
Computers and Technology, 04.05.2021 19:30 cia196785920
File Factorials contains a program that calls the factorial method of the MathUtils class to compute the factorials of integers entered by the user. Save these files to your directory and study the code in both, then compile and run Factorials to see how it works. Try several positive integers, then try a negative number. You should find that it works for small positive integers (values < 17), but that it returns a large negative value for larger integers and that it always returns 1 for negative integers.
Create a custom exception class called myException. This class should take in one String parameter that can be passed to the super class inside the constructor
Returning 1 as the factorial of any negative integer is not correct—mathematically, the factorial function is not defined for negative integers. To correct this, you could modify your factorial method to check if the argument is negative, but then what? The method must return a value, and even if it prints an error message, whatever value is returned could be misconstrued. Instead it should throw an exception indicating that something went wrong so it could not complete its calculation. Use your myException class to handle the situation. Modify your program as follows:
Modify the header of the factorial method to indicate that factorial can throw a myException.
Modify the body of factorial to check the value of the argument and, if it is negative, throw a myException. Note that what you pass to throw is actually an instance of the myException class, and that the constructor takes a String parameter. Use this parameter to be specific about what the problem is.
Compile and run your Factorials program after making these changes. Now when you enter a negative number an exception will be thrown, terminating the program. The program ends because the exception is not caught, so it is thrown by the main method, causing a runtime error.
Modify the main method in your Factorials class to catch the exception thrown by factorial and print an appropriate message, but then continue with the loop. Think carefully about where you will need to put the try and catch.
Returning a negative number for values over 16 also is not correct. The problem is arithmetic overflow—the factorial is bigger than can be represented by an int. This can also be thought of as a myException — this factorial method is only defined for arguments up to 16. Modify your code in factorial to check for an argument over 16 as well as for a negative argument. You should throw a myException in either case, but pass different messages to the constructor so that the problem is clear.
Submit the following:
Modified MathUtils. java
Modified Factorials. java
myException. java
//
// Factorials. java
//
// Reads integers from the user and prints the factorial of each.
//
//
import java. util. Scanner;
public class Factorials
{
public static void main(String[] args)
{
String keepGoing = "y";
Scanner scan = new Scanner(System. in);
while (keepGoing. equals("y") || keepGoing. equals("Y"))
{
System. out. print("Enter an integer: ");
int val = scan. nextInt();
System. out. println("Factorial(" + val + ") = "
+ MathUtils. factorial(val));
System. out. print("Another factorial? (y/n) ");
keepGoing = scan. next();
}
}
}
//
// MathUtils. java
//
// Provides static mathematical utility functions.
//
//
public class MathUtils
{
//
// Returns the factorial of the argument given
//
public static int factorial(int n)
{
int fac = 1;
for (int i=n; i>0; i--)
fac *= i;
return fac;
}
}

Answers: 2
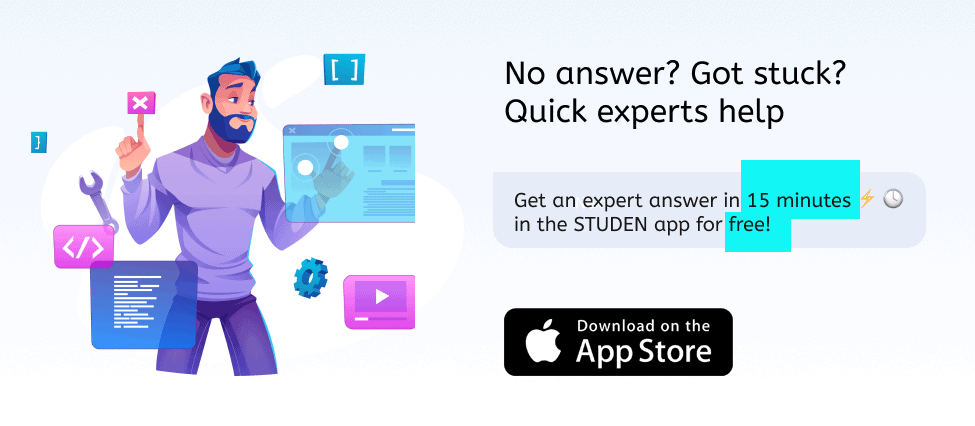
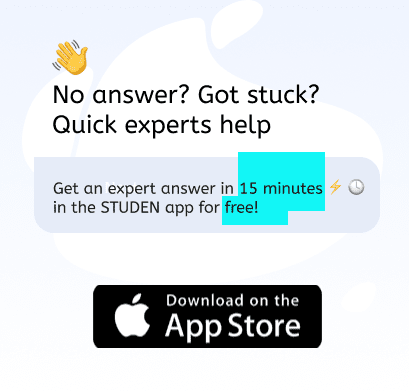
Another question on Computers and Technology

