
Computers and Technology, 05.05.2021 21:10 coolaadhya123
In the BinarySearchTree class, write the new method: private E deletePredecessor(BSTNode where)
This method should find and delete the in-order predecessor of where, returning the value that was deleted. If where itself is null, or if where doesn’t have a predecessor (i. e., the left subtree doesn’t exist), the method should return null. The method is declared private since it’s meant to be called only from another method of BinarySearchTree, to be written later.
In the BinarySearchTree class, write the new method: private E deleteSuccessor(BSTNode where)
This method should find and delete the in-order successor of where, returning the value that was deleted. If where itself is null, or if where doesn’t have a successor (i. e., the right subtree doesn’t exist), the method should return null. The method is declared private since it’s meant to be called only from another method of BinarySearchTree, to be written later.
In the BinarySearchTree class, write the new method: public E remove(E item)
This method should find and delete the item from the BST, returning the value that was re- moved. If the item is not found in the tree, the method should leave the tree unchanged and return null. Use the pseudocode in Figure 9.6.1 of the zyBook as a starting point. However, when removing a node with two children, your code should randomly select whether to use the in-order predecessor or successor in the delete algorithm. This will prevent the tree from get- ting too unbalanced. Call your previously written deletePredecessor and deleteSuccessormethods as needed.
In the BinarySearchTree class, write a main method that tests your removemethod. Be sure to test at least four cases:
• Removing a node with no children
• Removing a node with only a left child
• Removing a node with only a right child
• Removing a node with two children
BinarySearchTree
public class BinarySearchTree< E extends Comparable> {
// Maintains the root (first node) of the tree
private BSTNode root;
// Adds the newValue to the BST
// This method also prevents duplicate values from being added to the BST.
public void add(E newValue) {
// Create a new BSTNode that contains the newValue
BSTNode newNode = new BSTNode<>(newValue, null, null);
// If tree is empty, the newNode should become the root
if (root == null)
root = newNode;
else {
BSTNode currentNode = root;
while (currentNode != null) {
// Compare the newValue vs. the data in the currentNode
int compareResult = newValue. compareTo(currentNode. getData());
// newValue is "less than" the current node - go left
if (compareResult < 0) {
// If there is no left child for currentNode, make newNode
// the left child of currentNode
if (currentNode. getLeft() == null) {
currentNode. setLeft(newNode);
currentNode = null;
}
// If there *is* a left child for currentNode, just move
// currentNode down the left subtree
else
currentNode = currentNode. getLeft();
}
// newValue is "greater than" the current node - go right
else if (compareResult > 0) {
// If there is no right child for currentNode, make newNode
// the right child of currentNode
if (currentNode. getRight() == null) {
currentNode. setRight(newNode);
currentNode = null;
}
// If there *is* a right child for currentNode, just move
// currentNode down the right subtree
else
currentNode = currentNode. getRight();
}
// newValue is "equal to" the current node - exit the loop without adding newValue
else
currentNode = null;
}
}
}

Answers: 3
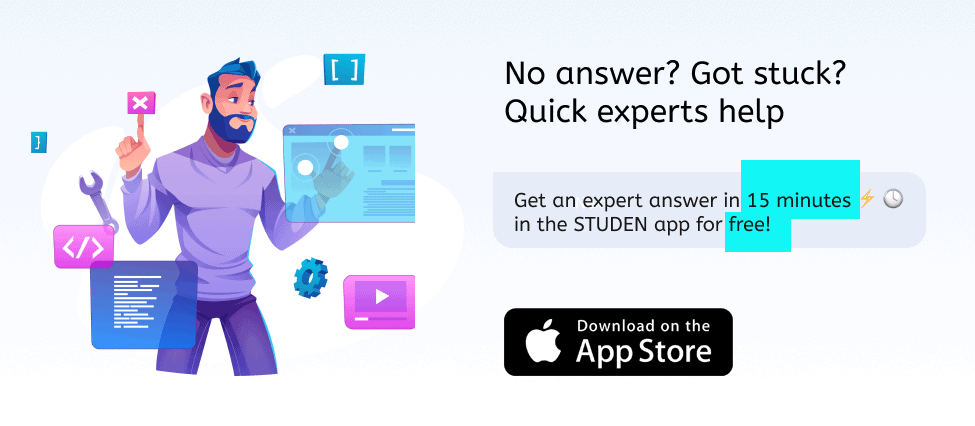
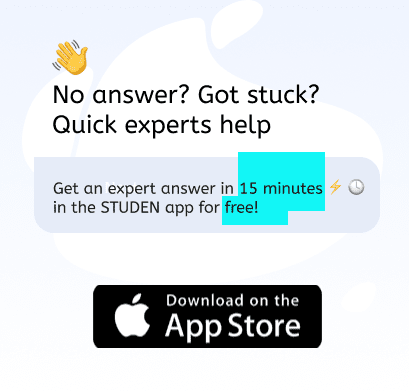
Another question on Computers and Technology

