
Computers and Technology, 21.05.2021 03:40 osmarirodriguez1163
I would like assistance regarding this Java Edhesive Assignment.
Create a class RightTriangle which implements the API exactly as described in the following Javadoc.
Don't forget - you will need to use the Pythagorean theorem to find the hypotenuse (and therefore the perimeter) of a right triangle. You can find the area of a right triangle by multiplying the base and height together, then dividing this product by 2.
Use the runner_RightTriangle file to test the methods in your class; do not add a main method to your RightTriangle class.
Hint 1 - Javadoc only shows public methods, variables and constructors. You will need to add some private member variables to your RightTriangle class to store the necessary information. Think carefully about what information actually needs to be stored and how this will need to be updated when methods change the state of a RightTriangle object.
Hint 2 - As in the previous lesson's exercise it's helpful to add your method/constructor headers and any dummy returns needed before implementing each one properly. This will allow you to test your code using the runner class as you go along.
Runner Code:
import java. util. Scanner;
public class runner_RightTriangle{
public static void main(String[] args){
Scanner scan = new Scanner(System. in);
RightTriangle t = new RightTriangle();
String instruction = "";
while(!instruction. equals("q")){
System. out. println("Type the name of the method to test. Type c to construct a new triangle, q to quit.");
instruction = scan. nextLine();
if(instruction. equals("getArea")){
System. out. println(t. getArea());
}
else if(instruction. equals("getBase")){
System. out. println(t. getBase());
}
else if(instruction. equals("getHeight")){
System. out. println(t. getHeight());
}
else if(instruction. equals("getHypotenuse")){
System. out. println(t. getHypotenuse());
}
else if(instruction. equals("getPerimeter")){
System. out. println(t. getPerimeter());
}
else if(instruction. equals("toString")){
System. out. println(t);
}
else if(instruction. equals("setBase")){
System. out. println("Enter parameter value:");
double arg = scan. nextDouble();
t. setBase(arg);
scan. nextLine();
}
else if(instruction. equals("setHeight")){
System. out. println("Enter parameter value:");
double arg = scan. nextDouble();
t. setHeight(arg);
scan. nextLine();
}
else if(instruction. equals("equals")){
System. out. println("Enter base and height:");
double bs = scan. nextDouble();
double ht = scan. nextDouble();
RightTriangle tOther = new RightTriangle(bs, ht);
System. out. println(t. equals(tOther));
scan. nextLine();
}
else if(instruction. equals("c")){
System. out. println("Enter base and height:");
double bs = scan. nextDouble();
double ht = scan. nextDouble();
t = new RightTriangle(bs, ht);
scan. nextLine();
}
else if(!instruction. equals("q")){
System. out. println("Not recognized.");
}
}
}
}

Answers: 1
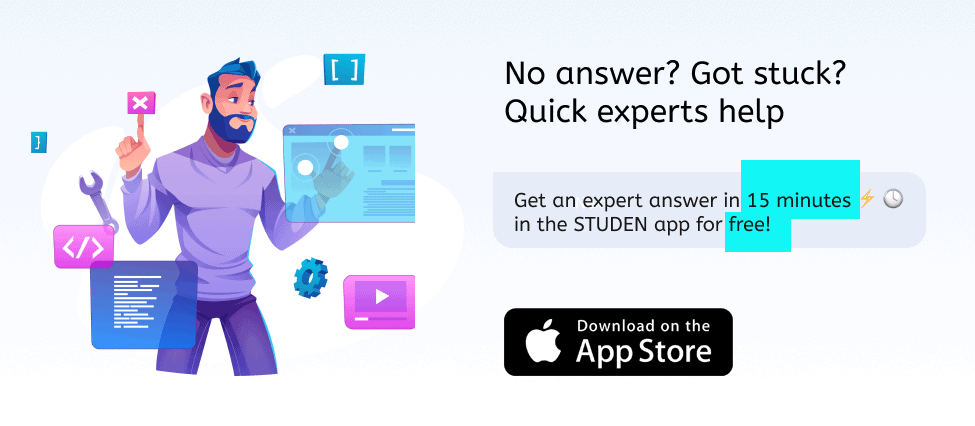
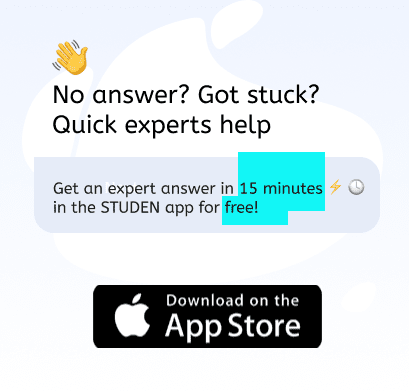
Another question on Computers and Technology

