
Computers and Technology, 08.03.2021 20:00 buky0910p6db44
1. Create a Java program.
2. The class name for the program should be 'EncryptTextMethods'.
3. In the program you should perform the following:
* You will modify the EncryptText program created in a previous assignment (see code below).
* You should move the logic for encrypting text from the main method into a method named encryptString.
* The encryptString method should have a single String argument and return a String value.
1. Create a second method.
2. The name of the method should be decryptString.
3. In the program you should perform the following:
* The method should have a single String argument and return a String value.
* This method should reverse the encryption process by subtracting one from each character in the String argument passed to it.
* The return value should be the result of the decrypting process.
4.The program should read an input String using the Scanner method.
5. The input value should be passed to the encryptString() method and the encrypted string should be passed to the decryptString() method.
6. The program should output the input string, the encrypted string and the decrypted string.
// Existing Code
import java. util. Scanner;
public class EncryptText {
private static String encodeMessage(String message) {
//convert string to character array
char stringChars[] = message. toCharArray();
//for each character
for(int i=0; i< stringChars. length; i++) {
char ch = stringChars[i];
//if character within range of alphabet
if(ch <= 'z' && ch >= 'a') {
//obtain the character value
//add 1 to the integer value
//enter modulus by 26 since z will become a
//once again add the remainder to 'a'
stringChars[i] = (char)('a' + ((int)(ch - 'a') + 1) % 26 );
}
}
//construct the string message
return String. valueOf(stringChars);
}
public static void main(String[] args) {
Scanner keyboard = new Scanner(System. in);
System. out. println("Please enter text to encode: ");
String message = keyboard. nextLine();
message = message. toLowerCase();
System. out. println("Encoded: " + encodeMessage(message));
keyboard. close();
}
}

Answers: 2
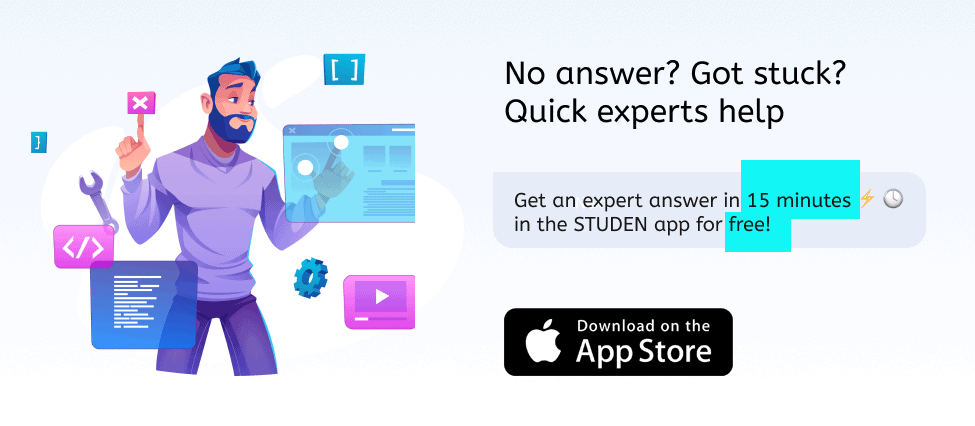
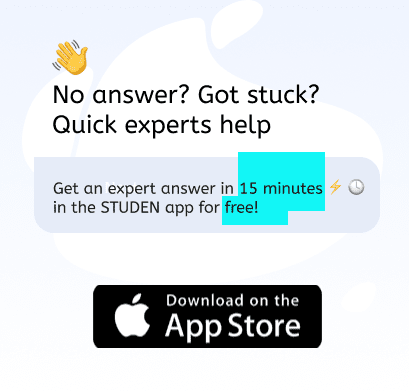
Another question on Computers and Technology

Computers and Technology, 22.06.2019 18:00
What is the first view you place in your drawing?
Answers: 1

Computers and Technology, 22.06.2019 18:30
All of the following are characteristics that must be contained in any knowledge representation scheme except
Answers: 3

Computers and Technology, 23.06.2019 18:50
What is transmission control protocol/internet protocol (tcp/ip)? software that prevents direct communication between a sending and receiving computer and is used to monitor packets for security reasons a standard that specifies the format of data as well as the rules to be followed during transmission a simple network protocol that allows the transfer of files between two computers on the internet a standard internet protocol that provides the technical foundation for the public internet as well as for large numbers of private networks
Answers: 2

Computers and Technology, 24.06.2019 02:10
Which sentences describe the things you need to ensure while creating a sketch and a drawing? while an artistic or creative drawing is a creative expression, a technical drawing is an informative expression. you need to create accurate and neat drawings to convey accurate information. a technical drawing clearly conveys its meaning or information, and does not leave room for interpretation maintain a good speed while creating drawings
Answers: 1
You know the right answer?
1. Create a Java program.
2. The class name for the program should be 'EncryptTextMethods'.
3...
3...
Questions

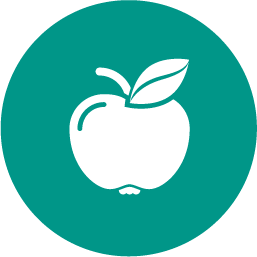



Mathematics, 02.07.2020 23:01

Business, 02.07.2020 23:01

Mathematics, 02.07.2020 23:01
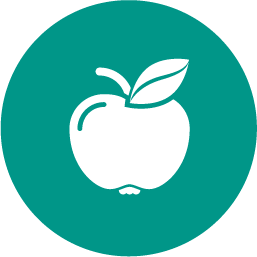


Mathematics, 02.07.2020 23:01
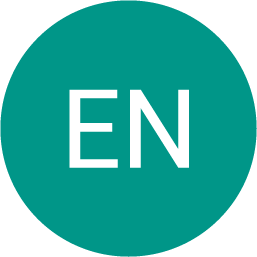


Health, 02.07.2020 23:01

Mathematics, 02.07.2020 23:01

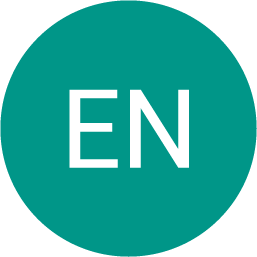
English, 02.07.2020 23:01

Mathematics, 02.07.2020 23:01
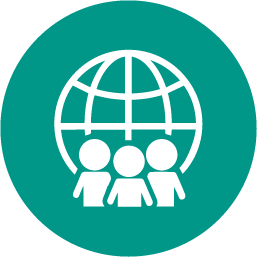
History, 02.07.2020 23:01
