
Computers and Technology, 09.02.2021 19:10 emmaea831
Part A
One of the biggest benefits of writing code inside functions is that we can reuse the code. We simply call it whenever we need it!
Let’s take a look at a calculator program that could be rewritten in a more reusable way with functions. Notice that two floats (decimal numbers, but they can also include integers) are inputted by the user, as an operation that the user would like to do. A series of if statements are used to determine what operation the user has chosen, and then, the answer is printed inside a formatted print statement.
num1 = float(input("Enter your first number: "))
num2 = float(input("Enter your second number: "))
operation = input("What operation would you like to do? Type add, subtract, multiply, or divide.")
if operation == "add":
print(num1, "+", num2,"=", num1 + num2)
elif operation == "subtract":
print(num1, "-", num2,"=", num1 - num2)
elif operation == "multiply":
print(num1, "*", num2,"=", num1 * num2)
elif operation == "divide":
print(num1, "/", num2,"=", num1 / num2)
else:
print("Not a valid operation.")
Your job is to rewrite the program using functions. We have already looked at a function that adds two numbers. Using that as a starting point, we could call the add function from within our program in this way:
if operation == “add”:
result = add(num1, num2)
print(num1, "+", num2,"=",result)
Now it’s your turn to do the following:
Type all of the original code into a new file in REPL. it.
Copy the add function from the unit and paste it at the top of your program.
Write 3 additional functions: subtract, multiply, and divide. Pay careful attention to the parameters and return statement. Remember to put the three functions at the top of your Python program before your main code.
Rewrite the main code so that your functions are called.

Answers: 2
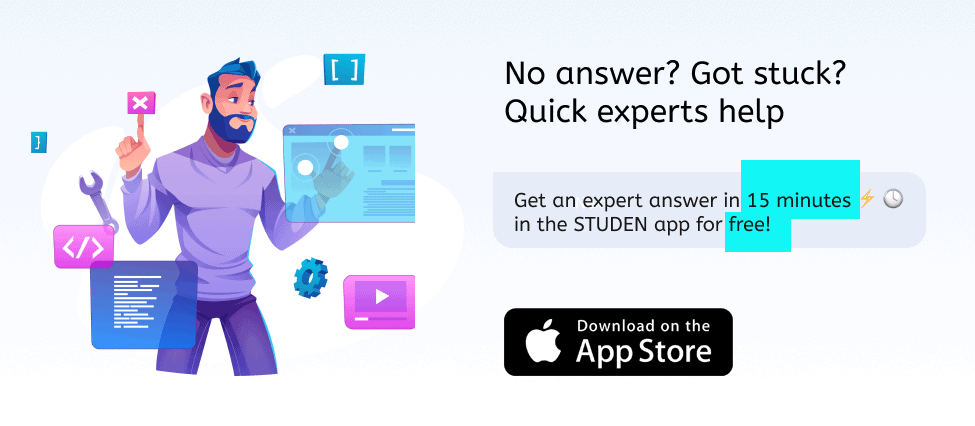
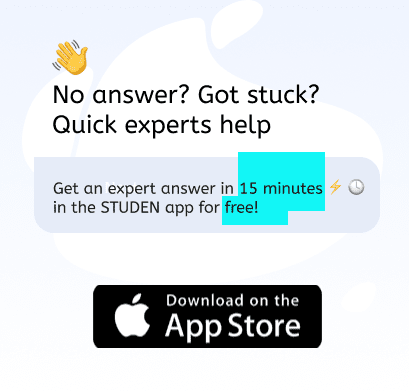
Another question on Computers and Technology

Computers and Technology, 22.06.2019 22:30
Who needs to approve a change before it is initiated? (select two.) -change board -client or end user -ceo -personnel manager -project manager
Answers: 1

Computers and Technology, 23.06.2019 05:20
What did creator markus “notch" persson initially call his game
Answers: 1

Computers and Technology, 23.06.2019 18:30
Write a program that prints the day number of the year, given the date in the form month-day-year. for example, if the input is 1-1-2006, the day number is 1; if the input is 12-25-2006, the day number is 359. the program should check for a leap year. a year is a leap year if it is divisible by 4, but not divisible by 100. for example, 1992 and 2008 are divisible by 4, but not by 100. a year that is divisible by 100 is a leap year if it is also divisible by 400. for example, 1600 and 2000 are divisible by 400. however, 1800 is not a leap year because 1800 is not divisible by 400.
Answers: 3

Computers and Technology, 23.06.2019 20:40
Instruction active describing list features which statements accurately describe the features of word that are used to create lists? check all that apply. the tab key can be used to create a sublist. the enter key can be used to add an item to a list. the numbering feature allows for the use of letters in a list. the numbering feature can change the numbers to bullets in a list. the multilevel list feature provides options for different levels in a list.
Answers: 2
You know the right answer?
Part A
One of the biggest benefits of writing code inside functions is that we can reuse the code....
Questions
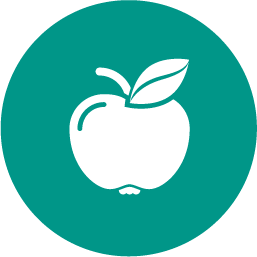
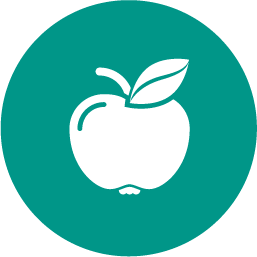
Physics, 04.02.2021 05:10

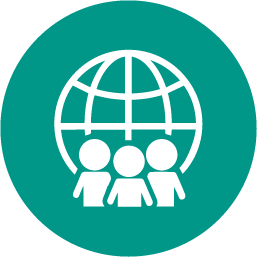

Mathematics, 04.02.2021 05:10


Medicine, 04.02.2021 05:10

Biology, 04.02.2021 05:10

Biology, 04.02.2021 05:10
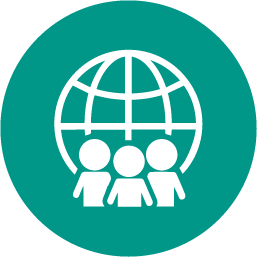
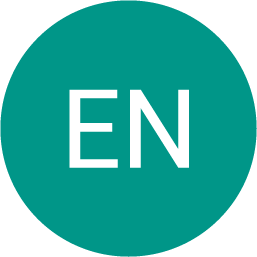




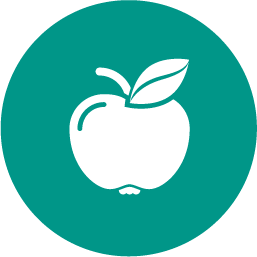


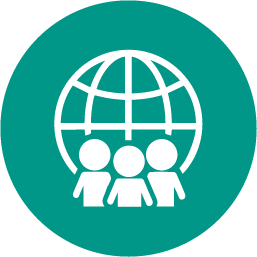