Computers and Technology, 22.06.2019 17:40
Write a modular program (no classes yet, just from what you learned last year), that allows two players to play a game of tic-tac-toe. use a two-dimensional char array with 3 rows and 3 columns as the game board. each element of the array should be initialized with an asterisk (*). the program should display the initial board configuration and then start a loop that does the following: allow player 1 to select a location on the board for an x by entering a row and column number. then redisplay the board with an x replacing the * in the chosen location. if there is no winner yet and the board is not yet full, allow player 2 to select a location on the board for an o by entering a row and column number. then redisplay the board with an o replacing the * in the chosen location. the loop should continue until a player has won or a tie has occurred, then display a message indicating who won, or reporting that a tie occurred. player 1 wins when there are three xs in a row, a column, or a diagonal on the game board. player 2 wins when there are three ox in a row, a column, or a diagonal on the game board. a tie occurs when all of the locations on the board are full, but there is no winner. input validation: only allow legal moves to be entered. the row must be 1, 2, or 3. the column must be 1, 2 3. the (row, column) position entered must currently be empty (i.e., still have an asterisk in it).
Answers: 1

Computers and Technology, 23.06.2019 04:00
In a word processing program, such as microsoft word, which feature to you choose the desired picture enhancement?
Answers: 2

Computers and Technology, 23.06.2019 18:30
List 3 items that were on kens resume that should have been excluded
Answers: 1

Computers and Technology, 24.06.2019 10:00
When writing a business letter, how many times can you use the same merge field in a document? once once, unless using the address block feature unlimited it will depend on the type of document you choose
Answers: 1
You know the right answer?
I would like assistance regarding this Java Edhesive Assignment.
Create a class RightTriangle which...
Questions
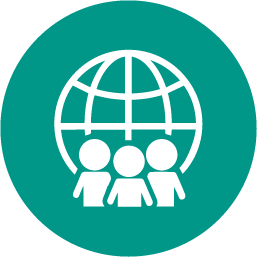

Mathematics, 05.05.2020 15:24



Chemistry, 05.05.2020 15:25
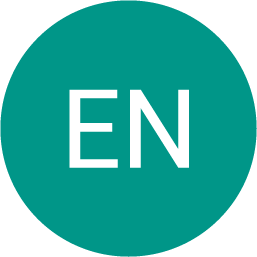

Mathematics, 05.05.2020 15:25

Business, 05.05.2020 15:25


Mathematics, 05.05.2020 15:25
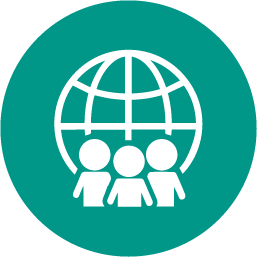
History, 05.05.2020 15:25
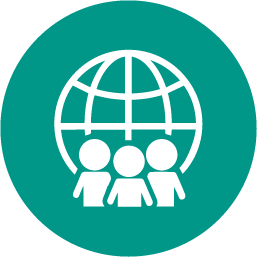


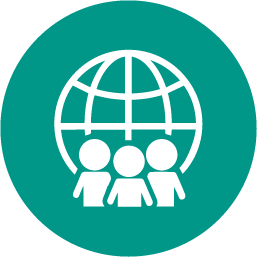

Mathematics, 05.05.2020 15:25


Mathematics, 05.05.2020 15:25
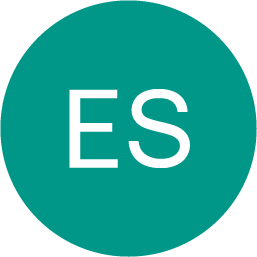

Mathematics, 05.05.2020 15:25