
Computers and Technology, 05.05.2021 15:50 Nicoleebel2984
Modify the classes related to circles to demonstrate polymorphism. 1. Create a new project called 09.03 Assignment in the Module 09 Assignments folder. 2. Download the Java files to the newly-created project folder. o Circle2.java o Cylinder2.java o Oval2.java o OvalCylinder2.java 3. Update each circle implementation class to include a getName method that will return the name of the circle. For instance, the Circle2 class should return just Circle. 4. Create a new client class that will be used to test the circle classes. Be sure to update the heading to include the program's purpose, your name, and today's date. 5. In the main method, declare and initialize at least one new instance for each of the circle classes. 6. Use an ArrayList to better organize the circles. Add each of the new objects to the ArrayList. 7. In the tester program, create a static method named showCenter. This method will accept as a parameter any object related to a circle. The method will output the result of calling the getCenter method that all the circle classes have in common. See the sample output below. 8. In the main method, test the showCenter method on each circle object stored in the ArrayList. Expected Output: When your program runs correctly, the output should resemble the following: Bluel: Terminal Window Ρ
Options For this Circle2 the center is at (2, 4). For this Cylinder2 the center is at (10, 15). For this Oval2 the center is at (25, 10). For this OvalCylinder2 the center is at (40, 10). 09.03 Polymorphism Grading Rubric Points Possible Points Earned Components Comments include name, date, and purpose of the program. 1 Tester class correctly created. 1 ArrayList with new objects of each circle declared. 2 The showCenter method properly tested using ArrayList of objects, loop, and getName. 2. The showCenter method header defined with correct parameter list and return type. 2 The showCenter method properly implemented to display output. 3 The getName method added to each implementation class. 1 No compiler errors. No runtime errors. Output is accurate. 2 Thoughtful PMR included. 1 Total 15
Circle2:
public class Circle2
{
// instance variables
private int x;
private int y;
private int radius;
// Constructor for objects of class Circle
public Circle2(int p_x, int p_y, int r)
{
// initialize instance variables
x = p_x;
y = p_y;
radius = r;
}
public int getRadius()
{
return radius;
}
// Concatenates a String to show the center x, y point of the circle
public String getCenter()
{
return "center is at (" + x + ", " + y + ")";
}
}
Cyclinder2:
public class Cylinder2 extends Circle2
{
// instance variables
private int height;
// Constructor for objects of class Cylinder
public Cylinder2(int x, int y, int rad, int h)
{
// call superclass
super(x, y, rad);
// initialize instance variables
height = h;
}
public int getHeight()
{
return height;
}
}
Oval2:
public class Oval2 extends Circle2
{
// instance variables
private int radius2;
// Constructor for objects of class Oval
public Oval2(int x, int y, int rad1, int rad2)
{
// call superclass
super(x, y, rad1);
// initialize instance variables
radius2 = rad2;
}
public int getRadius2()
{
return radius2;
}
}
OvalCylinder2:
public class OvalCylinder2 extends Oval2
{
// instance variables
private int height;
// Constructor for objects of class OvalCylinder
public OvalCylinder2(int x, int y, int rad1, int rad2, int h)
{
// call superclass
super(x, y, rad1, rad2);
// initialize instance variables
height = h;
}
public int getHeight()
{
return height;
}
}