Computers and Technology, 22.06.2019 23:30
To check spelling errors in a document, the word application uses the to determine appropriate spelling. internet built-in dictionary user-defined words other text in the document
Answers: 1

Computers and Technology, 23.06.2019 00:40
Consider the following statements: struct nametype{string first; string last; }; struct coursetype{string name; int callnum; int credits; char grade; }; struct studenttype{nametype name; double gpa; coursetype course; }; studenttype student; studenttype classlist[100]; coursetype course; nametype name; mark the following statements as valid or invalid. if a statement is invalid, explain why.a.) student.course.callnum = "csc230"; b.) cin > > student.name; c.) classlist[0] = name; d.) classlist[1].gpa = 3.45; e.) name = classlist[15].name; f.) student.name = name; g.) cout < < classlist[10] < < endl; h.) for (int j = 0; j < 100; j++)classlist[j].name = name; i.) classlist.course.credits = 3; j.) course = studenttype.course;
Answers: 1

Computers and Technology, 23.06.2019 20:30
If chris has a car liability insurance, what damage would he be covered for
Answers: 1

Computers and Technology, 23.06.2019 20:50
3.11.3 quiz: comparing and analyzing function typesquestion 4 of 102 pointswhat can you say about the y-values of the two functions f(x) = 3x2-3 andg(x)=2* - 3?
Answers: 2
You know the right answer?
File Factorials contains a program that calls the factorial method of the MathUtils class to compute...
Questions
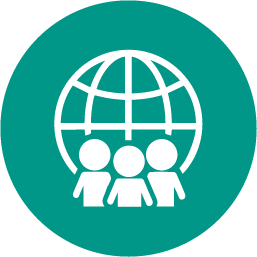


Mathematics, 29.10.2020 05:40


Mathematics, 29.10.2020 05:40

Mathematics, 29.10.2020 05:40
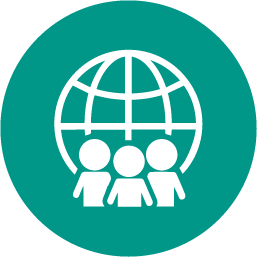
History, 29.10.2020 05:40
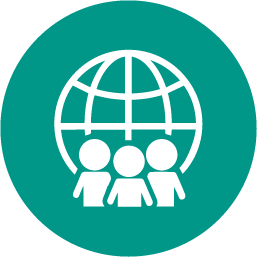

Arts, 29.10.2020 05:40


Mathematics, 29.10.2020 05:40

Mathematics, 29.10.2020 05:40

Biology, 29.10.2020 05:40
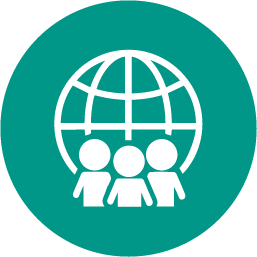
Social Studies, 29.10.2020 05:40


Mathematics, 29.10.2020 05:40
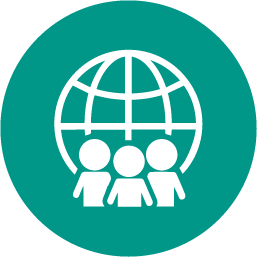

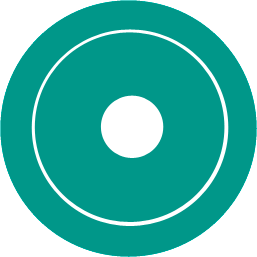
Advanced Placement (AP), 29.10.2020 05:40

Mathematics, 29.10.2020 05:40