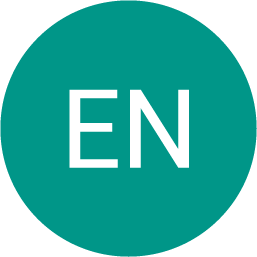
English, 07.12.2020 16:10

Business, 07.12.2020 16:10