Computers and Technology, 22.06.2019 12:50
You have just been hired as an information security engineer for a large, multi-international corporation. unfortunately, your company has suffered multiple security breaches that have threatened customers' trust in the fact that their confidential data and financial assets are private and secured. credit-card information was compromised by an attack that infiltrated the network through a vulnerable wireless connection within the organization. the other breach was an inside job where personal data was stolen because of weak access-control policies within the organization that allowed an unauthorized individual access to valuable data. your job is to develop a risk-management policy that addresses the two security breaches and how to mitigate these risks.requirementswrite a brief description of the case study. it requires two to three pages, based upon the apa style of writing. use transition words; a thesis statement; an introduction, body, and conclusion; and a reference page with at least two references. use a double-spaced, arial font, size 12.
Answers: 1

Computers and Technology, 22.06.2019 14:50
Drag each label to the correct location on the image list the do’s and don’ts of safeguarding your password. a. keep yourself logged in when you leave your computer.b. don’t write your password down and leave it where others can find it.c. share your password with your friends.d.each time you visit a website,retain the cookies on your computer.e. use a long password with mixed characters.1. do's 2. don'ts
Answers: 2

Computers and Technology, 22.06.2019 16:00
If a client wants to make minor edits, what should he/she use?
Answers: 3

Computers and Technology, 23.06.2019 09:20
How to print: number is equal to: 1 and it is odd number number is equal to: 2 and it is even number number is equal to: 3 and it is odd number number is equal to: 4 and it is even number in the console using java using 1 if statement, 1 while loop, 1 else loop also using % to check odds and evens
Answers: 3
You know the right answer?
In the BinarySearchTree class, write the new method: private E deletePredecessor(BSTNode where)
Th...
Questions


Mathematics, 04.06.2021 23:20
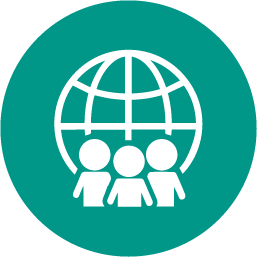

Mathematics, 04.06.2021 23:20


Mathematics, 04.06.2021 23:20
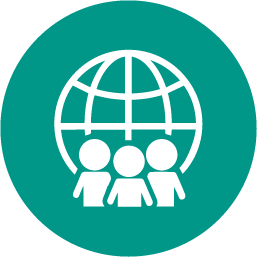


Chemistry, 04.06.2021 23:20
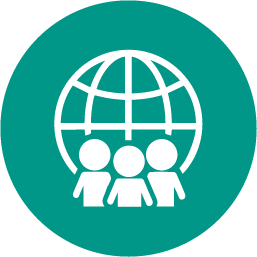


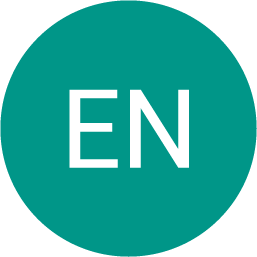

Mathematics, 04.06.2021 23:20
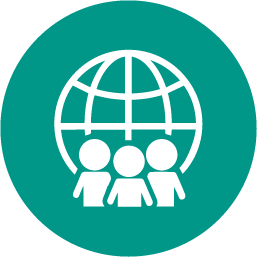


Mathematics, 04.06.2021 23:20

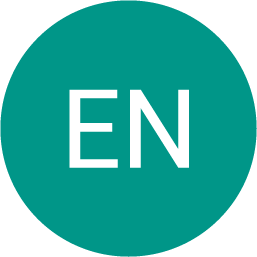
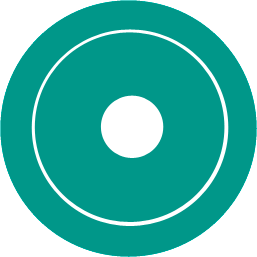
Advanced Placement (AP), 04.06.2021 23:20