Answers: 2
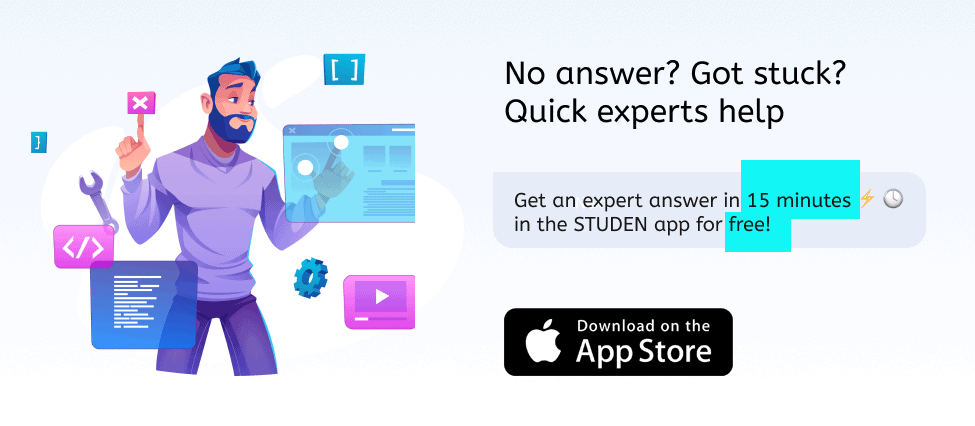
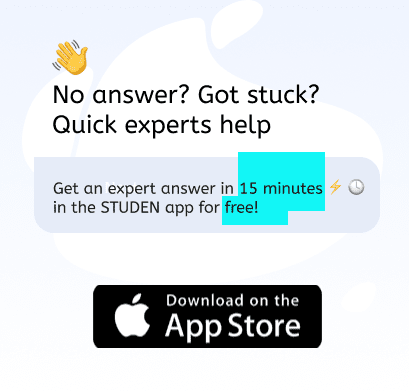
Another question on Computers and Technology

Computers and Technology, 23.06.2019 12:00
What type of slide show is a dynamic and eye-catching way to familiarize potential customers with what your company has to offer? a. ole b. photo album c. brochure d. office clipboard
Answers: 2

Computers and Technology, 23.06.2019 18:40
How does is make you feel when you're kind to others? what are some opportunities in your life to be more kind to your friends and loved ones? imagine a world where kindness has be outlawed. how would people act differently? would your day-to-day life change significantly? why or why not?
Answers: 2

Computers and Technology, 24.06.2019 10:00
Each time you save a document, you will need to type in the file type in which it should be saved you can select the save button to save it with the same file name if it has been previously saved you will need to select the location to save the file you will need to use the save as dialog box
Answers: 1

Computers and Technology, 24.06.2019 13:20
In the insert table dialog box, you select the checkbox to create the first row as the header of the table.
Answers: 3
You know the right answer?
Modify the classes related to circles to demonstrate polymorphism. 1. Create a new project called 09...
Questions

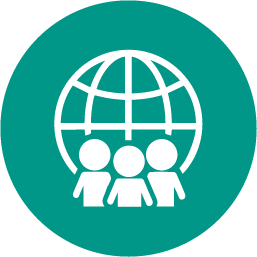
Social Studies, 18.04.2020 21:22

Mathematics, 18.04.2020 21:22
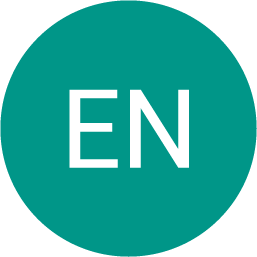
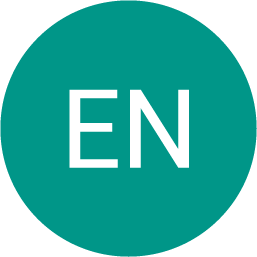

Chemistry, 18.04.2020 21:22




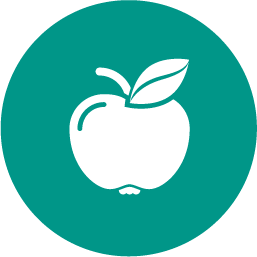
Physics, 18.04.2020 21:22

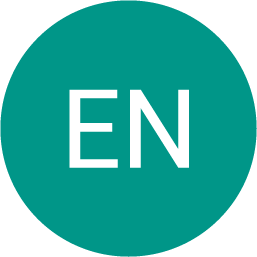



Mathematics, 18.04.2020 21:23

Mathematics, 18.04.2020 21:23

Mathematics, 18.04.2020 21:23

Mathematics, 18.04.2020 21:23
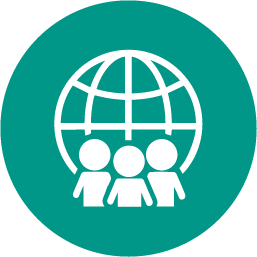
Social Studies, 18.04.2020 21:23