
Computers and Technology, 27.05.2021 06:20 demil4257
A simple software system for a library models a library as a collection of books and patrons, as per the requirements below:
- A patron can have at most three books out on loan at any given time.
- Each book has a title, an author, a patron to whom it has been checked out, and a list of patrons waiting for that book to be returned.
- When a patron wants to borrow a book, that patron is automatically added to the book’s wait list if the book is already checked out.
- When a patron returns a book, it is automatically loaned to the first patron on its wait list who can check out a book.
- Each patron has a name and the number of books that patron has currently checked out.
Develop the classes Book and Patron to model these objects. Think first of the interface or set of methods to be used with each class, and then choose appropriate data structures for the state of the objects.
Here is the code so far, please write the rest of the program based on the above guidelines:
class Patron(object):
"""This class represents a patron
with a name and a number of books checked out."""
MAX_BOOKS_OUT = 3
def __init__(self, name):
self._name = name
self._numBooksOut = 0
def __str__(self):
result = self._name + ', ' + str(self._numBooksOut) + \
" books out"
return result
def getNumBooksOut(self):
"""Returns the number of books out."""
def inc(self):
"""Increments the number of books out."""
def dec(self):
"""Decrements the number of books out."""
class Book(object):
"""This class represents a book with a title, author,
a patron to whom the book is check out, and a wait list
of patrons for it."""
def __init__(self, title, author):
"""Creates a new book with the given title and author."""
self._title = title
self._author = author
self._patron = None
self._waitList = []
def __str__(self):
result = 'Title: ' + self._title + '\n'
result += 'Author: ' + self._author + '\n'
if self._patron:
result += "Checked out to: " + str(self._patron) + '\n'
else:
result += "Not checked out\n"
result += "Wait list:\n"
for patron in self._waitList:
result += str(patron) + '\n'
return result
def borrowMe(self, patron):
"""Attempts to loan book to patron."""
def returnMe(self):
"""Current patron returns book, attempts to loan it
to a qualified waiting patron."""
def main():
"""Tests the Patron and Book classes."""
p1 = Patron("Ken")
p2 = Patron("Martin")
b1 = Book("Atonement", "McEwan")
b2 = Book("The March", "Doctorow")
b3 = Book("Beach Music", "Conroy")
b4 = Book("Thirteen Moons", "Frazier")
print(b1.borrowMe(p1))
print(b2.borrowMe(p1))
print(b3.borrowMe(p1))
print(b1.borrowMe(p2))
print(b4.borrowMe(p1))
print(p1)
print(b1)
print(b4)
print(b1.returnMe())
print(b2.returnMe())
print(b1)
print(b2)
main()

Answers: 3
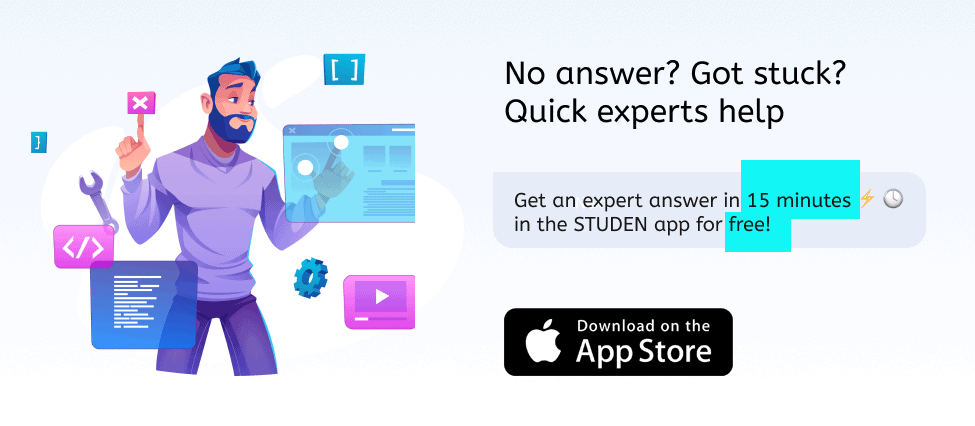
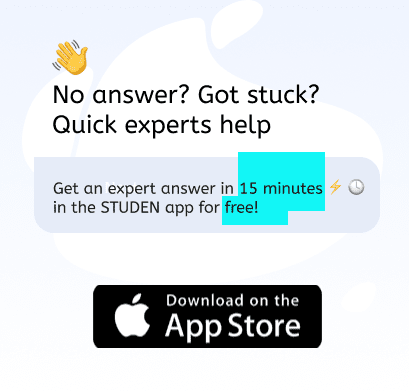
Another question on Computers and Technology

