
Computers and Technology, 13.05.2021 23:40 ava1018
Write a program that simulates flipping a coin and rolling a dice.
A user will input their choice of flipping a coin (C), rolling a dice (D), or Exiting(E).
If the user chooses a coin toss, the program will ask how many times the coin should be tossed, then will simulate tossing the coin that many times and print the result to the user.
If the user chooses rolling a die, the program will ask how many sides the die has and h ow many times it should be rolled. The program will then simulate rolling a die (with the number of sides specified) that many times.
The program will continue until the user presses E.
This should be a lot of fun!
Here are some great things to think about as you begin your program!
You will need two functions. A string flipCoin() and a int rollDice(int) function. These functions will generate a random number. For the coin flip the random number should be in the range of 1 to 2. If the number is 1 the function should return "heads". If the random number is 2, the function should return "tails". For the roll dice function, the random number should be in the range of 1 to the number of sides. The function should return the result of the die roll.
Important notes: You will want to seed your random number generator. To do this at the beginning of your program #include
Use the following commands to seed the random number generator:
//Get the system time to use it to seed the random number generator
unsigned seed = time(0);
//seed the random number generator
srand(seed);
to get a random number between one and 2 use the following code: int toss = 1 + rand() % 2;
Sample Output from the Program
Welcome to the Random Value Generator!
Would you like to flip a coin (C) or roll a die (D) or Exit (E): C
How many times do you want to flip the coin: 5
flip 1 : tails
flip 2 : heads
flip 3 : heads
flip 4 : heads
flip 5 : tails
Would you like to flip a coin (C) or roll a die (D) or Exit (E): D
How many sides does your die have: 6
How many times do you want to roll the die: 5
roll 1 : 5
roll 2 : 3
roll 3 : 2
roll 4 : 2
roll 5 : 2
Would you like to flip a coin (C) or roll a die (D) or Exit (E): D
How many sides does your die have: 20
How many times do you want to roll the die: 3
roll 1 : 19
roll 2 : 16
roll 3 : 15
Would you like to flip a coin (C) or roll a die (D) or Exit (E): E
Thanks for playing!
Press any key to continue . . .
Step 2: Processing Logic
Using the pseudocode below, write the code that will meet the requirements.
Main Function
Declare the number of tosses, number of sides, and user choice.
Seed the random number generator
while user choice !=E
Ask the user if they want to flip a coin, roll a die, or exit
If the choice is C
Ask the user how many times to flip the coin.
For i=1 to number of flips step 1
Call flipCoin()
Print result
If the choice is D
Ask the user how many sides the die has.
Ask the user how many times to roll the die
For i=1 to number of rolls step 1
Call rollDice()
Print result
Else
Display the closing message
flipCoin Function
generate an integer random number between 1 and 2.
If a 1 is generated return "heads", else return "tails"
rollDice Function
generate a random number between 1 and the number of sides
return the result of the roll.

Answers: 2
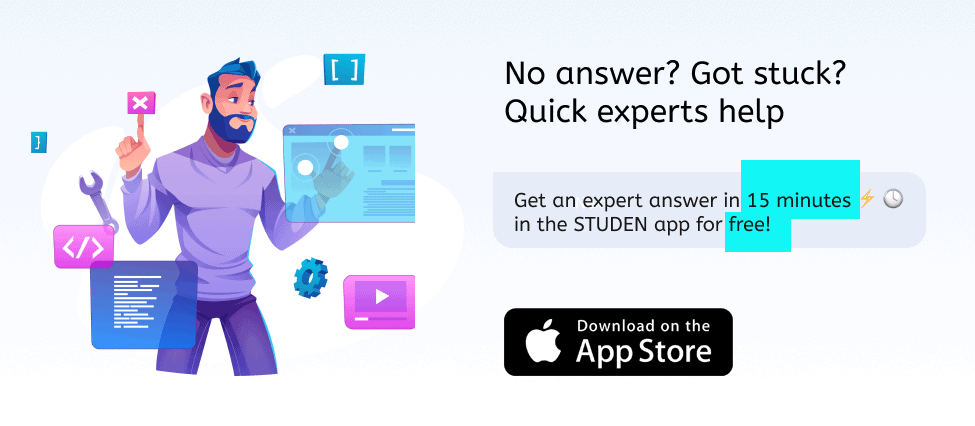
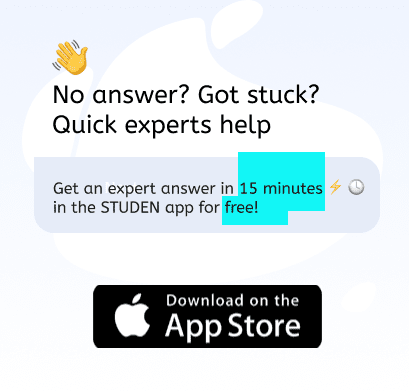
Another question on Computers and Technology

Computers and Technology, 21.06.2019 23:30
Show that there is a language a ⚆ {0, 1} â— with the following properties: 1. for all x â a, |x| ≤ 5. 2. no dfa with fewer than 9 states recognizes a. hint: you don’t have to define a explicitly; just show that it has to exist. count the number of languages satisfying (1) and the number of dfas satisfying (2), and argue that there aren’t enough dfas to recognize all those languages. to count the number of languages satisfying (1), think about writing down all the strings of length at most 5, and then to define such a language, you have to make a binary decision for each string about whether to include it in the language or not. how many ways are there to make these choices? to count the number of dfas satisfying (2), consider that a dfa behaves identically even if you rename all the states, so you can assume without loss of generality that any dfa with k states has the state set {q1, q2, . . , qk}. now think about how to count how many ways there are to choose the other four parts of the dfa.
Answers: 3

Computers and Technology, 22.06.2019 17:00
Aisha has finished working on a word processing document that contains 15 pages. she has added some special elements in the first three pages, page 9 and 10, and page 15 from the document. she wants to print only these pages to see how they look. which option is the correct way to represent (in the print dialog box) the pages that aisha wants to print
Answers: 3

Computers and Technology, 23.06.2019 07:50
Most shops require the technician to enter a starting and ending time on the repair order to track the actual time the vehicle was in the shop and closed out by the office. this time is referred to as _ time ? a. comeback b. ro c. cycle d. lead
Answers: 1

Computers and Technology, 23.06.2019 22:30
Apart from confidential information, what other information does nda to outline? ndas not only outline confidential information, but they also enable you to outline .
Answers: 1
You know the right answer?
Write a program that simulates flipping a coin and rolling a dice.
A user will input their choice o...
Questions

Mathematics, 24.06.2020 03:01
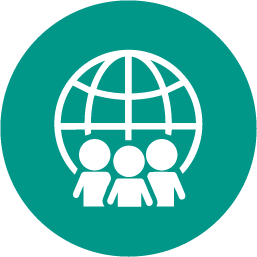


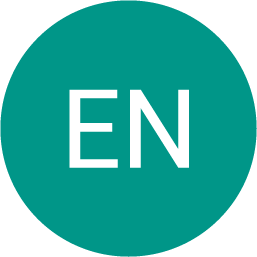


Mathematics, 24.06.2020 03:01


Mathematics, 24.06.2020 03:01





Mathematics, 24.06.2020 03:01



Mathematics, 24.06.2020 03:01
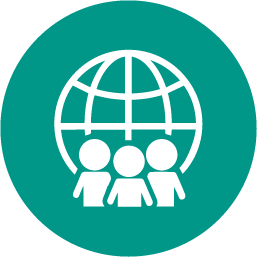
History, 24.06.2020 03:01


Mathematics, 24.06.2020 03:01