
Computers and Technology, 02.06.2021 22:20 genesiloves
For the first part of this lab, copy your working ArrayStringList code into the GenericArrayList class.(already in the code) Then, modify the class so that it can store any type someone asks for, instead of only Strings. You shouldn't have to change any of the actual logic in your class to accomplish this, only type declarations (i. e. the types of parameters, return types, etc.)
Note:
In doing so, you may end up needing to write something like this (where T is a generic type):
T[] newData = new T[capacity];
...and you will find this causes a compiler error. This is because Java dislikes creating new objects of a generic type. In order to get around this error, you can write the line like this instead:
T[] new Data = (T[]) new Object[capacity]
This creates an array of regular Objects which are then cast to the generic type. It works and it doesn't anger the Java compiler. How amazing!
Once you're done, screenshot or save your code for checkin later.
For the second part of the lab, modify your GenericArrayList so that it can store any type that is comparable to a Point. Remember the Point andPoint3D classes? Both of those implement the Comparable interface, so they both can compared to a Point. In fact, they are the only classes that can be compared to a Point, so after modifying your GenericArrayList, it should only be able to contain these two classes.
n both parts, test your classes by following the directions in the comments. They will ask you to uncomment some code and look for a specific result.
public class GenericArrayList {
/* YOUR CODE HERE
* Copy your code from your ArrayStringList class, and place it within
* this class.
*
* Only copy the code you filled out! Don't copy the main method.
*/
// Place code here
public class ArrayStringList {
private String[] data;
private int size;
private void resizeData(int newSize) {
String[] str = new String[newSize];
for(int i = 0; i < size; i++) {
str[i] = data[i];
}
data=str;
}
public ArrayStringList(int initialCapacity) {
data = new String[initialCapacity];
size = 0;
}
public void add(String str) {
if(size < data. length) {
data[size] = str;
size++;
} else {
resizeData(2 * data. length);
data[size] = str;
size++;
}
}
ublic void add(int index, String str) {
if(index < data. length && index >= 0) {
data[index] = str;
size++;
}
}
public String get(int index) {
if(index < data. length && index >= 0) {
return data[index];
}
return null;
}
public void remove(int index) {
if(index < data. length && index >= 0) {
for(int i = index; i < data. length; i++) {
if((i + 1) < size) {
data[i] = data[i + 1];
}
}
size--;
}
}
public int size() {
return size;
}
public boolean contains(String str) {
for(int i = 0; i < data. length; i++) {
if(str. equals(data[i])) {
return true;
}
}
return false;
}
public static void main(String[] args) {
/* PART 1:
* Modify the GenericArrayList above so that it can store *any* class,
* not just strings.
* When you've done that, uncomment the block of code below, and see if
* it compiles. If it does, run it. If there are no errors, you did
* it right!
*/
GenericArrayList pointList = new GenericArrayList(2);
pointList. add(new Point(0, 0));
pointList. add(new Point(2, 2));
pointList. add(new Point(7, 0));
pointList. add(new Point(19.16f, 22.32f));
pointList. remove(0);
Point p = pointList. get(2);
if (p. x != 19.16f && p. y != 22.32f) {
throw new AssertionError("Your GenericArrayList compiled properly "
+ "but is not correctly storing things. Make sure you didn't "
+ "accidentally change any of your ArrayStringList code, aside "
+ "from changing types.");
}
GenericArrayList floatList = new GenericArrayList(2);
for (float f = 0.0f; f < 100.0f; f += 4.3f) {
floatList. add(f);
}
float f = floatList. get(19);
System. out. println("Hurray, everything worked!");
/* PART 2:
* Now, modify your GenericArrayList again so that it can only store
* things that are comparable to a Point.
*
* If you don't know how to do this, reference zybooks and your textbook
* for help.
*
* When you are ready to test it, uncomment the code above and run the
* code below.
*/
/*
GenericArrayList pointList = new GenericArrayList(2);
GenericArrayList pointList3D = new GenericArrayList(3);
pointList. add(new Point(0, 0));
pointList. add(new Point(2, 2));
pointList. add(new Point(7, 0));
pointList. add(new Point(19.16f, 22.32f));
pointList3D. add(new Point3D(1.0f, 2.0f, 3.0f));
pointList3D. add(new Point3D(7.3f, 4, 0));
Point p = pointList. get(2);
Point3D p3 = pointList3D. get(0);
// You should get a compilation error on this line!
GenericArrayList floatList = new GenericArrayList(2);
*/
}
}
}

