Does somebody know how to this. This is what I got so far
import java. io.*;
import java. uti...

Computers and Technology, 10.02.2021 21:20 kikiwaka1
Does somebody know how to this. This is what I got so far
import java. io.*;
import java. util. Scanner;
public class Lab33bst
{
public static void main (String args[]) throws IOException
{
Scanner input = new Scanner(System. in);
System. out. print("Enter the degree of the polynomial --> ");
int degree = input. nextInt();
System. out. println();
PolyNode p = null;
PolyNode temp = null;
PolyNode front = null;
System. out. print("Enter the coefficent x^" + degree + " if no term exist, enter 0 --> ");
int coefficent = input. nextInt();
front = new PolyNode(coefficent, degree, null);
temp = front;
int tempDegree = degree;
//System. out. println(front. getCoeff() + " " + front. getDegree());
for (int k = 1; k <= degree; k++)
{
tempDegree--;
System. out. print("Enter the coefficent x^" + tempDegree + " if no term exist, enter 0 --> ");
coefficent = input. nextInt();
p = new PolyNode(coefficent, tempDegree, null);
temp. setNext(p);
temp = p;
}
System. out. println();
p = front;
while (p != null)
{
System. out. println(p. getCoeff() + "^" + p. getDegree() + "+" );
p = p. getNext();
}
System. out. println();
}
}
class PolyNode
{
private int coeff; // coefficient of each term
private int degree; // degree of each term
private PolyNode next; // link to the next term node
public PolyNode (int c, int d, PolyNode initNext)
{
coeff = c;
degree = d;
next = initNext;
}
public int getCoeff()
{
return coeff;
}
public int getDegree()
{
return degree;
}
public PolyNode getNext()
{
return next;
}
public void setCoeff (int newCoeff)
{
coeff = newCoeff;
}
public void setDegree (int newDegree)
{
degree = newDegree;
}
public void setNext (PolyNode newNext)
{
next = newNext;
}
}
This is the instructions for the lab. Somebody please help. I need to complete this or I'm going fail the class please help me.
Write a program that will evaluate polynomial functions of the following type:
Y = a1Xn + a2Xn-1 + a3Xn-2 + . . . an-1X2 + anX1 + a0X0 where X, the coefficients ai, and n are to be given.
This program has to be written, such that each term of the polynomial is stored in a linked list node.
You are expected to create nodes for each polynomial term and store the term information. These nodes need to be linked to each previously created node. The result is that the linked list will access in a LIFO sequence. When you display the polynomial, it will be displayed in reverse order from the keyboard entry sequence.
Make the display follow mathematical conventions and do not display terms with zero coefficients, nor powers of 1 or 0. For example the polynomial Y = 1X^0 + 0X^1 + 0X^2 + 1X^3 is not concerned with normal mathematical appearance, don’t display it like that. It is shown again as it should appear. Y = 1 + X^3
Normal polynomials should work with real number coefficients. For the sake of this program, assume that you are strictly dealing with integers and that the result of the polynomial is an integer as well. You will be provided with a special PolyNode class. The PolyNode class is very similar to the ListNode class that you learned about in chapter 33 and in class. The ListNode class is more general and works with object data members. Such a class is very practical for many different situations. For this assignment, early in your linked list learning, a class has been created strictly for working with a linked list that will store the coefficient and the degree of each term in the polynomial.
class PolyNode
{
private int coeff; // coefficient of each term
private int degree; // degree of each term
private PolyNode next; // link to the next term node
public PolyNode (int c, int d, PolyNode initNext)
{
coeff = c;
degree = d;
next = initNext;
}
public int getCoeff()
{
return coeff;
}
public int getDegree()
{
return degree;
}
public PolyNode getNext()
{
return next;
}
public void setCoeff (int newCoeff)
{
coeff = newCoeff;
}
public void setDegree (int newDegree)
{
degree = newDegree;
}
public void setNext (PolyNode newNext)
{
next = newNext;
}
}
You are expected to add various methods that are not provided in the student version. The sample execution will indicate which methods you need to write. Everything could be finished in the main method of the program, but hopefully you realize by now that such an approach is rather poor program design.