Computers and Technology, 22.06.2019 05:20
Write a program called assignment3 (saved in a file assignment3.java) that computes the greatest common divisor of two given integers. one of the oldest numerical algorithms was described by the greek mathematician, euclid, in 300 b.c. it is a simple but very e↵ective algorithm that computes the greatest common divisor of two given integers. for instance, given integers 24 and 18, the greatest common divisor is 6, because 6 is the largest integer that divides evenly into both 24 and 18. we will denote the greatest common divisor of x and y as gcd(x, y). the algorithm is based on the clever idea that the gcd(x, y) = gcd(x ! y, y) if x > = y and gcd(x, y) = gcd(x, y ! x) if x < y. the algorithm consists of a series of steps (loop iterations) where the “larger” integer is replaced by the di↵erence of the larger and smaller integer. this continues until the two values are equal. that is then the gcd.
Answers: 3

Computers and Technology, 22.06.2019 10:00
Jackson is teaching the decimal number system. he wants his students to know how to expand numbers by powers of 10. which is the correct order in which digits are assigned values in the decimal number system?
Answers: 1

Computers and Technology, 22.06.2019 22:40
Least square fit to polynomial write a function leastsquarefit3pol that solves a linear system of equations to find a least squares fit of a third order polynomial to an experimental data set given as two row arrays. the function leastsquarefit3pol must explicitly solve a set of linear equations and cannot use polyfit. there should be no restriction on the size of the problem that can be solved.
Answers: 1

Computers and Technology, 23.06.2019 23:40
Which of the following calculates the total from the adjacent cell through the first nonnumeric cell by default, using the sum function in its formula? -average -autosum -counta -max
Answers: 1
You know the right answer?
A simple software system for a library models a library as a collection of books and patrons, as per...
Questions

Mathematics, 16.04.2021 03:40


Mathematics, 16.04.2021 03:40

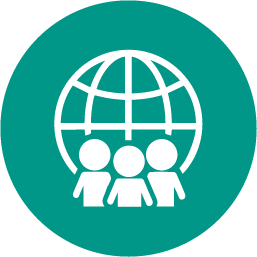



Geography, 16.04.2021 03:40
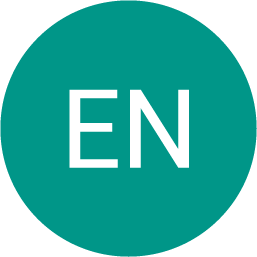
English, 16.04.2021 03:40
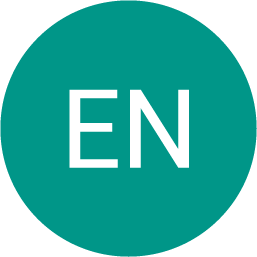


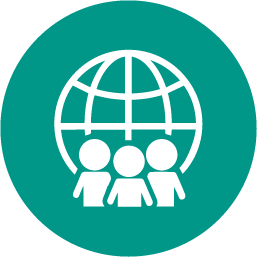

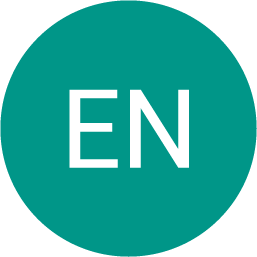


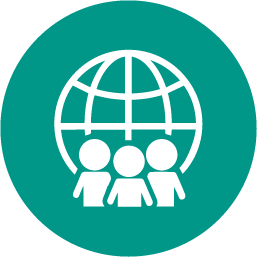
History, 16.04.2021 03:40
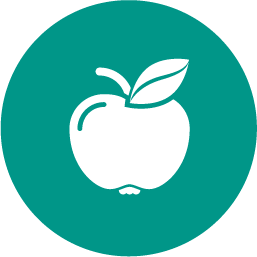
Physics, 16.04.2021 03:40