Answers: 1
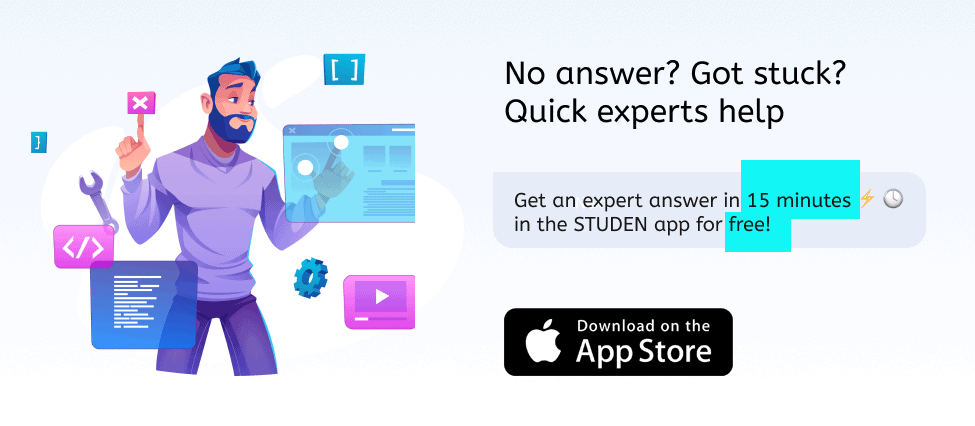
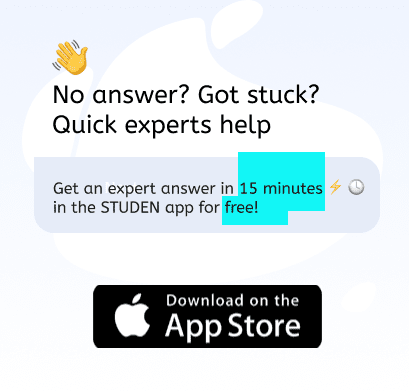
Another question on Computers and Technology

Computers and Technology, 21.06.2019 13:30
Question 1(multiple choice worth 5 points) (06.04 mc) how are the respiratory and circulatory systems similar? both systems provide glucose and other substances to cells. both systems regulate blood flow throughout the body. both systems remove carbon dioxide from the body. both systems send messages to the nervous system for breathing. question 2(multiple choice worth 5 points) (06.04 lc) the respiratory system controls and the circulatory systems controls antibodies; digestion breathing; blood flow muscles; bones nerves; senses question 3(multiple choice worth 5 points) (06.04 hc) read the following summary, which describes a body process. then, answer the question. blood enters the right side of the heart and travels to the lungs. there, the lungs eliminate carbon dioxide and exchange it for more oxygen. the oxygen-rich blood enters the left side of the heart. then, arteries carry blood away from the heart. what process is being described? circulation excretion immune response respiration question 4(multiple choice worth 5 points) (06.04 lc) the system is responsible for moving blood cells throughout the body. circulatory endocrine immune respiratory question 5(multiple choice worth 5 points) (06.04 mc) which of the following functions as part of the excretory and respiratory systems? blood heart intestines lungs
Answers: 1

Computers and Technology, 22.06.2019 07:00
You will be given two character arrays of the same size, one will contain a number of ships. ships will move around the character array based on which way they are facing and the route they are on. routes are given in the other array. the route consists of '-' and '|' for straight paths, '\' and '/' for curves, and '+' for intersections. there are ships on these routes. ships always face a direction, '^' for up, '> ' for right, 'v' for down, and '< ' for left. any time the ships hit a '\' or a '/' it will turn as you would expect a ship to turn (e.g. a '^' that moves into a '/' will turn right). at an intersection, ships will always continue straight through. all ships move at the same speed, ships take turns moving and all ships move during one 'tick'. the one in the most top left goes first, followed by those to its right, then the ones in the next row. it iterates along the rows and then down the columns. each ship moves one space on its turn moving along the route. your function needs to return the position of the first collision between two ships and the number of ticks before the crash occurred.
Answers: 2


Computers and Technology, 23.06.2019 12:50
Which syntax error in programming is unlikely to be highlighted by a compiler or an interpreter? a variable name misspelling a missing space a comma in place of a period a missing closing quotation mark
Answers: 1
You know the right answer?
For the first part of this lab, copy your working ArrayStringList code into the GenericArrayList cla...
Questions

Mathematics, 18.07.2019 02:30

Mathematics, 18.07.2019 02:30


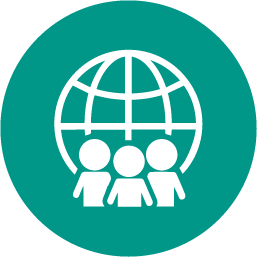



Mathematics, 18.07.2019 02:30


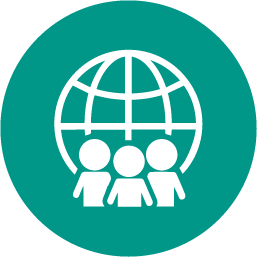
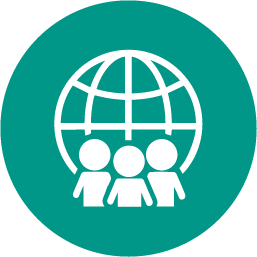

Biology, 18.07.2019 02:30
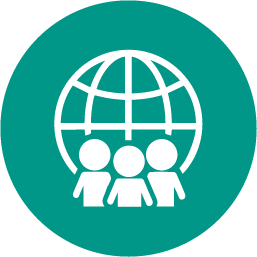
Social Studies, 18.07.2019 02:30
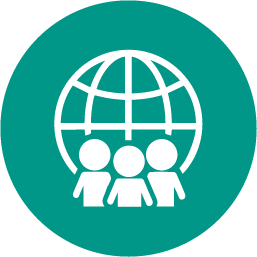
Social Studies, 18.07.2019 02:30


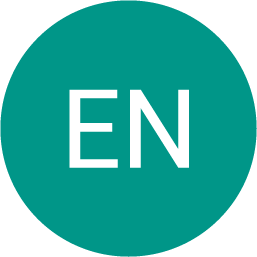
English, 18.07.2019 02:30

Mathematics, 18.07.2019 02:30