Answers: 2
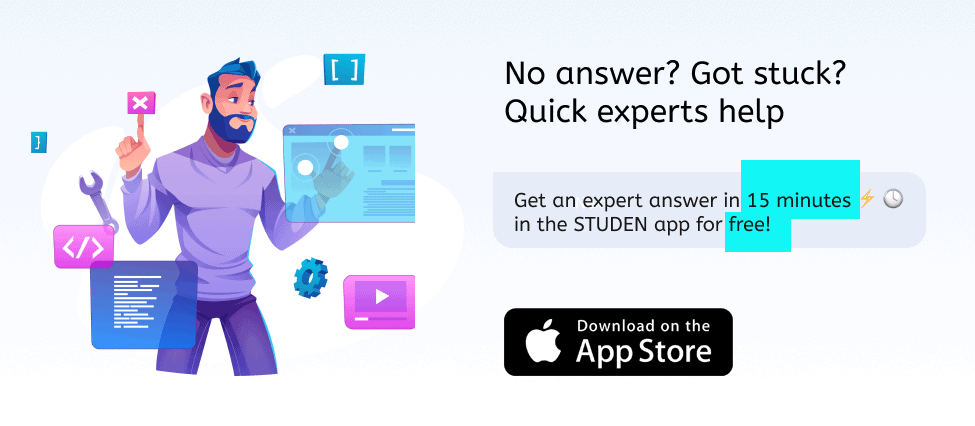
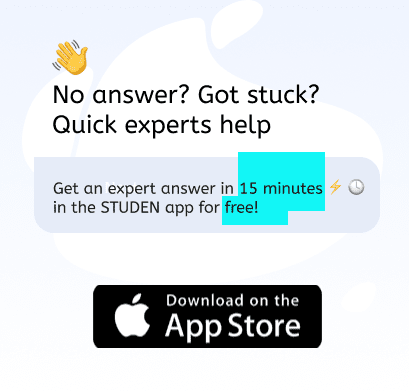
Another question on Computers and Technology

Computers and Technology, 23.06.2019 22:30
Lakendra finished working on her monthly report. in looking it over, she saw that it had large blocks of white space. what steps could lakendra take to reduce the amount of white space?
Answers: 3

Computers and Technology, 23.06.2019 23:30
A. in packet tracer, only the server-pt device can act as a server. desktop or laptop pcs cannot act as a server. based on your studies so far, explain the client-server model.
Answers: 2

Computers and Technology, 24.06.2019 17:30
What is the next step if your volume does not work on computer
Answers: 2

Computers and Technology, 24.06.2019 17:40
The value of sin(x) (in radians) can be approximated by the alternating infinite series create a function (prob3_2) that takes inputs of a scalar angle measure (in radians) and the number of approximation terms, n, and estimates sin(x). do not use the sin function in your solution. you may use the factorial function. though this can be done without a loop (more efficiently), your program must use (at least) one. you may find the mod() function useful in solving the problem.
Answers: 1
You know the right answer?
Questions
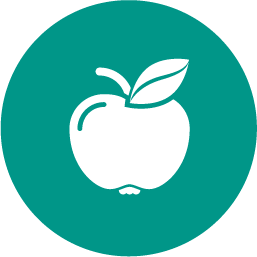

Mathematics, 24.09.2019 00:00


Mathematics, 24.09.2019 00:00
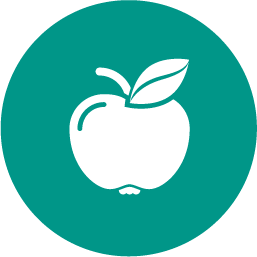
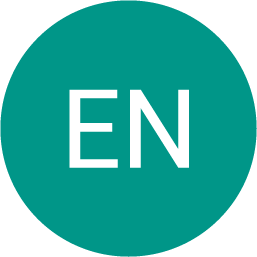



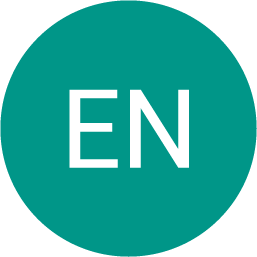
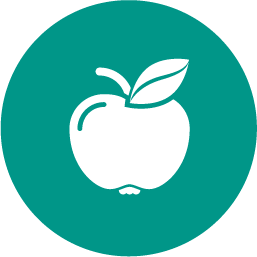
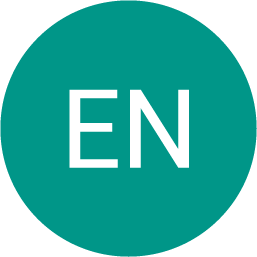
English, 24.09.2019 00:00

Computers and Technology, 24.09.2019 00:00

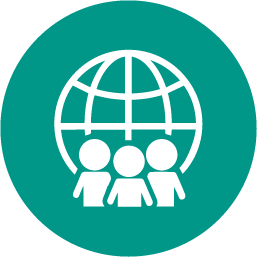
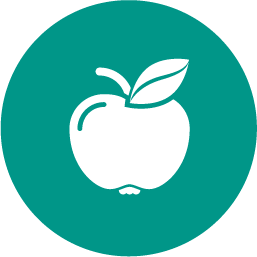

Mathematics, 24.09.2019 00:00
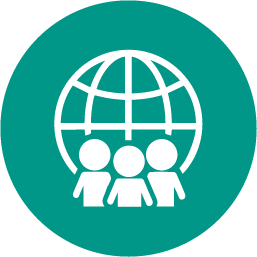
Social Studies, 24.09.2019 00:00
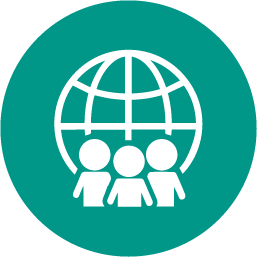

Biology, 24.09.2019 00:00